Key Points
- PHP's type prompt allows developers to specify the expected data type of parameters in the function declaration, thereby enhancing the robustness and readability of the code. However, PHP's type prompt does not support scalar data types, such as strings or integers.
- PHP is a weakly typed language, which means it does not require you to declare data types. The addition of scalar PHP type hints in PHP 5.4 have been controversial, and opponents believe this is contrary to the basic design of PHP. Although initially supported, this feature is not entering PHP 5.4 due to community responses.
- Although there are limitations in PHP's type hints, you can perform basic verification to achieve this when the parameter is not a scalar type. In this case, functions such as
is_string()
oris_int()
can be used to raise an error or throw an exception.
Starting with PHP 5, you can use type prompts to specify the expected data type of parameters in a function declaration. When calling a function, PHP will check whether the parameter is of the specified type. If not, the runtime will raise an error and stop execution. Valid types include the class name of the receiving object and the array
of the receiving array. Here is an example:
<?php function enroll(Student $student, School $school) { echo "Enrolling " . $student->name . " in " . $school->name; } ?>
By telling the PHP enroll()
method what type of object you expect to receive, you can ensure that students are registered to a school rather than a monastery or 401K plan. Again, you know there won't be any stubborn pachyderms appearing on the first day of third grade. What happens if I try to register myself with Medicare?
<?php $me = new Student("Amanda"); $medicare = new Program("Medicare"); $enroll = enroll($me, $medicare); ?>
Although I am a student, the following error occurs:
<code>Catchable fatal error: Argument 2 passed to enroll() must be an instance of School, instance of Program given, called in typehint.php on line 32 and defined in typehint.php on line 6</code>
If <cod>null</cod>
is used as the default value of the parameter, it will also be allowed. Here is an example, this time using an array:
<?php function startParty(array $guests, array $food = null) { // party stuff... } $guests = array("Susan Foreman", "Sarah Jane Smith", "Rose Tyler", "Donna Noble"); startParty($guests, null); ?>
As long as there are guests, there will be parties regardless of whether there is food or not.
Limitations of Type Tips
Any defined class can be a valid type prompt, although PHP does not support type prompts for generic objects. What about other situations? Here is a special example about the limitations of PHP type prompts:
<?php function stringTest(string $string) { echo $string; } stringTest("definitely a string"); ?>
<code>Catchable fatal error: Argument 1 passed to stringTest() must be an instance of string, string given, called in typehint.php on line 42 and defined in typehint.php on line 39</code>
You are not the first to think of "What's crazy? I gave you a string instance, but you complain that it must be a string instance!" It doesn't matter. This is a common thing. In fact, at first glance, this can be a rather confusing error message. stringTest()
is not looking for strings, but for instances of string classes. PHP's type prompt does not support scalar data types, such as strings or integer values. But that's OK! If you need to raise an error or throw an exception when the argument is not a scalar type (such as a string or integer), you can perform basic verification using functions such as is_string()
or is_int()
to achieve this.
Scalar War
There are some controversies about adding scalar PHP type prompts to PHP 5.4. Opponents of the change believe that this support would violate the basic design of PHP. PHP is considered a weak-type language. Essentially, this means that PHP does not require you to declare the data type. Variables are still associated with the data type they are associated with, but you can do something radical, such as adding strings to integers without causing errors. In May 2010, support for scalar type hints was added to the PHP backbone. But due to community responses, this feature will not enter version 5.4.
Summary
Type prompts are the introduction of PHP technology for object-oriented programming (especially for identifying types that catch exceptions). I encourage you to read more about using objects here. Pictures from Carlos E. Santa Maria / Shutterstock
PHP Type Tips FAQ (FAQ)
What is the importance of PHP type prompt?
Type prompts in PHP are an important feature that allows developers to specify the expected data type of parameters in function declarations. It enhances the robustness of the code by ensuring that the function receives the correct type of numeric values. This results in fewer bugs and vulnerabilities in the code, making it more reliable and easier to debug. It also improves the readability of the code, making it easier for other developers to understand the functions of the code.
Can I use type prompts for all data types in PHP?
PHP supports type prompts for a variety of data types, including objects, arrays, interfaces, callables, and iterables. However, it should be noted that PHP does not support scalar type prompts (int, float, string, and bool) until version 7.0. Starting with PHP 7.0, you can use type prompts for all data types.
What happens if the data type of the passed parameter does not match the type prompt?
