PostgreSQL Hibernate 6 JSON Example
This example demonstrates storing and retrieving JSON data using PostgreSQL and Hibernate 6. We'll use a simple Product
entity with a JSONB column to store product details. Assume you have a PostgreSQL database set up and a Hibernate project configured.
First, let's define the Product
entity:
import javax.persistence.*; @Entity @Table(name = "products") public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(columnDefinition = "jsonb") private String details; // Using String to represent JSONB // Getters and setters public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getDetails() { return details; } public void setDetails(String details) { this.details = details; } }
Next, you'll need a Hibernate configuration file (hibernate.cfg.xml
or equivalent) specifying your database connection details and mapping the Product
entity. Crucially, you don't need any special Hibernate annotations for JSONB; Hibernate handles it automatically thanks to the columnDefinition
attribute.
Finally, here's some sample code to save and retrieve a Product
:
// ... Hibernate Session setup ... Session session = sessionFactory.openSession(); Transaction transaction = session.beginTransaction(); Product product = new Product(); product.setDetails("{\"name\": \"Example Product\", \"price\": 19.99, \"description\": \"This is a test product\"}"); session.persist(product); transaction.commit(); session.close(); // Retrieve the product session = sessionFactory.openSession(); Product retrievedProduct = session.get(Product.class, product.getId()); System.out.println(retrievedProduct.getDetails()); session.close();
This code snippet demonstrates the basic usage. Remember to replace placeholders with your actual database credentials and adjust the path to your hibernate.cfg.xml
file. The key is using columnDefinition = "jsonb"
in the entity mapping to tell Hibernate that this column should be treated as JSONB.
How can I efficiently store and retrieve JSON data using PostgreSQL and Hibernate 6?
Efficiently storing and retrieving JSON data with PostgreSQL and Hibernate 6 hinges on several factors:
-
Use JSONB: PostgreSQL's JSONB data type is crucial for performance. It's optimized for indexing and querying, unlike the JSON type. Always use
columnDefinition = "jsonb"
in your Hibernate entity mappings. -
Indexing: Create appropriate indexes on JSONB columns. Instead of indexing the entire JSONB column, create indexes on specific JSONB paths using the
GIN
index type. For example, if you frequently query by thename
field within your JSONB data, you might use an index like this in your SQL:CREATE INDEX idx_product_name ON products USING gin((details->>'name'));
This allows PostgreSQL to efficiently search for specific values within that JSONB field. - Partial Indexes: For even better performance, consider partial indexes. These indexes only cover a subset of the data, improving query performance when only a specific part of the JSONB data is needed.
-
Optimized Queries: Avoid using
jsonb_each
,jsonb_each_text
, and similar functions in your Hibernate queries unless absolutely necessary. These functions can lead to performance degradation as they expand the JSON data into rows. Instead, leverage PostgreSQL's JSONB operators (->
,->>
,@>
,, etc.) directly within your JPQL or Criteria API queries.
- Batch Processing: For large-scale operations, use batch processing techniques to insert or update JSON data efficiently. This minimizes the number of database round trips.
- Data Normalization: While JSONB offers flexibility, consider if some data should be normalized into separate tables for better query performance. Overly large or complex JSONB data can negatively impact performance.
What are the best practices for mapping JSON columns to Java objects with Hibernate 6 and PostgreSQL?
Best practices for mapping JSON columns to Java objects in Hibernate 6 and PostgreSQL revolve around choosing the right approach for your data structure and query patterns:
-
Native JSONB Mapping: As demonstrated in the example, the simplest and often most efficient approach is directly mapping the JSONB column to a
String
orJsonNode
(from the Jackson library) in your Java entity. This avoids unnecessary object mapping overhead. This is ideal when you need flexibility and perform frequent partial queries. - Custom Type: For more complex JSON structures, you might create a custom Hibernate UserType. This allows for mapping specific parts of the JSONB data to Java objects, providing type safety and potentially improving query performance for specific scenarios.
- Embedded Objects: If the JSON data represents a well-defined structure that's consistently used, consider using embedded objects or collections of embedded objects within your Java entity. This improves type safety and can simplify queries, but might lead to data redundancy if not managed carefully.
- Avoid Over-Mapping: Don't map every single field in the JSONB to a Java field if you don't need to access them directly in your Java code. Over-mapping can lead to unnecessary complexity and performance overhead.
Are there any performance considerations when working with JSON data in PostgreSQL using Hibernate 6?
Yes, several performance considerations are crucial when working with JSON data in PostgreSQL using Hibernate 6:
- Data Size: Large JSONB documents can significantly impact query performance. Keep JSONB data relatively concise and avoid storing unnecessary information.
- Query Selectivity: Poorly designed queries can lead to full table scans, significantly impacting performance. Use indexes effectively and leverage PostgreSQL's JSONB operators to target specific parts of the JSONB data.
- Index Strategy: Choose the right index type (GIN or BRIN) and carefully consider indexed paths within your JSONB data to optimize query performance. Avoid over-indexing, as this can also slow down write operations.
- Database Configuration: Ensure your PostgreSQL database server is appropriately configured with sufficient resources (CPU, memory, disk I/O) to handle the expected load.
- Connection Pooling: Utilize a connection pool to minimize the overhead of establishing database connections for each query.
- Caching: Implement appropriate caching strategies (e.g., Hibernate second-level cache) to reduce database access for frequently accessed data.
- Monitoring and Profiling: Monitor your application's performance using database monitoring tools and profiling techniques to identify bottlenecks and optimize your queries and data structures. Understanding query execution plans is crucial for performance tuning.
The above is the detailed content of PostgreSQL Hibernate 6 JSON Example. For more information, please follow other related articles on the PHP Chinese website!
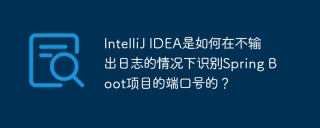
Start Spring using IntelliJIDEAUltimate version...
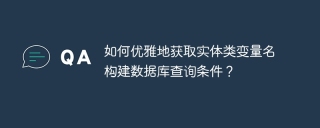
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
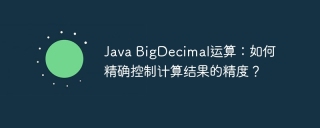
Java...
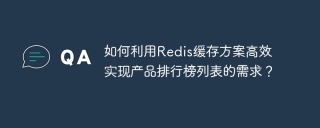
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
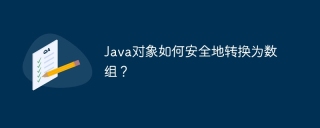
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
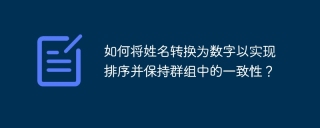
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
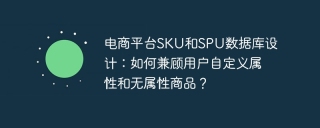
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
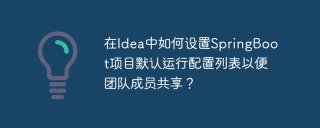
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
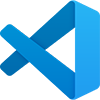
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software