Division operation in Bash script: efficiently handle integers and floating point numbers
Bash scripts are a powerful tool for automating tasks on Linux and Unix-like systems. Although it is known for file and process operations, arithmetic operations such as division also play a crucial role in many scripts. Understanding how to correctly divide by two variables can help with resource allocation, data processing, and more. This article explores the subtleties of performing division in Bash, giving you the knowledge to perform arithmetic operations smoothly and efficiently.
Basic concept
Variables in Bash In Bash, variables are the names assigned to a piece of data that can be changed during script execution. Variables are often used to store numbers, strings, or file names, and these data can be manipulated to perform various operations.
Overview of Arithmetic Operations Bash supports basic arithmetic operations directly or through external utilities. These operations include addition, subtraction, multiplication, and division. However, Bash itself performs integer arithmetic, which means it can only handle integers without decimals, unless using other tools.
Introduction to Arithmetic Commands There are two main methods to perform arithmetic operations in Bash:
- expr: An external utility that evaluates expressions, including arithmetic calculations.
- Arithmetic extension $(( )): A function of Bash that allows for direct arithmetic operations in scripts.
Setting script
Create a Bash script file To start writing scripts, create a file with a .sh extension using a text editor such as Nano or Vim. For example:
nano myscript.sh
Make the script executable After writing a script, you need to use the chmod command to make it executable:
chmod x myscript.sh
Basic Syntax Bash scripts usually start with shebang (#!) followed by the path to the Bash interpreter:
#!/bin/bash # 你的脚本从这里开始
Declare variable
Assignment To declare and assign values to variables in Bash, use the following syntax:
var1=10 var2=5
These variables can now be used in arithmetic operations.
Perform division
Use the expr expr command to use:
result=$(expr $var1 / $var2)
echo "结果是 $result"
This outputs the result of var1 divided by var2.
Training integer division
Since expr only supports integer arithmetic, dividing two integers that cannot be divided will cause the fractional part to be truncated.
Use arithmetic extension $(( )) Arithmetic extension allows for simpler syntax and direct script integration:
result=$(($var1 / $var2))
echo "结果是 $result"
This method is simpler and does not produce external processes, so it is faster than expr.
Processing non-integer results
Challenges of floating point division Bash itself does not support floating point arithmetic, which complicates division that leads to non-integral values.
Solutions for floating point division To handle floating point division, you can use the bc tool, which is an arbitrary precision calculator language:
result=$(echo "$var1 / $var2" | bc -l)
echo "结果是 $result"
This command sends the division operation to bc, which processes floating point arithmetic and returns the result.
Common traps and errors
Divided by zero Trying to divide by zero will cause a script error. Before performing division, be sure to check if the denominator is zero:
if [ $var2 -eq 0 ]; then
echo "错误:被零除。"
else
result=$(($var1 / $var2))
echo "结果是 $result"
fi
Processing non-digital inputs Make sure the input is a digital to avoid runtime errors:
if ! [[ "$var1" =~ ^[0-9] $ ]] || ! [[ "$var2" =~ ^[0-9] $ ]]; then
echo "错误:非数字输入。"
else
result=$(($var1 / $var2))
echo "结果是 $result"
fi
Practical example
Interactive scripts are used for user input Create a script that accepts user input and performs division:
#!/bin/bash
echo "输入两个数字:"
read var1 var2
if [[ "$var2" -eq 0 ]]; then
echo "不能被零除。"
else
result=$(echo "$var1 / $var2" | bc -l)
echo "除法结果:$result"
fi
Conclusion
This article introduces the key points of performing division in Bash scripts, from integer operations to processing floating point numbers. By understanding these principles, you can enhance the functionality of your scripts and perform complex calculations easily. Try using these techniques to improve your scripting skills and solve more advanced problems.
The above is the detailed content of How to Divide Two Variables in Bash Scripting. For more information, please follow other related articles on the PHP Chinese website!
