Key points for writing self-documented JavaScript code
This article will explore how to write self-documented and maintained self-documented JavaScript code through structured techniques, naming conventions and syntax techniques. While self-documented code can reduce the need for comments, it does not completely replace good comments and comprehensive documentation.
Core skills
- Structured technology: Move the code into a function, replace conditional expressions with functions, and use pure functions to make the code clearer and easier to understand.
- Naming Convention:Name variables, functions, and classes with meaningful names to improve code readability.
- Syntax Skills: Avoid using syntax techniques, use named constants and make the code clearer.
- Extract code with caution: Avoid over-extracting code in pursuit of short functions, which may reduce the comprehensibility of the code.
Technical Overview
We divide the self-documented code into three categories:
- Structure:Use the structure of code or directory to clarify the purpose of the code.
- Name-related: For example, the naming of functions or variables.
- Syntax Related: Use (or avoid) language features to make the code clearer.
Structured technology
-
Move code into function: Move existing code into new function to make its functions clearer. For example,
var width = (value - 0.5) * 16;
can be rewritten as:
var width = emToPixels(value); function emToPixels(ems) { return (ems - 0.5) * 16; }
-
Replace conditional expressions with functions: Convert complex conditional statements into functions to improve readability.
-
Replace expressions with variables: Decompose complex expressions into multiple variables to improve comprehensibility.
-
Class and Module Interfaces: The public methods and properties of a class can be used as documentation of their usage. A clear interface can directly reflect the usage of the class.
-
Code grouping: Grouping related codes can indicate that there is an association between the codes and facilitate maintenance.
-
Use pure functions: Pure functions are easier to understand because their output only depends on input parameters and has no side effects.
-
Directory and file structure: Organize files and directories according to existing naming conventions in the project to facilitate code search and understanding.
Naming skills
-
Function rename: Use verbs in active voice and explicitly indicate the return value. Avoid using vague words such as "handle" or "manage".
-
Variable rename: Use a meaningful name and specify the unit (e.g.
widthPx
). Avoid using abbreviations. -
Adhere to established naming conventions: Maintain a consistent naming style in the project.
-
Use meaningful error messages: Ensure that the error messages thrown by the code are descriptive and contain relevant information that caused the error.
Grammar Skills
-
Avoid using grammar tips: Avoid using difficult-to-understand grammar tips, such as
imTricky && doMagic();
, and use clearerif
statements. -
Use named constants to avoid magic values:Use named constants instead of magic values to improve code readability and maintainability.
-
Avoid Boolean flags: Boolean flags may make the code difficult to understand and should be considered for a clearer approach.
-
Get full use of language features:Use the features provided by languages, such as array iteration methods, to make the code more concise and easy to understand.
Anti-mode
-
Overextracting code for short functions: Avoid overextracting code in order to pursue short functions, which may reduce the comprehensibility of the code.
-
Don't force it: If a method is not suitable, do not force it to use it.
Summary
Writing self-documented code can significantly improve the maintainability of the code and reduce the need for comments. However, self-documented code cannot completely replace documents or comments. Good annotations and API documentation are still crucial for large projects.
The above is the detailed content of 15 Ways to Write Self-documenting JavaScript. For more information, please follow other related articles on the PHP Chinese website!
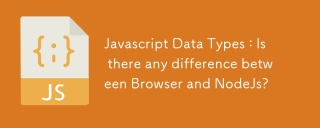
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
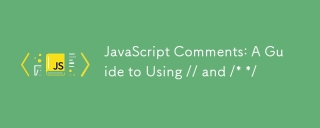
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
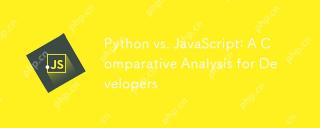
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
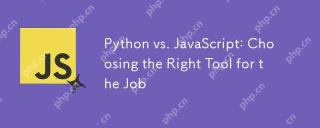
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
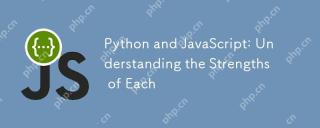
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
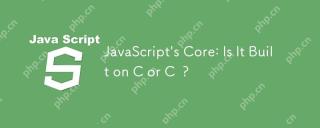
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
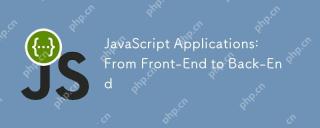
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
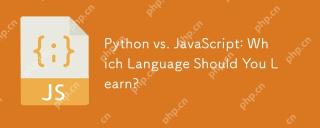
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
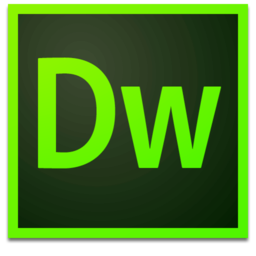
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
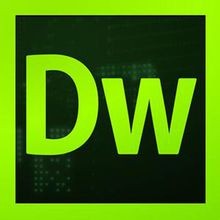
Dreamweaver CS6
Visual web development tools
