This article introduces Flask, a popular Python mini framework that provides an alternative to the huge Django framework.
Flask's design philosophy is lightweight and flexible, allowing developers to quickly and easily create web applications. This article will cover what Flask is, its key features, the advantages of a simple API, its flexibility in supporting third-party extensions, the most commonly used Flask extensions, and when Flask should or should not be used.
Key points
- Flask Features and Use Cases Overview: This article introduces Flask, focusing on its lightweight, flexible features and its applicability to a variety of web projects. It explores Flask's simplicity, extended support, key features, and practical use cases such as prototyping, RESTful APIs, and e-commerce applications.
- Comparative analysis with Django: This article compares Flask with Django, emphasizing Flask's mini-framework approach, which provides the necessary tools without complex structures, which makes it a need for minimalism and self-contained approach. Ideal for defining an extended project.
- Begin with Flask: This article provides a step-by-step guide on setting up a Flask development environment, creating and running basic applications, and leveraging Flask features in routing, templates, forms, and extensions.
What is Flask?
Flask is a miniature web framework written in Python for developing web applications. It is built on a simple concept: keeping it simple and lightweight, providing developers with the most important tools they need to build web applications without unnecessary complexity.
It is built on the Werkzeug WSGI toolkit, which provides the web server functionality required to handle requests and responses, as well as the Jinja2 template engine, which enables Flask to handle HTML templates, allowing developers to create dynamic web applications .
Here are some key features that make Flask a great framework:
- It has a simple API for creating web routing and handling requests.
- Jinja template engine supports HTML templates, allowing developers to easily create web pages.
- Since it supports third-party extensions, it is highly scalable, so developers can install extensions according to the needs of the project.
- It bundles a development server that allows easy testing and debugging of applications.
Overall, Flask provides a powerful, flexible and simple framework to build web applications. It is a great choice for beginners and experienced web developers and is one of the most popular web frameworks in the Python web development ecosystem.
Advantages of Flask
Let us now take a closer look at some of the advantages of using Flask in development.
Simplicity. Flask's design philosophy emphasizes simplicity, which makes it easy for developers of any level to understand and use it. This also means that developers have a very low learning curve because they only need to learn a few concepts and APIs to start building web applications.
Flexibility. Flask's miniature features—providing only the core functionality of the Web framework—enable developers to customize and extend them using Flask extensions or third-party libraries to meet their needs.
Document. The Flask documentation is very comprehensive, covering content from basic to advanced topics, making it easy for developers to learn how to use the framework.
Compatibility. Flask is compatible with various Python versions, which makes it easy to use with existing Python code bases. It also supports multiple web servers, which makes it easy to deploy on a variety of hosting platforms.
Fast development. Flask's simplicity and flexibility reduces the boilerplate code required to set up your application, allowing developers to get started quickly.
Flask is used in many interesting ways on the web. Some notable examples include:
- PgAdmin. The Postgres management interface runs on Flask instances, providing developers with an interface where they can manage their Postgres database.
- Twilio. This is a communications platform that uses Flask in its multiple APIs.
- Pinterest. This photo sharing app uses Flask in its web development stack, allowing its team to easily create some custom features and integrations.
When to use Flask
Flask's simplicity and ease of use make it an excellent choice for a variety of web projects:
- Prototype design. Its ease of use and flexibility makes it an excellent choice for quickly creating prototypes, allowing developers to quickly build and test new features.
- Create a RESTful API. Its own simple API makes it easy to create and process HTTP requests.
- E-commerce application. It is perfect for building online marketplaces and e-commerce platforms.
- Financial. It can be used to build financial applications, including account management, transaction processing, and investment tracking.
- AI. It provides a useful and straightforward way to build and deploy AI training models.
When not to use Flask
While Flask is a great framework and has many advantages and powerful features, in some cases these features can be detrimental to it. Let's explore some projects that are more suitable for other types of frameworks.
Projects that require built-in functions. As a mini framework, Flask only provides the core parts needed to create web applications. If a project requires, say, an administrative interface, authentication, or ORM, then Django is a better choice.