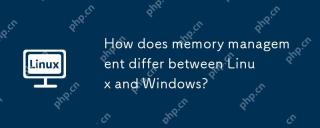
LinuxandWindowsmanagememorydifferentlyduetotheirdesignphilosophies.Linuxusesovercommittingforbetterperformancebutrisksout-of-memoryerrors,whileWindowsemploysdemand-pagingandmemorycompressionforstabilityandefficiency.Thesedifferencesimpactdevelopmenta
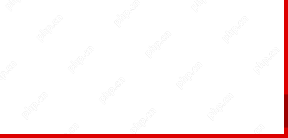
Linux systems rely on firewalls to safeguard against unauthorized network access. These software barriers control network traffic, permitting or blocking data packets based on predefined rules. Operating primarily at the network layer, they manage
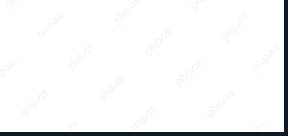
Determining if your Linux system is a desktop or laptop is crucial for system optimization. This guide outlines simple commands to identify your system type. The hostnamectl Command: This command provides a concise way to check your system's chassis
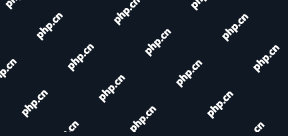
Guide to adjust the number of TCP/IP connections for Linux servers Linux systems are often used in servers and network applications. Administrators often encounter the problem that the number of TCP/IP connections reaches the upper limit, resulting in user connection errors. This article will guide you how to improve the maximum number of TCP/IP connections in Linux systems. Understanding TCP/IP connection number TCP/IP (Transmission Control Protocol/Internet Protocol) is the basic communication protocol of the Internet. Each TCP connection requires system resources. When there are too many active connections, the system may reject new connections or slow down. By increasing the maximum number of connections allowed, server performance can be improved and more concurrent users can be handled. Check the current number of Linux connections limits Change settings
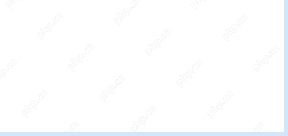
SVG (Scalable Vector Graphics) files are ideal for logos and illustrations due to their resizability without quality loss. However, PNG (Portable Network Graphics) format often offers better compatibility with websites and applications. This guide d
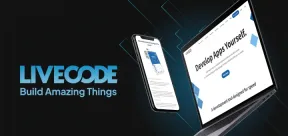
LiveCode: A Cross-Platform Development Revolution LiveCode, a programming language debuting in 1993, simplifies app development for everyone. Its high-level, English-like syntax and dynamic typing enable the creation of robust applications with ease
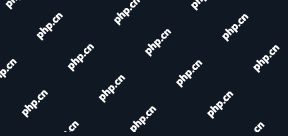
This guide provides a step-by-step process for resetting a malfunctioning USB device via the Linux command line. Troubleshooting unresponsive or disconnected USB drives is simplified using these commands. Step 1: Identifying Your USB Device First, i
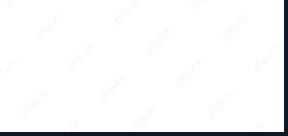
Temporarily setting a static IP address on Linux is invaluable for network troubleshooting or specific session configurations. This guide details how to achieve this using command-line tools, noting that the changes are not persistent across reboots


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
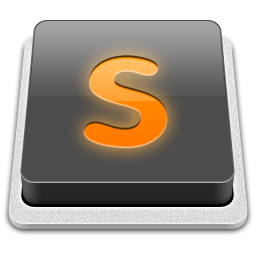
SublimeText3 Mac version
God-level code editing software (SublimeText3)
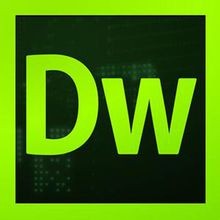
Dreamweaver CS6
Visual web development tools
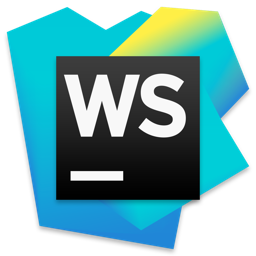
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
