This article demonstrates building and validating a simple web form using HTML and PHP. The form, created with HTML, collects user data (name, address, email, fruit consumption preferences, and brochure request). PHP handles validation and processing. The goal is to illustrate basic HTML form elements and how PHP accesses their data.
The form includes text fields, radio buttons, a multiple-select list, a checkbox, and a submit button. Validation ensures all required fields are filled. Empty fields display "Missing" upon resubmission. Successful submission displays the entered data. The complete code is available on GitHub.
Key Concepts:
- PHP Form Validation: Verifies complete form submission, displaying error messages for missing data.
-
$_POST
Superglobal: Securely retrieves user input from forms using the POST method. -
htmlspecialchars()
: Prevents Cross-Site Scripting (XSS) attacks by converting special HTML characters to their entities. - Persistent User Selections: PHP remembers user choices in radio buttons and select lists for improved user experience.
- Advanced Validation: Suggests using libraries like laminas-validator for more robust validation.
Form Structure:
The HTML form simulates a fruit survey for "The World of Fruit," gathering user details, fruit consumption habits, and brochure preferences.
HTML Elements:
-
Text Fields (name, address, email): Standard text input fields using
<input type="text">
. -
Radio Buttons (howMany): A group of radio buttons (
<input type="radio">
) for selecting fruit consumption amount (0, 1, 2, or more than 2). -
Select List (favoriteFruit): A multiple-select list (
<select multiple></select>
) allowing users to choose multiple favorite fruits. -
Checkbox (brochure): A checkbox (
<input type="checkbox">
) for brochure requests. -
Submit Button: A submit button (
<input type="submit">
) to send the form data. -
Form Element (
<form></form>
): Uses the POST method (method="POST"
) andaction="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>"
to send data to the same script for processing.
PHP Processing:
The PHP code validates the form data. It checks for empty required fields and stores errors in the $errors
array. If no errors, it displays the submitted data. If errors exist, the form is redisplayed with error messages and pre-filled values. The code utilizes $_POST
to access submitted data and htmlspecialchars()
to sanitize input, preventing XSS vulnerabilities. The example provides basic validation; more robust validation (length checks, email format, date validation) is recommended using libraries like laminas-validator for production applications.
CSS Styling:
Simple CSS is used to style error messages:
.error { color: #FF0000; }
Improving User Experience:
The PHP code is enhanced to remember user selections in radio buttons and the select list, improving the user experience upon resubmission.
Summary:
This tutorial provides a foundation for building and validating web forms using HTML and PHP. It emphasizes secure coding practices and highlights the importance of robust validation for data integrity and security. Remember to expand upon the basic validation techniques shown here for real-world applications.
The above is the detailed content of Form Validation with PHP. For more information, please follow other related articles on the PHP Chinese website!
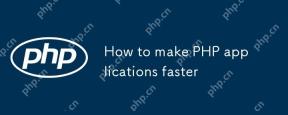
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
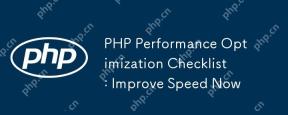
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
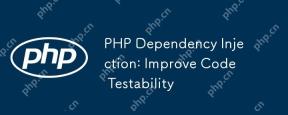
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
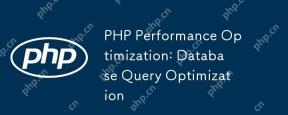
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
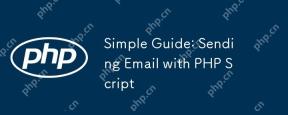
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
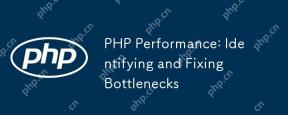
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
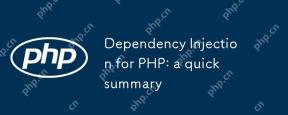
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
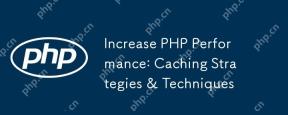
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
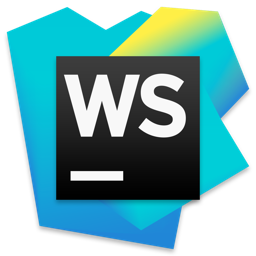
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
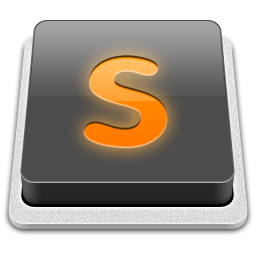
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