Projects with strict safety requirements. Since Flask is a flexible framework, we must rely on third-party extensions to achieve some level of security in our applications. While this certainly works, it is best to rely on a more tested framework that takes a safer approach, such as Tornado or Twisted.
Projects that enforce certain coding standards. Due to Flask's flexibility, developing applications on it allows developers to create applications in the way they see fit. However, frameworks like Django ensure that developers follow specific conventions, which means developers can easily move from one project to another.
Set up Flask development environment
Let's see how to get started with Flask, from setting up the development environment, to install, and finally launching a minimal application.
Prerequisites
Python must be installed on the development machine. Here are instructions on how to install Python (although we may have installed it).
Create a virtual environment
The virtual environment is an isolated Python environment where we can install packages for a specific project without affecting the global Python installation. (The following is a further discussion on why a virtual environment is useful.) There are different packages that can create virtual environments in Python, such as virtualenv, venv, or Conda.
In this article, we will use virtualenv. We can install it using the following command:
<code>pip install virtualenv</code>
After installing virtualenv, we can create a directory where our Flask application will reside. We can name the directory as we like - except Flask, as this will lead to conflicts. We will name it flask_intro:
<code>mkdir flask_intro</code>
Next, go to that directory so we can start using it:
<code>cd flask_intro</code>
In this directory, let's now create our virtual environment using the following command:
<code>virtualenv myenv</code>
The above command creates a virtual environment and name it myenv. Let's activate it so we can install Flask in it. To activate a virtual environment on Linux or macOS, use the following command:
<code>. myenv/bin/activate</code>
On Windows, use this command:
<code>. myenv\Scripts\activate</code>
Once our virtual environment is activated, it will display the name of the virtual environment on the shell prompt, similar to the output below:
<code>(myenv)/~(path to your project directory)$</code>
In the activated virtual environment, we can continue to install Flask using the following command:
<code>pip install Flask</code>
When Flask is installed, let's go ahead and create a minimal application. We will create a module to save our Flask application. For simplicity, we name it hello.py. On Linux or macOS, we can create this file in our flask_intro directory using the following command:
<code>touch hello.py</code>
The above command creates a file named hello.py. We can also use the development environment to create this file. After creating the file, put the following code into it and save:
<code># hello.py from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!'</code>
In the above code, we import the Flask class from the flask module, then create a Flask instance named app and pass the __name__ variable.
Then we have the route decorator @app.route("/"), which means that the hello_world() function will be fired after someone accesses the root route of the application.
We can run the application in a number of ways, so let's look at some. The first method is to provide multiple parameters to the flask command: --app, then save the name of the module that our Flask application and then run. See below:
<code>flask –app <the module="" name="" of="" your=""> run</the></code>
Run our sample application with the example above:
<code>pip install virtualenv</code>
This will run our application on default port 5000, so the application will be available on http://localhost:5000/ or http://127.0.0.1:5000/. If we want the application to be available on other ports, we can specify the port using the -p or --port option. For example:
<code>mkdir flask_intro</code>
This will run the server on port 8080. Another way we can run the application is to just use the flask run command. However, in order to be able to do this, we need to tell Flask the name of the module that holds the Flask instance, which we do by setting the FLASK_APP environment variable. So, in our case, the Flask application is included in a file called hello.py. So we can set it like this:
<code>cd flask_intro</code>
Now that we have set the FLASK_APP environment variable, we can run the development server like this:
<code>virtualenv myenv</code>
Using this code, we now run a web application. This demonstrates the core philosophy of Flask: we don't need a lot of boilerplate code to make things go smoothly. However, the application we set up above is not very practical or useful, as it only renders the string "Hello World!" on our webpage. To do anything more useful, we can turn to templates. Next we'll see how to deal with them.
Flask template
Flask template is a way to create dynamic web pages that can display different content based on various factors, such as data from a database or user input. Templates in Flask are a combination of HTML and special placeholders (called template variables) that are replaced with actual values at runtime.
Template is stored in the templates directory. Therefore, to use templates, we need to import the render_template() method from flask. The render_template() method accepts the template name and any optional data that needs to be passed to the template.
Let's look at an example function that uses templates to render web pages:
<code>. myenv/bin/activate</code>
In the example above, we have a view function - index() - which is bound to the root URL ("/") via the @app.route() decorator. The function has two variables, title and message. Finally, we pass the template index.html to render_template(), as well as the title and message variables.
