UniApp Download File: How to Handle File Encoding
This question addresses how to manage file encoding during the download process within the UniApp framework. UniApp, being a cross-platform framework, interacts with different operating systems and environments which may handle encoding differently. The key is to ensure the downloaded file retains its original encoding, preventing corruption or display issues. This often involves understanding the encoding of the downloaded file and configuring the download process to respect it. Unfortunately, UniApp itself doesn't directly manage encoding during the download. The responsibility lies primarily with how you handle the received data after downloading it. You will need to rely on JavaScript's built-in encoding handling capabilities. If the server providing the file doesn't specify the encoding (e.g., via Content-Type
header), you might need to detect it based on file content or use a default encoding (but this is prone to errors). The solution usually involves receiving the file as a byte array (e.g., using Uint8Array
) and then using the appropriate JavaScript functions (like TextDecoder
) to decode it correctly based on the known or detected encoding.
How Can I Ensure Downloaded Files Maintain Their Original Encoding in UniApp?
To guarantee that downloaded files retain their original encoding in UniApp, a multi-pronged approach is necessary. First, the server providing the file must accurately specify the encoding in the HTTP response headers, particularly the Content-Type
header. For example, Content-Type: text/plain; charset=utf-8
clearly indicates UTF-8 encoding. Second, your UniApp code must correctly interpret this header. You can access this header using the response.headers
object within the download's success callback. Third, after receiving the file's raw bytes (typically as a Uint8Array
), use JavaScript's TextDecoder
to decode it based on the encoding specified in the Content-Type
header. For example:
uni.request({ url: 'your-download-url', method: 'GET', responseType: 'arraybuffer', // Crucial for receiving raw bytes success: function (res) { const encoding = res.header['content-type'].split(';')[1].split('=')[1]; // Extract encoding from header const decoder = new TextDecoder(encoding); // Create decoder based on encoding const decodedText = decoder.decode(new Uint8Array(res.data)); // Decode the data // Now 'decodedText' contains the correctly encoded string. Handle accordingly. }, fail: function (err) { console.error('Download failed:', err); } });
Remember to handle potential errors, like the Content-Type
header being missing or malformed. Consider providing fallback mechanisms, perhaps using a default encoding (with a clear warning), or prompting the user for encoding information.
What Are the Common Encoding Issues Encountered When Downloading Files with UniApp, and How Can I Solve Them?
Common encoding problems when downloading files with UniApp include:
- Character garbling: This happens when the downloaded file's encoding doesn't match the encoding used for display or interpretation. The solution is accurate encoding detection and decoding as described above.
- Missing characters: Similar to garbling, this indicates a mismatch in encoding. The solution remains accurate header parsing and appropriate decoding.
-
Unexpected symbols: Unrecognized characters appear due to encoding conflicts. Again, proper decoding using
TextDecoder
with the correct encoding is the key. - Incorrect line breaks: Different encodings might handle line breaks differently (e.g., rn vs. n). Ensure consistent line-break handling after decoding.
-
Server-side encoding issues: The server might not be correctly setting the
Content-Type
header, leading to decoding failures on the client side. You'll need to coordinate with the server administrator to fix this.
Solving these problems relies on robust error handling and a clear understanding of encoding mechanisms. Always verify the Content-Type
header and use TextDecoder
to handle different encodings. Consider adding logging to pinpoint where the encoding issue originates.
What Are the Best Practices for Handling Different File Encodings (e.g., UTF-8, GBK) When Downloading Files Using UniApp?
Best practices for handling diverse file encodings in UniApp downloads involve:
-
Prioritize server-side encoding specification: Ensure the server reliably sets the
Content-Type
header correctly for all files. This is the most crucial step. -
Robust header parsing: Implement error handling when extracting the encoding from the
Content-Type
header. Handle cases where the header is missing or malformed gracefully. -
Use
TextDecoder
: Always use theTextDecoder
API to decode the raw byte data based on the detected encoding. This ensures proper handling of various encodings. -
Provide fallback mechanisms: Implement a fallback mechanism, such as using a default encoding (e.g., UTF-8) if the
Content-Type
header is unavailable or invalid. Inform the user about this fallback. - Testing and logging: Thoroughly test your download and decoding logic with various file encodings. Include logging to track encoding information and identify potential issues.
- Consider a library: For more complex scenarios or if you need advanced encoding detection capabilities, explore JavaScript libraries that specialize in encoding handling.
- User feedback: If possible, allow users to specify the encoding if automatic detection fails. This adds flexibility but adds complexity.
By following these best practices, you can ensure reliable and consistent handling of various file encodings when downloading files within your UniApp applications. Remember that the responsibility for accurate encoding primarily lies with the server, but your client-side code needs to be robust enough to handle various situations and potential errors.
The above is the detailed content of How to handle file encoding with UniApp download. For more information, please follow other related articles on the PHP Chinese website!
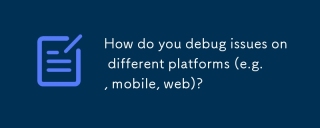
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
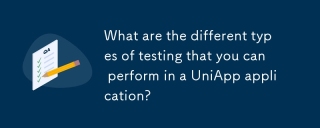
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
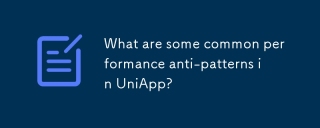
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
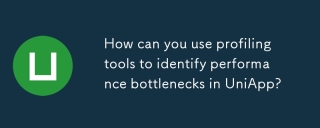
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
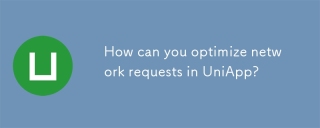
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
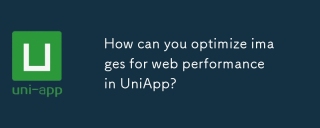
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
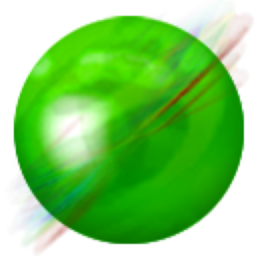
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
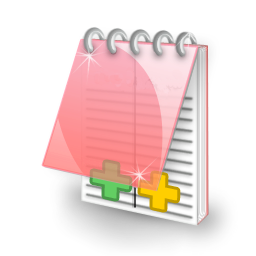
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.