PHP will throw a "fatal error" if the data type of the passed parameter does not match the type prompt in the function declaration. This error will stop the execution of the script and prevent any further processing. This is why you have to ensure that the correct data type is passed to the function.
How does PHP type prompt work with class inheritance?
In PHP, type prompts can be used with class inheritance. If a function expects parameters of a specific class type, it will also accept instances of subclasses of that class. This is because a subclass is a specific type of its parent class, thus satisfying the type hint.
Can I use type prompts in PHP's built-in functions?
No, the type prompt in PHP can only be used for user-defined functions. Type prompts are not supported by built-in functions in PHP. However, these functions often have internal mechanisms for handling different data types.
What is the strict pattern in PHP type prompt?
Strict mode in PHP type prompts is a feature introduced in PHP 7.0. When strict mode is enabled, PHP enforces exact matching of data types based on type prompts. If the data type of the passed parameter does not exactly match the type prompt, PHP will throw a "fatal error" even if the parameter can be cast to the correct type.
How to enable strict mode in PHP?
To enable strict mode in PHP, you need to declare declare(strict_types=1);
at the top of the PHP file. This statement must be the first statement in the script.
What is the difference between weak mode and strict mode in PHP type prompts?
The difference between weak and strict modes in PHP type prompts is how PHP deals with type mismatch. In weak mode, if possible, PHP will attempt to cast the passed parameters to the correct data type. In contrast, in strict mode, PHP will not attempt to cast values, and a "fatal error" will be thrown if the data types do not match exactly.
Can I use type prompts for function return values?
Yes, starting with PHP 7.0, you can use type prompts for function return values. This feature allows you to specify the expected data type of the function return value, thereby enhancing the reliability and readability of your code.
What is the nullable type prompt in PHP?
Nullable type prompt is a feature introduced in PHP 7.1. It allows you to specify the function parameter or return value can be of the specified type or null. To make the type prompt empty, prefix the type name with a question mark (?). For example, "?string" means that the value can be a string or null.
The above is the detailed content of Type Hinting in PHP. For more information, please follow other related articles on the PHP Chinese website!
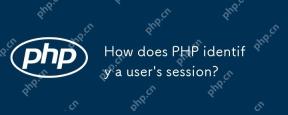
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
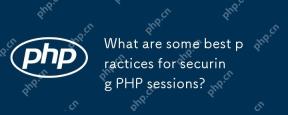
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
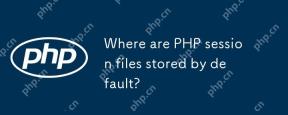
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita
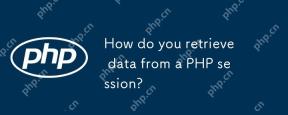
ToretrievedatafromaPHPsession,startthesessionwithsession_start()andaccessvariablesinthe$_SESSIONarray.Forexample:1)Startthesession:session_start().2)Retrievedata:$username=$_SESSION['username'];echo"Welcome,".$username;.Sessionsareserver-si
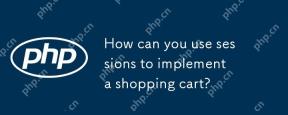
The steps to build an efficient shopping cart system using sessions include: 1) Understand the definition and function of the session. The session is a server-side storage mechanism used to maintain user status across requests; 2) Implement basic session management, such as adding products to the shopping cart; 3) Expand to advanced usage, supporting product quantity management and deletion; 4) Optimize performance and security, by persisting session data and using secure session identifiers.
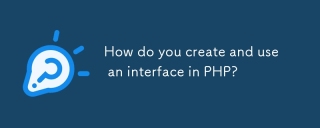
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
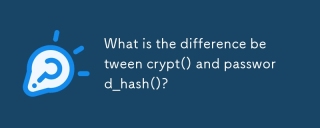
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
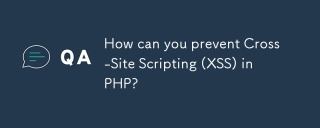
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
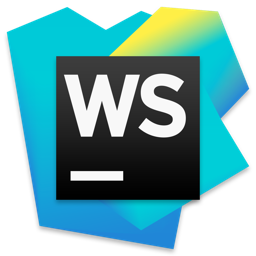
WebStorm Mac version
Useful JavaScript development tools
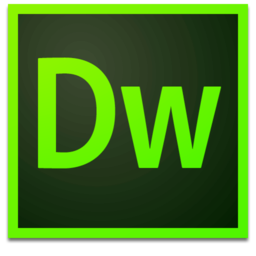
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
