Laravel 6 Tutorial: A Comprehensive Guide
This section provides a foundational understanding of Laravel 6, focusing on its core concepts and features. Laravel is a robust PHP framework known for its elegant syntax and developer-friendly features. A Laravel 6 tutorial would typically cover several key areas:
-
Installation and Setup: This involves installing Composer (PHP's dependency manager), cloning the Laravel framework using Composer (
composer create-project --prefer-dist laravel/laravel your-project-name
), and configuring the database connection in the.env
file. Understanding the directory structure (app, config, database, public, resources, routes, storage, etc.) is crucial. -
Routing: Laravel uses a fluent, expressive routing system defined in
routes/web.php
(for web requests) androutes/api.php
(for API requests). Tutorials would demonstrate defining routes using various methods (GET, POST, PUT, DELETE), route parameters, route groups for organizing routes, and using route middleware for authentication and other functionalities. - Controllers: Controllers handle user requests and interact with models to manipulate data. A tutorial would cover creating controllers, defining methods to process requests, and returning views or JSON responses.
- Models and Eloquent ORM: Laravel's Eloquent ORM provides an elegant way to interact with databases. Tutorials would cover defining models, performing CRUD (Create, Read, Update, Delete) operations, using relationships (one-to-one, one-to-many, many-to-many), and querying data using Eloquent's query builder.
-
Views and Blade Templating Engine: Laravel uses Blade, a templating engine, to create reusable views. A tutorial would cover creating views, using Blade directives (e.g.,
@if
,@foreach
,@include
), passing data to views from controllers, and using layouts for consistent design. - Migrations: Migrations are used to manage database schema changes. A tutorial would cover creating migrations, defining table structures, and running migrations using Artisan commands.
- Artisan CLI: The Artisan command-line interface provides a powerful way to interact with the Laravel framework. Tutorials would demonstrate using common Artisan commands for tasks like generating code (controllers, models, migrations), running database seeds, and clearing cached data.
Where can I find comprehensive documentation for Laravel 6's features and functionalities?
The official Laravel documentation is the best resource for comprehensive information on Laravel 6's features and functionalities. You can find it at [https://laravel.com/docs/6.x](https://laravel.com/docs/6.x). This documentation is well-structured, regularly updated, and covers all aspects of the framework, from basic concepts to advanced topics. In addition to the official documentation, you can find numerous tutorials, blog posts, and videos online covering specific aspects of Laravel 6. Searching for specific features or functionalities on sites like YouTube, Laracasts, and other Laravel community forums will yield helpful resources. Remember to always check the date of the resource to ensure its relevance to Laravel 6, as newer versions may introduce changes.
What are some common pitfalls to avoid when building applications with Laravel 6?
Several common pitfalls can hinder development when using Laravel 6. Avoiding these can lead to more efficient and maintainable applications:
- Ignoring Eloquent Relationships: Failing to utilize Eloquent relationships can lead to inefficient and repetitive database queries. Properly defining relationships significantly improves performance and code readability.
- Over-Reliance on Global Helpers: While convenient, excessive use of global helper functions can reduce code clarity and testability. Favor well-defined methods within classes whenever possible.
- Neglecting Proper Error Handling: Insufficient error handling can lead to unexpected application behavior and security vulnerabilities. Implement robust error handling mechanisms, including logging and user-friendly error messages.
- Ignoring Security Best Practices: Failing to sanitize user inputs and implement proper authentication and authorization mechanisms can expose your application to security risks. Always validate and sanitize user input, and leverage Laravel's built-in security features.
- Poorly Structured Code: Lack of consistent code style and structure can make the application difficult to maintain and extend. Adhere to coding standards and use design patterns to improve code organization.
- Ignoring Caching: Failing to implement caching can lead to slow performance, especially with database-intensive applications. Laravel provides various caching mechanisms that can significantly improve response times.
- Insufficient Testing: Lack of thorough testing can introduce bugs and regressions. Write unit tests, integration tests, and functional tests to ensure the quality and reliability of your application.
How can I effectively integrate Laravel 6 with popular third-party services and APIs?
Laravel 6 provides several ways to integrate with popular third-party services and APIs:
- Using Packages: Many packages are available through Composer to simplify integration with various services. Search for packages specific to the services you need (e.g., packages for Stripe, Twilio, Mailchimp). These packages often provide convenient wrappers and helpers for interacting with the APIs.
- Using Guzzle HTTP Client: Laravel's built-in Guzzle HTTP client provides a flexible way to make HTTP requests to external APIs. You can use Guzzle to send requests, handle responses, and manage authentication.
- Creating Custom Services: For more complex integrations, you can create custom services or repositories to encapsulate the logic for interacting with the third-party APIs. This promotes code reusability and maintainability.
- Using API Gateways: Consider using API gateways like Kong or Tyk to manage and secure your communication with external APIs. These tools can handle authentication, rate limiting, and other essential tasks.
- Following API Documentation: Always consult the official documentation of the third-party services you're integrating with. Understanding the API's endpoints, request formats, and authentication mechanisms is crucial for successful integration. Pay close attention to rate limits and other usage restrictions.
Remember to properly handle authentication and authorization when interacting with external APIs, ensuring the security of your application and user data. Always consider the potential impact of API calls on the performance of your application and implement appropriate error handling and retry mechanisms.
The above is the detailed content of Laravel6 usage tutorial. For more information, please follow other related articles on the PHP Chinese website!

Developers can efficiently track new versions of Laravel and ensure the use of the latest and safest code bases: 1. Use code snippets to check the latest version and compare it with the current version, 2. Use Composer and Laravel for dependency management, 3. Implement automated testing to deal with version conflicts, 4. Get feedback on new versions through community interaction, 5. Pay attention to Laravel's public roadmap and GitHub dynamics to plan updates.

Laravel's latest version (9.x) brings important security updates, including: 1) patching known vulnerabilities such as CSRF attacks; 2) enhancing overall security, such as CSRF protection and SQL injection defense. By understanding and applying these updates correctly, you can ensure that your Laravel app is always in the safest state.

LaravelMigrationsareversioncontrolfordatabases,allowingschemamanagementandevolution.1)Theyhelpmaintainteamsyncandconsistencyacrossenvironments.2)Usethemtocreatetableslikethe'users'tablewithnecessaryfields.3)Modifyexistingtablesbyaddingfieldslike'phon

SoftdeleteinLaravelisimplementedbyaddingtheSoftDeletestraittoamodel,markingrecordsasdeletedwithoutremovingthemfromthedatabase.1)AddSoftDeletestraittothemodelanddefine'deleted_at'asadate.2)Use'delete()'tosetthe'deleted_at'timestampinsteadofdeletingthe

React,Vue,andAngularcanbeintegratedwithLaravelbyfollowingspecificsetupsteps.1)ForReact:InstallReactusingLaravelUI,setupcomponentsinapp.js.2)ForVue:UseLaravel'sbuilt-inVuesupport,configureinapp.js.3)ForAngular:SetupAngularseparately,servethroughLarave

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
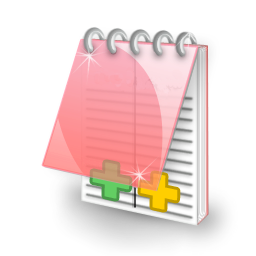
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
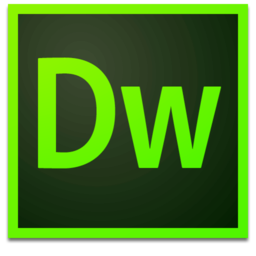
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