In order for the above code to work, we need to have an index.html template in the templates directory. Therefore, the template will look like this:
<code>. myenv\Scripts\activate</code>
In the index.html file, placeholders {{title}} and {{message}} are replaced with values passed to the template in the render_template() method.
templates can also contain more complex logic, such as if statements and for loops, which allow more dynamic pages to be generated.
Therefore, templates in Flask provide developers with a very powerful option to create dynamic web pages full of user-generated information.
Flask routing
Most web applications will have multiple URLs, so we need to have a way to know which function handles which URL. In Flask, this mapping is called routing—the process of binding or mapping URLs to view functions. Bind URLs to view functions allow the application to handle different types of requests, such as GET, POST, PUT, DELETE, etc. It also enables the application to handle multiple requests from different clients.
To set up the route in Flask, we use the route() decorator. The decorator binds the URL to the view function—so when the user accesses a URL that exists in the application, Flask triggers the associated view function to handle the request.
Let's take an example:
<code>pip install virtualenv</code>
In the example above, we define an about URL (/about). When the application receives a request for the about URL, Flask calls the about() function, which returns the string "This is the about page".
So far, even though these examples return different pages, they all use only GET HTTP requests. To be able to handle any specific request, we can specify the HTTP method as an optional parameter to the route() decorator.
Let's look at an example of a PUT request:
<code>mkdir flask_intro</code>
In this example, we define a route to handle PUT requests to update user details given its user_id. We use
In the update_user() function, we use the request.get_json() method to get user data from the request body. We perform certain actions on user data, such as updating users in the database, and then returning a response indicating success or failure and an HTTP status code (in this case 200, indicating success).
In general, routing allows Flask to be able to handle different types of requests and allows our applications to process and process data in different ways based on the URLs accessed by the user.
Flask Forms and Verification
In addition to displaying data for users, the Flask template can also receive user input for further processing or storage. To do this, Flask provides built-in support for processing HTML forms and handling user input. Flask forms are based on the WTForms library, which provides a flexible and powerful way to process form data and perform validation. However, the library is not part of a standard Flask installation, so we need to install it using the following command:
<code>cd flask_intro</code>
After installing WTForms, to use forms in Flask, we need to define a form class that will inherit from flask_wtf.FlaskForm. This class will contain fields on the form and any validation rules that should be applied to them.
Let's look at an example of a login form:
<code>virtualenv myenv</code>
In the example above, we define a login form with two fields - email and password - and a submit button. We also have a validators parameter that specifies validation rules for each field. For example, in this case, we require that the email field contain a valid email address and the password field contains a password of at least six characters.
After defining the form class, we can use it in the login view function to render the form and process the form data submitted by the user. Let's look at an example of a view function:
<code>pip install virtualenv</code>
In the example above, we have a login view that accepts two HTTP methods (GET and POST), so when the user accesses the URL from the browser, LoginForm will render as an HTML form using the render_template method, when the user submits the form When we use the validate_on_submit method to check whether the form is valid. If the form is valid, we will access the email and password.
login.html form may look like this:
<code>mkdir flask_intro</code>
The above template will render the email and password fields and their labels, as well as a submit button with the text "Login". The form.csrf_token field is included to prevent cross-site request forgery (CSRF) attacks. The {% for %} loop is used to display any validation errors that may occur.
By using Flask forms, we have a powerful way to process user input and we will be able to verify the data they entered.
Flask extension
As we can see, Flask is a miniature framework that contains only the most important parts needed to create a web application. However, if we need to add the functionality that Flask itself does not provide, we need to add packages to the installation. Flask extensions are our way of providing this additional feature. We can simply install the required packages. The Flask community has created many extensions.
The following are some of the most popular extensions:
- Flask-SQLAlchemy: Provides integration with the SQLAlchemy toolkit to easily interact with the database.
- Flask-Login: Provides user authentication and session management for Flask.
- Flask-Mail: Provides a simple interface to send emails from Flask.
The Flask community has created hundreds of extensions to handle different features. Using extensions is usually simple. First, we need to install the required extensions using pip.
