Master JavaScript asynchronous operations: Async/Await Detailed explanation
This article explores in-depth async
/await
in JavaScript, teaching you how to efficiently control asynchronous operations and write clearer and easier to read code.
Core points:
-
async
/await
in JavaScript simplifies the processing of asynchronous operations, making the code look like it is executed synchronously, but is still non-blocking.
The -
async
function returns a promise, and theawait
keyword pauses the function execution until the promise is resolved, thereby enhancing the readability of the code and flow control.
The error handling of -
async
functions can be efficiently managed usingtry
/catch
block orPromise
method.catch()
Use - to optimize running asynchronous commands in parallel, allowing multiple
Promise.all
to be resolved simultaneously rather than executed sequentially.Promise
Async iterators and - loops allow the correct processing of
for...of
functions in the loop, ensuring the correct order of execution and better performance management.async
The top layer introduced by ES2022 allows the use of - at the top layer of the ES module, simplifying the integration of asynchronous code without the need for additional wrappers.
await
await
How to create JavaScript asynchronous function
-
- Keywords
-
async
Keywords -
await
Different ways to declare asynchronous functions
/ -
- Underground use Promise
await
async
Switch from Promise to- /
-
async
await
-
- The
- method using function call
-
catch()
Run asynchronous commands in parallel -
- Asynchronous in synchronous loop
-
await
Top Level -
await
Writing asynchronous code confidently
Consider the following code:
The
function fetchDataFromApi() { // 数据获取逻辑 console.log(data); } fetchDataFromApi(); console.log('数据获取完成');JavaScript interpreter does not wait for the asynchronous
function to complete before executing the next statement. Therefore, it will record the actual data returned by the API fetchDataFromApi
. 数据获取完成
and async
keywords to make our program wait for the asynchronous operation to complete before continuing to execute. await
How to create JavaScript asynchronous function
Let's take a closer look at the data acquisition logic in the function. Data fetching in JavaScript is a primary example of asynchronous operations. fetchDataFromApi
function fetchDataFromApi() { // 数据获取逻辑 console.log(data); } fetchDataFromApi(); console.log('数据获取完成');
Here, we get a programming joke from JokeAPI. The response of the API is in JSON format, so after the request is completed (using the json()
method) we extract the response and then log the joke to the console.
Please note that JokeAPI is a third-party API, so we cannot guarantee the quality of the jokes we return!
If we run this code in a browser or in Node (version 17.5 using the --experimental-fetch
flag), we see that the console is still logging the content in the wrong order.
Let's change it.
async
Keywords
The first thing we need to do is to mark the include function as an asynchronous function. We can do this using the async
keyword, and we put it in front of the function
keyword:
function fetchDataFromApi() { fetch('https://v2.jokeapi.dev/joke/Programming?type=single') .then(res => res.json()) .then(json => console.log(json.joke)); } fetchDataFromApi(); console.log('数据获取完成');
Async functions always return a promise (more on this later), so just link then()
to the function call to get the correct execution order:
async function fetchDataFromApi() { fetch('https://v2.jokeapi.dev/joke/Programming?type=single') .then(res => res.json()) .then(json => console.log(json.joke)); }
If we run the code now, we will see something like this:
fetchDataFromApi() .then(() => { console.log('数据获取完成'); });
But we don't want to do this! JavaScript's Promise syntax can be a bit complicated, and that's where async
/await
flashes: it allows us to write asynchronous code using syntax that looks more like synchronous code, and is easier to read.
await
Keywords
The next thing to do is to preface any asynchronous operations in the function with the await
keyword. This will force the JavaScript interpreter to "pause" execution and wait for the result. We can assign the results of these operations to variables:
<code>程序员的浪漫:一行代码解决千行代码的bug。 数据获取完成</code>
We also need to wait for the result of calling the fetchDataFromApi
function:
async function fetchDataFromApi() { const res = await fetch('https://v2.jokeapi.dev/joke/Programming?type=single'); const json = await res.json(); console.log(json.joke); }
Unfortunately, if we try to run the code now, we will encounter an error:
await fetchDataFromApi(); console.log('数据获取完成');
This is because we cannot use async
outside of await
functions in non-module scripts. We'll cover this in more detail later, but now the easiest way to solve this problem is to wrap the call code with its own function, which we also mark as async
:
<code>Uncaught SyntaxError: await is only valid in async functions, async generators and modules</code>
If we run the code now, everything should be output in the correct order:
async function fetchDataFromApi() { const res = await fetch('https://v2.jokeapi.dev/joke/Programming?type=single'); const json = await res.json(); console.log(json.joke); } async function init() { await fetchDataFromApi(); console.log('数据获取完成'); } init();
The fact that we need this extra boilerplate code is unfortunate, but in my opinion the code is still easier to read than the Promise-based version.
Different ways to declare asynchronous functions
The previous example uses two named function declarations (the function
keyword followed by the function name), but we are not limited to this. We can also mark function expressions, arrow functions, and anonymous functions as async
.
If you want to review the differences between function declarations and function expressions, check out our guide
(The following content is the same. Rewrite paragraph by paragraph according to the original text, maintain the original meaning, and adjust the language style to make it smoother and more natural)
The above is the detailed content of A Beginner's Guide to JavaScript async/await, with Examples. For more information, please follow other related articles on the PHP Chinese website!
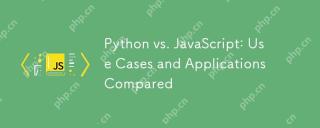
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
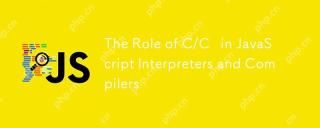
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
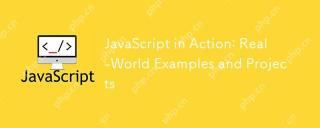
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
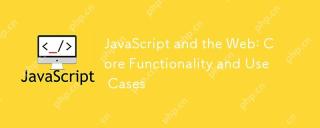
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
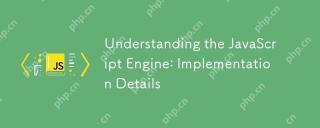
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
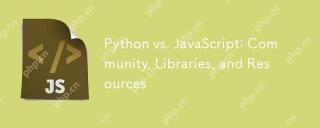
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
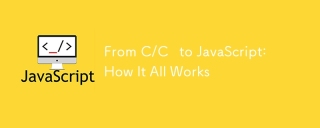
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
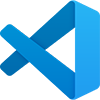
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software