Handling Download Link Failures in UniApp
This article addresses common issues related to downloading files within a UniApp application and provides solutions for handling failed download links.
UniApp Download File: How to Handle Download Link Failure?
When a download link fails in a UniApp application, several scenarios can occur: the link might be broken, the server might be down, or there might be network connectivity issues. Robust error handling is crucial for a smooth user experience. The most common approach involves using the uni.downloadFile
API, which provides a fail
callback function. This callback function is triggered when the download fails. Within this function, you should implement error handling logic to inform the user about the failure and potentially offer alternative solutions.
Here's an example using uni.downloadFile
:
uni.downloadFile({ url: 'your_download_link', success: function (res) { // Download successful console.log('Download successful:', res.tempFilePath); //Further actions like opening the file or saving it }, fail: function (err) { // Download failed console.error('Download failed:', err); uni.showModal({ title: 'Download Error', content: 'Failed to download the file. Please check your internet connection and try again later.', showCancel: false }); } });
This code snippet shows a basic implementation. You should extend it to handle specific error codes (if available) and provide more informative error messages to the user. For instance, you might differentiate between network errors and server errors to provide more tailored feedback.
How Can I Prevent Download Link Failures in My UniApp Project?
Preventing download link failures entirely is challenging, but you can significantly reduce their occurrence through proactive measures:
- Thorough Link Testing: Before deploying your application, rigorously test all download links in various network conditions (e.g., slow connection, no connection). Use automated testing tools where feasible.
- Link Validation: Implement server-side validation to ensure that the download links are valid and point to existing files. This could involve checking file existence before providing the link to the client.
- Error Handling on the Server: Implement robust error handling on your server-side to gracefully handle situations where the file is unavailable or inaccessible. Return appropriate error codes and messages to the client.
- Regular Link Updates: Regularly check and update your download links to ensure they remain active and functional.
- Using a CDN: Consider using a Content Delivery Network (CDN) to distribute your files across multiple servers. This improves reliability and reduces the chance of download failures due to server overload or downtime.
- Retry Mechanism: Implement a retry mechanism in your UniApp code. If the download fails, the app could automatically retry after a short delay, perhaps with exponential backoff to avoid overwhelming the server.
What Are the Best Practices for Handling Broken Download Links in a UniApp Application?
Best practices for handling broken download links focus on providing a positive user experience even when things go wrong:
- User-Friendly Error Messages: Avoid technical jargon. Use clear, concise language that the average user can understand. For example, instead of "HTTP 404 Not Found," say "The file you requested is currently unavailable."
- Informative Feedback: Provide specific information about the error, if possible, without exposing sensitive details.
- Alternative Solutions: Offer alternative ways to access the content, such as providing a different download link or suggesting contacting support.
- Logging and Reporting: Log all download failures to help you identify recurring problems and improve your application's reliability. Use a centralized logging system if possible.
- Graceful Degradation: If a download fails, ensure that the rest of your application continues to function without crashing.
What Alternative Solutions Exist if a Download Link Fails in a UniApp App?
If a download link fails, several alternative solutions can be considered:
- Providing Alternative Download Methods: Offer users the option to download the file from a different source, such as a cloud storage service (e.g., Dropbox, Google Drive) or an alternative server.
- Caching Downloaded Files: If the file is frequently accessed, consider caching it locally on the device. This avoids repeated downloads and improves performance. UniApp offers ways to access the device's file system.
- Progressive Download: For large files, consider using a progressive download technique, allowing users to start using the file before the entire download is complete.
- Displaying an Error Page: If the download is crucial to the application's functionality, display a dedicated error page explaining the situation and providing contact information for support.
- Using a different API: Explore alternative APIs or libraries for downloading files that might offer better error handling or resilience.
By implementing these strategies, you can create a more robust and user-friendly UniApp application that gracefully handles download link failures.
The above is the detailed content of How to handle UniApp download file failure. For more information, please follow other related articles on the PHP Chinese website!
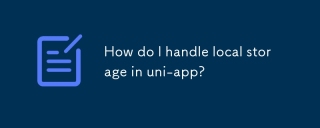
This article details uni-app's local storage APIs (uni.setStorageSync(), uni.getStorageSync(), and their async counterparts), emphasizing best practices like using descriptive keys, limiting data size, and handling JSON parsing. It stresses that lo

This article details uni-app's geolocation APIs, focusing on uni.getLocation(). It addresses common pitfalls like incorrect coordinate systems (gcj02 vs. wgs84) and permission issues. Improving location accuracy via averaging readings and handling
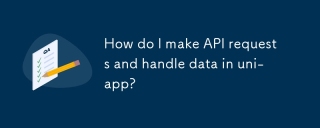
This article details making and securing API requests within uni-app using uni.request or Axios. It covers handling JSON responses, best security practices (HTTPS, authentication, input validation), troubleshooting failures (network issues, CORS, s
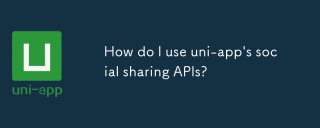
The article details how to integrate social sharing into uni-app projects using uni.share API, covering setup, configuration, and testing across platforms like WeChat and Weibo.
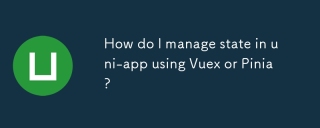
This article compares Vuex and Pinia for state management in uni-app. It details their features, implementation, and best practices, highlighting Pinia's simplicity versus Vuex's structure. The choice depends on project complexity, with Pinia suita
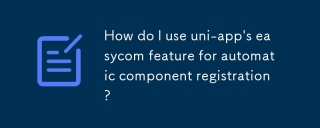
This article explains uni-app's easycom feature, automating component registration. It details configuration, including autoscan and custom component mapping, highlighting benefits like reduced boilerplate, improved speed, and enhanced readability.
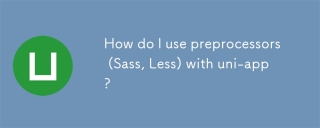
Article discusses using Sass and Less preprocessors in uni-app, detailing setup, benefits, and dual usage. Main focus is on configuration and advantages.[159 characters]
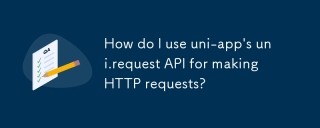
This article details uni.request API in uni-app for making HTTP requests. It covers basic usage, advanced options (methods, headers, data types), robust error handling techniques (fail callbacks, status code checks), and integration with authenticat


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
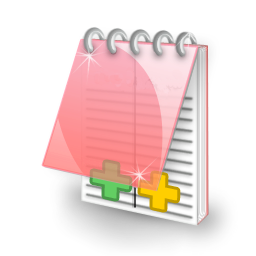
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor