Google Translate API with PHP: A Comprehensive Guide
This guide provides a step-by-step walkthrough on integrating the Google Translate API into your PHP applications. We'll cover account setup, API usage, error handling, and best practices for efficient and cost-effective translation.
Key Concepts:
- API Account & Key: You'll need a Google Cloud Platform (GCP) project, enabled billing, and a generated API key to access the Translate API.
-
API Methods: The Translate API offers three core methods:
translate
(for translation),detect
(for language detection), andlanguages
(for listing supported languages). These are accessed via GET requests. - Pricing: The API is a paid service. Costs are based on character count for translation and detection. Daily usage limits can be set to manage expenses.
- Error Handling: Robust error handling is crucial. Check HTTP response codes and parse JSON error responses for effective debugging.
1. Setting Up Your Google Cloud Project:
- Create a GCP project if you don't already have one.
- Enable the Cloud Translation API within your project.
- Enable billing for your project. This is necessary to use the paid Translate API.
- Create API credentials (an API key) under "Credentials" in the GCP console. Keep this key secure; it's essential for authentication.
2. Accessing the API with PHP:
The Translate API uses GET requests. PHP's curl
library is ideal for making these requests. Remember to URL-encode your parameters using rawurlencode()
.
Example: Checking API Connectivity (Languages Method):
This simple example verifies your API key and connection by retrieving a list of supported languages.
<?php $apiKey = '<YOUR_API_KEY>'; // Replace with your actual API key $url = 'https://translation.googleapis.com/language/translate/v2/languages?key=' . $apiKey; $ch = curl_init($url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); $languages = json_decode($response, true); print_r($languages); ?>
3. Performing Translations (Translate Method):
This example translates "Hello, world!" from English to French.
<?php $apiKey = '<YOUR_API_KEY>'; $text = 'Hello, world!'; $source = 'en'; $target = 'fr'; $url = 'https://translation.googleapis.com/language/translate/v2?key=' . $apiKey . '&q=' . rawurlencode($text) . '&source=' . $source . '&target=' . $target; $ch = curl_init($url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); $responseCode = curl_getinfo($ch, CURLINFO_HTTP_CODE); curl_close($ch); $translation = json_decode($response, true); if ($responseCode == 200) { echo 'Source: ' . $text . '<br>'; echo 'Translation: ' . $translation['data']['translations'][0]['translatedText']; } else { echo 'Error: ' . $responseCode . ' - ' . $response; } ?>
4. Error Handling:
Always check the HTTP response code (curl_getinfo()
). A non-200 code indicates an error. The JSON response will usually contain details about the error.
5. Language Detection (Detect Method):
The detect
method identifies the language of input text. Its usage is similar to the translate
method, but the URL and parameter handling will differ slightly. Refer to the Google Cloud Translation API documentation for the correct parameters.
6. Managing Costs:
- Daily Limits: Set daily character limits in your GCP project to control costs.
- Caching: Cache translations to avoid redundant API calls.
7. Advanced Usage: Consider batch translations for efficiency and exploring other features like glossary support.
8. Security: Never expose your API key directly in client-side code. Use server-side processing to protect your credentials.
This enhanced guide provides a more complete and structured approach to using the Google Translate API with PHP, addressing security and cost management more explicitly. Remember to consult the official Google Cloud Translation API documentation for the most up-to-date information and detailed parameter specifications.
The above is the detailed content of Using Google Translate API with PHP. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
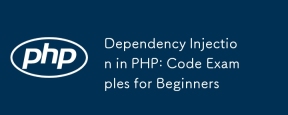
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
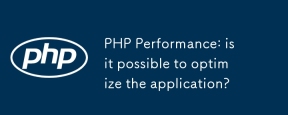
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
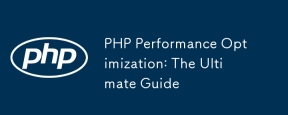
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
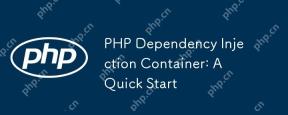
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
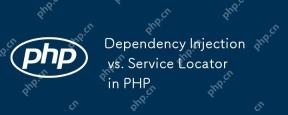
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
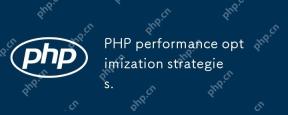
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
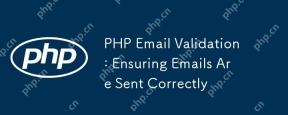
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
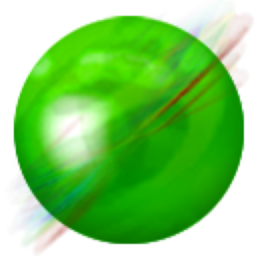
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
