Node.js application debugging: a comprehensive guide. Sooner or later, your Node.js application will encounter errors. Ideally, these errors will be accompanied by clear messages. However, sometimes errors manifest subtly, producing unexpected results, or even worse, silently causing catastrophic damage. This guide explores effective debugging strategies.
Key Concepts
- Master advanced Node.js debugging tools such as the V8 Inspector and VS Code's integrated debugger for efficient code stepping, variable inspection, and breakpoint management.
- Leverage environment variables (e.g.,
NODE_ENV=development
) and command-line options (e.g.,--inspect
) to enable detailed debugging features and enhance application transparency. - Implement strategic logging using
util.debuglog
or third-party modules like Winston to capture detailed, context-specific logs for thorough analysis. - Embrace test-driven development (TDD) and utilize linters like ESLint to proactively identify and address bugs early in development, improving code quality and reliability.
- Utilize Chrome DevTools for Node.js applications (via the
--inspect
flag) for a familiar debugging environment, facilitating effective inspection of call stacks, variable states, and control flow.
Understanding Debugging
Debugging is the process of identifying and resolving software defects. While fixing a bug is often straightforward, locating the root cause can be time-consuming. Node.js offers powerful tools to streamline this process.
Debugging Terminology
Term | Explanation |
---|---|
Breakpoint | A point in the code where the debugger pauses execution, allowing inspection of the program's state. |
Debugger | A tool providing debugging functionalities, such as stepping through code line by line and inspecting variables. |
Feature (not bug) | A common developer phrase used to jokingly dismiss a reported bug. |
Frequency | How often a bug occurs under specific conditions. |
"It doesn't work" | A vague and unhelpful bug report. |
Log Point | An instruction to the debugger to display a variable's value at a specific point during execution. |
Logging | Outputting runtime information to the console or a file. |
Logic Error | The program runs without crashing, but produces incorrect results. |
Priority | The ranking of a bug's importance in the list of planned updates. |
Race Condition | A hard-to-trace bug caused by the unpredictable sequence or timing of events. |
Refactoring | Rewriting code to improve readability and maintainability. |
Regression | The re-emergence of a previously fixed bug, often due to subsequent code changes. |
Related Bug | A bug similar to or connected to another bug. |
Reproduce | The steps needed to trigger the error. |
RTFM Error | User error disguised as a bug report (Read The Flipping Manual). |
Step Into | In a debugger, execute a function call line by line. |
Step Out | In a debugger, complete the current function's execution and return to the calling code. |
Step Over | In a debugger, execute a command without stepping into any functions it calls. |
Severity | The impact of a bug on the system (e.g., data loss is more severe than a minor UI issue). |
Stack Trace | A historical list of all functions called before an error occurred. |
Syntax Error | Errors caused by typos or incorrect code structure (e.g., console.lug() ). |
User Error | An error caused by user actions, but may still require a fix depending on the user's role. |
Watch | A variable monitored during debugger execution. |
Watchpoint | Similar to a breakpoint, but the program pauses only when a specific variable reaches a particular value. |
Preventing Bugs
Proactive measures can significantly reduce bug occurrences.
Utilize a Robust Code Editor
A good code editor offers features like line numbering, auto-completion, syntax highlighting, bracket matching, formatting, and more, improving code quality and reducing errors. Popular choices include VS Code, Atom, and Brackets.
Employ a Code Linter
Linters identify potential code issues (syntax errors, indentation problems, undeclared variables) before testing. ESLint, JSLint, and JSHint are popular options for JavaScript and Node.js. They can be run from the command line (eslint myfile.js
) or integrated into code editors.
Leverage Source Control
Source control systems (e.g., Git) track code changes, making it easier to identify when and where bugs were introduced. Online repositories like GitHub and Bitbucket provide convenient tools and storage.
Implement an Issue-Tracking System
An issue-tracking system helps manage bug reports, track duplicates, document reproduction steps, assign priorities, and monitor progress. Many online repositories include basic issue tracking, but dedicated solutions are better for larger projects.
Adopt Test-Driven Development (TDD)
TDD involves writing tests before the code, ensuring functionality and catching issues early.
Take Breaks
Stepping away from debugging for a while can often lead to fresh insights and solutions.
Node.js Debugging: Environment Variables
Environment variables control Node.js application settings. NODE_ENV
is commonly set to development
during debugging. Variables can be set on Linux/macOS (NODE_ENV=development
), Windows cmd (set NODE_ENV=development
), or Windows PowerShell ($env:NODE_ENV="development"
). They can also be stored in a .env
file and loaded using the dotenv
module.
