This jQuery code snippet adds drag and touch support for iPads and other touch-enabled devices. It's particularly useful when working with jQuery UI's draggable functionality and floating elements.
//iPad Touch Support $.fn.addTouch = function() { this.each(function(i, el) { $(el).bind('touchstart touchmove touchend touchcancel', function(event) { handleTouch(event); }); }); const handleTouch = function(event) { const touches = event.changedTouches, first = touches[0], type = ''; switch (event.type) { case 'touchstart': type = 'mousedown'; break; case 'touchmove': type = 'mousemove'; event.preventDefault(); break; case 'touchend': type = 'mouseup'; break; default: return; } const simulatedEvent = document.createEvent('MouseEvent'); simulatedEvent.initMouseEvent(type, true, true, window, 1, first.screenX, first.screenY, first.clientX, first.clientY, false, false, false, false, 0, null); first.target.dispatchEvent(simulatedEvent); }; };
Frequently Asked Questions (FAQs): jQuery and iPad Touch Support
This section addresses common questions regarding jQuery and touch event handling on iPads and other touch devices.
Q: How do I add drag/touch support to my iPad app using jQuery?
A: Utilize the jQuery UI Touch Punch. This plugin bridges the gap between touch events and jQuery UI's draggable functionality. Include it after jQuery UI and before any dependent scripts.
Q: Can jQuery recognize touch events in iPad's Safari browser?
A: Yes. jQuery supports touchstart
, touchmove
, touchend
, and touchcancel
events for building touch-enabled web applications.
Q: How can I save a jQuery-edited HTML table to local storage?
A: Convert the table data into a JSON object using jQuery and then store it using localStorage.setItem()
. Retrieve it later with localStorage.getItem()
.
Q: What is jQuery's role in the Shiny Things technology stack?
A: In Shiny Things, jQuery serves as a lightweight, efficient JavaScript library simplifying HTML manipulation, event handling, and animations across various browsers.
Q: How do I use the jQuery.touch plugin?
A: Include the jQuery.touch.js
file in your HTML after the jQuery script. This plugin enhances jQuery with touch events and CSS3 animation capabilities.
Q: How do I troubleshoot jQuery issues on Ubuntu?
A: Check the browser's console for error messages, verify correct jQuery inclusion in your HTML, and ensure your jQuery code is free of syntax errors.
Q: Can I create a touch-enabled slider with jQuery?
A: Yes, use jQuery UI and jQuery UI Touch Punch to add touch support to your slider.
Q: How can I optimize jQuery code for touch devices?
A: Minimize heavy animations, reduce DOM manipulations, and employ event delegation for efficient event handling.
Q: Can jQuery detect iPad touch event types?
A: Yes, jQuery's touch events allow you to identify the specific type of touch interaction.
Q: How can I handle multi-touch gestures with jQuery on an iPad?
A: This is more complex, requiring tracking multiple touch points. Consider using plugins like jQuery MultiSwipe for simplification.
The above is the detailed content of jQuery Add Drag/Touch Support for iPad. For more information, please follow other related articles on the PHP Chinese website!
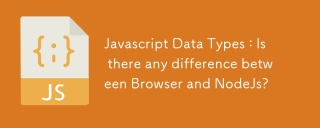
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
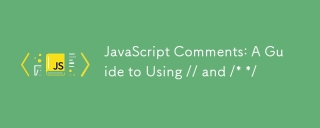
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
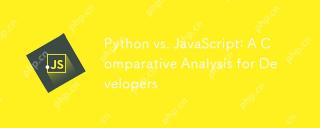
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
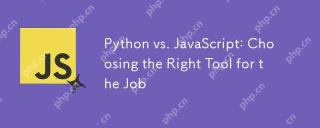
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
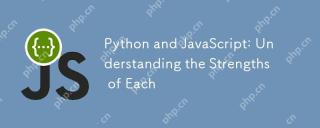
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
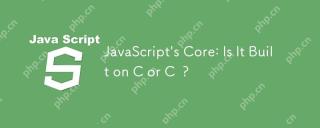
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
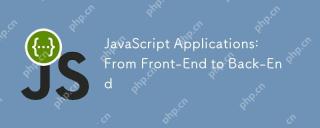
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
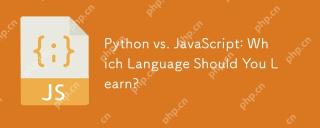
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
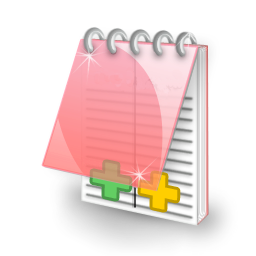
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
