Leveraging ES6 Features in AngularJS Development: A Comprehensive Guide
Key Advantages:
ES6 (ECMAScript 2015) significantly enhances AngularJS development with features like arrow functions, template literals, classes, modules, and promises. These improvements boost code readability, maintainability, and performance.
Development Setup:
Integrating ES6 requires a transpiler (e.g., Babel) to convert ES6 code into browser-compatible ES5. A module bundler (Webpack or Browserify) manages JavaScript modules and dependencies effectively.
ES6 Classes and AngularJS:
ES6 classes streamline component, service, and controller definitions, offering a cleaner syntax for object creation and inheritance. This leads to better code organization and understanding.
Asynchronous Operations with ES6 Promises:
ES6 promises provide a superior alternative to traditional callbacks for handling asynchronous tasks in AngularJS. Wrap asynchronous operations in Promise objects and utilize .then()
and .catch()
for result and error management.
This article demonstrates building an AngularJS application (a simple online bookshelf) using ES6 features and modules. The complete code is available on our GitHub repository.
Bookshelf Application Overview:
This example includes:
- Home Page: Displays active books; allows marking books as read and archiving them.
- Add Book Page: Adds new books (prevents duplicate titles).
- Archive Page: Lists archived books.
ES6 Application Setup:
We use the Traceur client-side library (available via Bower) to transpile ES6 code on the fly. The index.html
includes a script tag:
<🎜>
bootstrap.js
loads the main AngularJS module:
import bookShelfModule from './ES6/bookShelf.main'; angular.bootstrap(document, [bookShelfModule]);
Note: ng-app
isn't used because modules load asynchronously.
Controller Definition:
AngularJS controllers can be defined using $scope
or the controller as
syntax. The latter integrates better with ES6 classes. Private fields are managed using WeakMap
. The HomeController
example illustrates this:
const INIT = new WeakMap(); const SERVICE = new WeakMap(); const TIMEOUT = new WeakMap(); class HomeController { // ... constructor, methods ... } HomeController.$inject = ['$timeout', 'bookShelfSvc']; export default HomeController;
This utilizes ES6 classes, arrow functions, and concise method creation. Dependency injection remains consistent with ES5.
Service Definition:
Services (factories in this case) are defined using a class with a static factory method:
const HTTP = new WeakMap(); class BookShelfService { // ... constructor, methods ... static bookShelfFactory($http) { return new BookShelfService($http); } } BookShelfService.bookShelfFactory.$inject = ['$http']; // ... AngularJS module registration ...
This employs static members and template literals for string concatenation.
Directive Definition:
Directives (like factories) require instance access within the link
function. A WeakMap
again helps manage dependencies. The UniqueBookTitle
directive example demonstrates this:
<🎜>
Main Module and Configuration:
The main module (bookShelf.main.js
) imports controllers, services, and directives, defining routes in the config
block:
import bookShelfModule from './ES6/bookShelf.main'; angular.bootstrap(document, [bookShelfModule]);
Conclusion:
ES6 significantly improves AngularJS development. This guide demonstrates how to leverage its features for cleaner, more maintainable, and performant applications. Remember to consult the GitHub repository for the complete code.
Frequently Asked Questions (FAQs):
(The original FAQs are already well-structured and comprehensive. No significant changes are needed here.)
The above is the detailed content of Writing AngularJS Apps Using ES6. For more information, please follow other related articles on the PHP Chinese website!
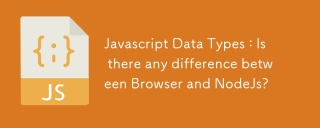
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
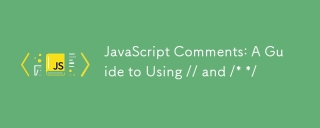
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
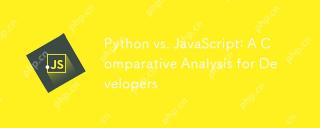
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
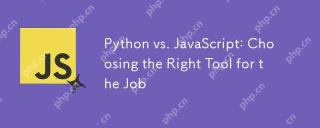
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
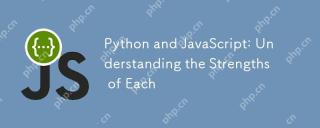
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
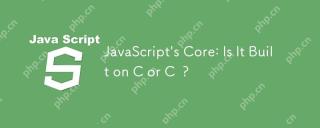
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
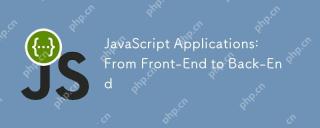
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
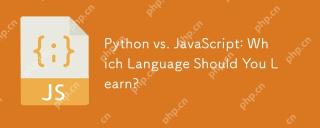
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
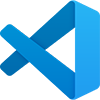
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
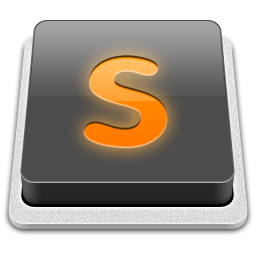
SublimeText3 Mac version
God-level code editing software (SublimeText3)
