Use
.size()
or.length()
in JavaScript?
Let's take a closer look....size()
is actually just calling .length
(the jQuery source code shown below clearly shows this), so we save a function call:
//https://code.jquery.com/jquery-latest.js // 匹配元素集中包含的元素数量 size: function() { return this.length; },
Tests proved that .length()
is faster than .size()
(jsperf test resultshttps://www.php.cn/link/5208093bcaf65dfea07bdab31d600223 Why are there .size()
functions in jQuery What? My initial guess is that they keep it abstract so that it can provide a backward compatible API if you need a different .length
calculation method in the future. You may have seen common usages:
// 检查 DOM 元素是否存在 if ($('#id').length > 0) { ... }
In short, I will use it all the time unless someone gives me a good reason not to use .length
.
FAQ for JavaScript Size and Length (FAQ)
What is the difference between size and length in JavaScript?
In JavaScript, both size
and length
are used to determine the number of elements in an object. However, they are used for different types of objects. The length
attribute is used for array objects, while the size
attribute is used for Map
and Set
objects. The length
property of the array returns the number of elements in the array. On the other hand, the Map
or Set
object's size
properties return the number of key-value pairs or elements in the object, respectively.
How to determine the size of an object in JavaScript?
Unlike arrays, objects in JavaScript do not have built-in size
or length
properties. However, you can use the Object.keys()
method to determine the size of the object (i.e. the number of attributes it has). This method returns an array of the object's own attribute names. You can then get the length of this array to find out the size of the object.
Can I use the length
attribute with strings in JavaScript?
Yes, you can use the length
attribute with strings in JavaScript. The length
property of a string returns the number of characters in the string, including spaces and special characters.
Why is the length of the JavaScript hollow array 0?
In JavaScript, the length
attribute of the array reflects the number of elements in the array. An empty array contains no elements, so its length is 0.
Can I change the length of an array in JavaScript?
Yes, you can change the length of an array in JavaScript. If you increase the length, a new element with a value of undefined
will be added to the end of the array. If you reduce the length, the element is removed from the end of the array.
What happens if I try to access an array element with an index greater than or equal to the length of the array?
If you try to access an array element with an index greater than or equal to the length of the array, JavaScript will return undefined
. This is because the index is beyond the range of the array.
Can I use the size
attribute with arrays in JavaScript?
No, you cannot use the size
attribute with arrays in JavaScript. The size
attribute is used for Map
and Set
objects, not arrays. To determine the number of elements in an array, you should use the length
attribute.
How to determine the size of Map
or Set
objects in JavaScript?
You can use the size
attribute to determine the size of the Map
or Set
object in JavaScript. This property returns the number of key-value pairs in the Map
object or the number of elements in the Set
object.
Can I use the length
attribute with Map
or Set
objects in JavaScript?
No, you cannot use the length
attribute with Map
or Set
objects in JavaScript. The length
attribute is used for arrays and strings, not Map
or Set
objects. To determine the number of key-value pairs in the Map
object or the number of elements in the Set
object, you should use the size
attribute.
What is the difference between size
attributes and length
attributes in terms of performance in JavaScript?
There is no significant difference between the size
attribute and the length
attribute in JavaScript in terms of performance. Both properties provide a quick and efficient way to determine the number of elements in an object. However, the choice between the two depends on the type of object you are using. The length
attribute is used for arrays and strings, while the size
attribute is used for Map
and Set
objects.
The above is the detailed content of Do I use .size() or .length in Javascript?. For more information, please follow other related articles on the PHP Chinese website!
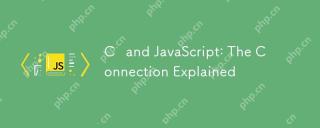
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
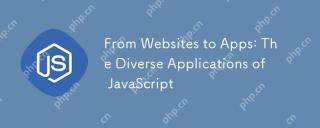
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
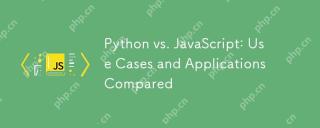
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
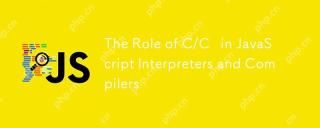
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
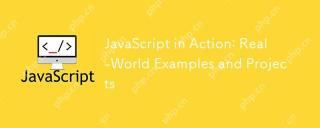
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
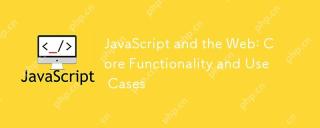
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
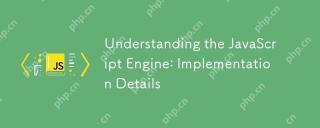
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),