Node.js Debugging: Command-Line Options
Command-line options modify the Node.js runtime behavior. --trace-warnings
outputs stack traces for warnings (including deprecations). Other options include --enable-source-maps
, --throw-deprecation
, and --inspect
.
Console Debugging
console.log()
is a basic but essential debugging tool. However, explore other console
methods: .dir()
, .table()
, .error()
, .count()
, .group()
, .time()
, .trace()
, and .clear()
. ES6 destructuring simplifies logging complex objects.
Node.js util.debuglog
util.debuglog
conditionally writes messages to STDERR, only activated when the NODE_DEBUG
environment variable is set appropriately. This allows for leaving debug statements in code without cluttering the console during normal operation.
Debugging with Log Modules
Third-party logging modules (cabin, loglevel, morgan, pino, signale, etc.) offer advanced features like logging levels, verbosity control, file output, and more.
Node.js V8 Inspector
The V8 Inspector is a powerful debugging tool. Start an application with node inspect ./index.js
. Commands include cont
(continue), next
(next command), step
(step into), out
(step out), pause
, watch
, setBreakpoint()
, and .exit
.
Node.js Debugging with Chrome
Use node --inspect ./index.js
to start the inspector, listening on port 9229. Open Chrome's chrome://inspect
and click "inspect" to attach DevTools. Set breakpoints, watch variables, and inspect the call stack. For remote debugging, use node --inspect=0.0.0.0:9229 ./index.js
.
Node.js Debugging with VS Code
VS Code provides integrated Node.js debugging. Set breakpoints by clicking in the gutter, or use conditional breakpoints and logpoints. For remote debugging or advanced configurations, use a launch.json
file.
Other Node.js Debugging Tools
Explore other IDEs (Visual Studio, JetBrains, WebStorm), extensions (Atom's node-debug
), ndb, IBM report-toolkit, and commercial services like LogRocket and Sentry.io.
Conclusion
Node.js offers a rich set of debugging tools. Mastering these tools significantly improves development speed and application reliability. While console.log()
remains useful, leverage the more advanced options for efficient debugging.
Frequently Asked Questions (FAQs)
- What tools can I use? Built-in debugger, Node.js Inspector, VS Code debugger, ndb, node-debug.
-
How to start with the built-in debugger? Use
node inspect your-script.js
ornode inspect-brk your-script.js
. -
Difference between
inspect
andinspect-brk
?inspect
attaches after startup;inspect-brk
breaks at the beginning. -
How to set breakpoints? Use the
debugger;
statement, the debugger's commands, or click in the editor's gutter (in IDEs). -
Purpose of
console.log()
? Output information to the console for inspection. -
Debugging asynchronous code? Use
async/await
and set breakpoints within async functions. -
Debugging performance issues? Use
--inspect
, flamegraphs, and profiling tools likeclinic.js
. - Remote debugging? Specify host and port options when starting the debugger and connect from your local environment.
The above is the detailed content of How to Debug a Node.js Application: Tips, Tricks and Tools. For more information, please follow other related articles on the PHP Chinese website!
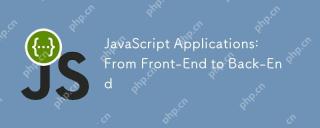
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
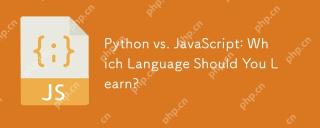
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
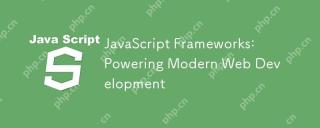
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
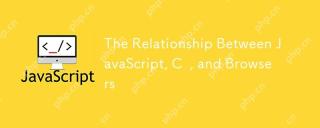
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
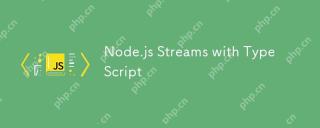
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
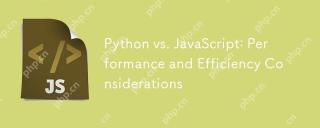
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
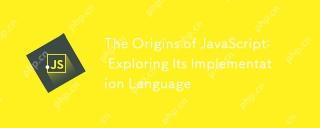
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
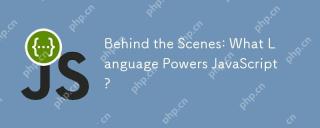
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
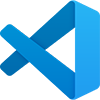
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
