Understanding export default
and Vue Component Templates
This article addresses common questions regarding the use of export default
with templates in Vue.js components. We'll explore its configuration, best practices, and impact on reusability and performance.
Vue中export default如何配置组件的template
In Vue.js, export default
is used to export the default component from a .vue
file or a JavaScript file containing a component definition. When it comes to configuring the template, you don't directly configure export default
to handle the template. Instead, export default
exports the entire component object, which includes the template. The template itself can be defined in several ways:
1. Using the <template></template>
tag within a .vue
single-file component: This is the most common and recommended approach. The <template></template>
tag neatly encapsulates your component's HTML structure.
<template> <div> <h1 id="My-Component">My Component</h1> <p>{{ message }}</p> </div> </template> <script> export default { data() { return { message: 'Hello from the template!' } } } </script>
2. Using the template
option within the export default
object (in a .js
file): This approach is less common but works when you're defining components entirely within JavaScript files.
export default { name: 'MyComponent', template: ` <div> <h1 id="My-Component">My Component</h1> <p>{{ message }}</p> </div> `, data() { return { message: 'Hello from the template!' } } };
In both cases, export default
simply exports the entire object containing the template
(along with data
, methods
, computed
, etc.). It doesn't directly manage the template; it's a container for the whole component definition.
How can I use export default
to effectively structure my Vue component's template for better organization?
export default
itself doesn't directly influence template organization. However, using it in conjunction with good component structuring practices leads to better organization. This includes:
-
Single-File Components (.vue): Leverage the
<template></template>
,<script></script>
, and<style></style>
sections within.vue
files for clear separation of concerns. This improves readability and maintainability. -
Component Composition: Break down complex templates into smaller, reusable sub-components. Import and use these sub-components within your main component's template. Each sub-component can have its own
export default
. -
Scoped Styles: Use scoped styles (e.g.,
<style scoped></style>
) within your.vue
files to prevent CSS conflicts between components. -
Template Syntax: Utilize features like
v-for
,v-if
, and other directives to create dynamic and well-structured templates. -
Code Splitting: For large applications, consider code splitting techniques to load only necessary components on demand. This doesn't directly involve
export default
, but it improves performance and organization.
What are the best practices for using export default
when defining the template within a Vue component?
Best practices center around maintaining clean and maintainable code:
-
Always use
export default
for single components: This makes it clear which component is the primary export of the file. -
Prefer
.vue
single-file components: They offer the best organization and maintainability for templates, scripts, and styles. - Keep templates concise and focused: Avoid overly complex templates within a single component. Break down complex logic into smaller, reusable components.
- Use consistent indentation and formatting: This improves readability and maintainability across your project.
- Use meaningful component names: Choose descriptive names that reflect the component's purpose.
- Thoroughly test your components: Ensure your templates render correctly and interact as expected with the component's logic.
Does using export default
with a template in a Vue component affect its reusability or performance?
No, using export default
itself doesn't directly impact reusability or performance. Reusability depends on how well you design and structure your component, independent of the export default
mechanism. Similarly, performance is largely affected by factors such as component size, rendering complexity, and data handling, not by the use of export default
. export default
is simply a mechanism for exporting the component, it doesn't inherently add overhead. However, poorly written components (regardless of how they're exported) will negatively affect reusability and performance.
The above is the detailed content of How to configure the template of the component in Vue. For more information, please follow other related articles on the PHP Chinese website!

Vue.js and React each have their own advantages in scalability and maintainability. 1) Vue.js is easy to use and is suitable for small projects. The Composition API improves the maintainability of large projects. 2) React is suitable for large and complex projects, with Hooks and virtual DOM improving performance and maintainability, but the learning curve is steeper.

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
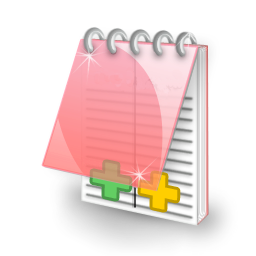
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
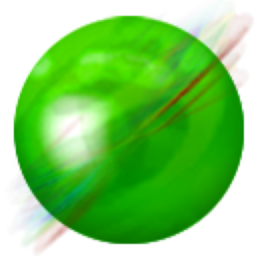
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
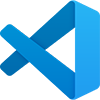
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
