This tutorial demonstrates building a React web application that fetches and displays Pokémon data using GraphQL and Apollo Client. We'll utilize the graphql-pokemon
API.
Key Concepts:
- GraphQL: A query language for APIs, enabling clients to request precisely the data they need. We'll use it to interact with the Pokémon API.
- Apollo Client: A powerful data management solution for JavaScript, simplifying GraphQL integration in React.
-
useQuery
Hook: A React hook from@apollo/react-hooks
for fetching GraphQL query results.
Prerequisites:
- Node.js and npm (or yarn) installed.
- Familiarity with JavaScript (ES6 ), React, and basic terminal commands.
Setup:
-
Create React App:
npx create-react-app react-pokemon cd react-pokemon
-
Install Apollo Client Packages:
npm install graphql apollo-client apollo-cache-inmemory apollo-link-http @apollo/react-hooks graphql-tag
-
Configure Apollo Client (src/index.js):
import { ApolloClient, InMemoryCache, HttpLink, ApolloProvider } from '@apollo/client'; import { BrowserRouter as Router } from 'react-router-dom'; // Add for routing if needed import App from './App'; import ReactDOM from 'react-dom/client'; const client = new ApolloClient({ cache: new InMemoryCache(), link: new HttpLink({ uri: 'https://graphql-pokemon.now.sh/' }), }); const root = ReactDOM.createRoot(document.getElementById('root')); root.render( <React.StrictMode> <ApolloProvider client={client}> <Router> {/* Wrap with Router if using routing */} <App /> </Router> </ApolloProvider> </React.StrictMode> );
-
Fetch Pokémon Data (src/App.js):
import { useQuery, gql } from '@apollo/client'; import React from 'react'; const GET_POKEMON = gql` query pokemons($first: Int!) { pokemons(first: $first) { id name image } } `; function App() { const { loading, error, data } = useQuery(GET_POKEMON, { variables: { first: 150 } }); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <div> <h1 id="Pokémon-List">Pokémon List</h1> {data.pokemons.map((pokemon) => ( <div key={pokemon.id}> <img src={pokemon.image} alt={pokemon.name} /> <h3 id="pokemon-name">{pokemon.name}</h3> </div> ))} </div> ); } export default App;
-
Styling (Optional): Add CSS to
src/App.css
or inline styles to customize the appearance. -
Run the App:
npm start
-
Deploy (Optional): Use a platform like Netlify, Vercel, or GitHub Pages to deploy your built application. Remember to run
npm run build
before deploying.
This revised response provides a more concise and streamlined tutorial, focusing on the core steps. Error handling and variable usage are improved for clarity and robustness. Remember to adjust styling as needed to match your preferences. The inclusion of routing is optional, depending on your application's complexity.
The above is the detailed content of How to Build a Web App with GraphQL and React. For more information, please follow other related articles on the PHP Chinese website!
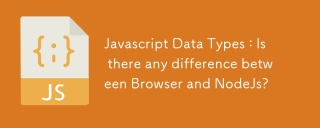
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
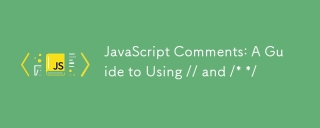
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
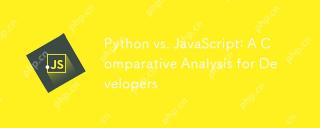
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
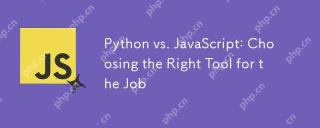
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
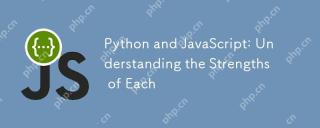
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
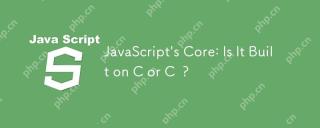
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
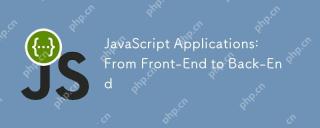
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
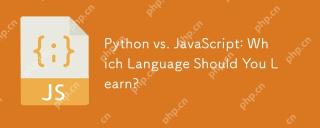
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
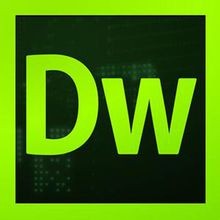
Dreamweaver CS6
Visual web development tools
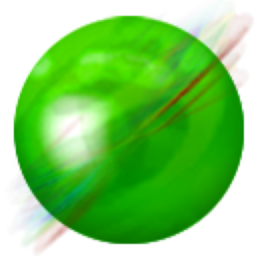
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
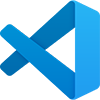
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
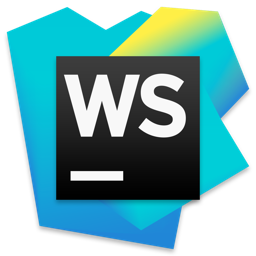
WebStorm Mac version
Useful JavaScript development tools