Let's look at an example using Flask-SQLAlchemy. First, we need to install it:
<code>cd flask_intro</code>
Next, we need to configure it. For example:
<code>virtualenv myenv</code>
In the example above, we have a User model with username and email fields. We also configured SQLALCHEMY_DATABASE_URI, instructing us to use the SQLite database located in example.db. After setting up, we can now use db objects to interact with the database. For example, we could create a new user and add it to the database as follows:
<code>. myenv/bin/activate</code>
With the Flask extension, our applications are able to have more features than they would normally be implemented with core Flask.
Conclusion
In this article, we introduce Flask, a lightweight and flexible Python web framework. We discuss the advantages of using Flask for web development, including its simplicity, flexibility, and ease of use. We also covered how to set up a development environment, create routes, use templates, process forms, and use extensions such as Flask-SQLAlchemy.
All in all, Flask is an excellent choice for building web applications of any size, from small personal projects to large commercial applications. It is easy to learn and use, but also offers advanced features through its numerous extensions.
If you are interested in learning more about Flask, here are some additional resources:
- Flask Document
- Flask Mega-Tutorial by Miguel Grinberg
- Miguel Grinberg's Flask Web Development
- A simple Flask application tutorial
- Real Python Flask Tutorial
If you want to learn more about Django and Flask and their best use cases, check out Python Web Development with Django and Flask.
You can also view these free Flask boilerplates to build your first web application.
FAQs about Flask (Python Framework)
What is Flask?
Flask is a miniature web framework for Python. It is designed to be lightweight and easy to use, making it an excellent choice for developing web applications and APIs.
How to install Flask?
You can install Flask using pip (Python's package manager). Use the command <code>pip install Flask</code> to install Flask on your system.
What are the key features of Flask?
Flask is known for its simplicity, flexibility and minimalism. It provides features such as URL routing, request processing, and template rendering, while allowing developers to select and integrate other components as needed.
How does Flask compare to other Python web frameworks such as Django?
Flask is a miniature framework, while Django is a full-stack web framework. Flask offers greater flexibility and freedom to choose your components, while Django comes with a lot of built-in features and conventions.
Can I build a RESTful API with Flask?
Yes, Flask is great for building RESTful APIs. Its simplicity and support for HTTP methods make it a popular choice for creating API endpoints.
Is Flask suitable for large web applications?
Flask can be used for large applications, but it may require more manual configuration and component integration than full-stack frameworks like Django.
The above is the detailed content of Getting Started with Flask, a Python Microframework. For more information, please follow other related articles on the PHP Chinese website!
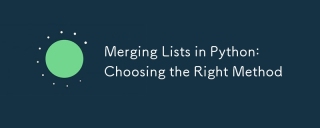
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
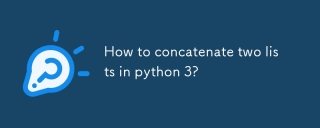
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
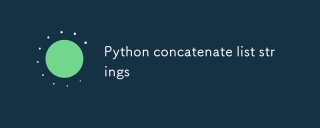
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
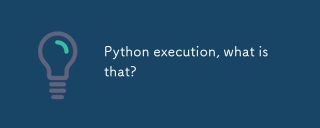
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
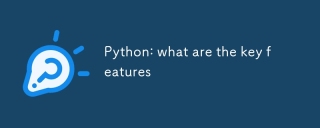
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
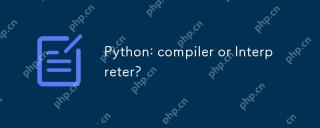
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
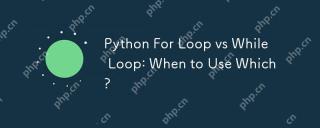
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
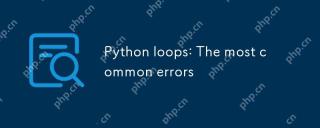
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
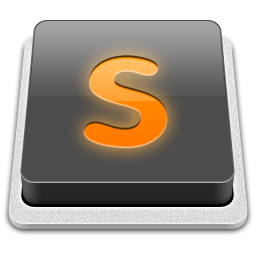
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
