This Java program efficiently removes all even-valued nodes from a singly linked list. Let's refine the explanation and presentation for clarity.
This article demonstrates how to remove all even-numbered nodes from a singly linked list in Java. We'll cover creating the list, adding nodes, deleting even-valued nodes, and displaying the final list.
A singly linked list is a linear data structure where each node points to the next node in the sequence. Each node contains data (in this case, an integer) and a pointer to the next node.
Problem: Delete all even-valued nodes from a singly linked list.
Input Example:
<code>Original List: 1 2 3 4 5 6</code>
Output Example:
<code>Original List: 1 2 3 4 5 6 List after deleting even nodes: 1 3 5</code>
Algorithm:
- Initialization: Create an empty singly linked list.
- Node Insertion: Add nodes with integer values (e.g., 1, 2, 3, 4, 5, 6) to the list.
-
Even Node Removal:
- Remove Leading Evens: Iterate from the head of the list, removing any even-valued nodes at the beginning until an odd-valued node is encountered.
-
Remove Internal Evens: Traverse the remaining list. If a node's
next
node has an even value, bypass it by linking the current node directly to the node after the even-valued node.
- Output: Print the remaining nodes in the list.
Java Code:
public class LinkedList { static class Node { int data; Node next; Node(int data) { this.data = data; this.next = null; } } Node head; public void insert(int data) { Node newNode = new Node(data); if (head == null) { head = newNode; } else { Node temp = head; while (temp.next != null) { temp = temp.next; } temp.next = newNode; } } public void deleteEvenNodes() { //Remove leading even nodes while (head != null && head.data % 2 == 0) { head = head.next; } //Remove internal even nodes if (head != null) { //Check if list is not empty after removing leading evens Node current = head; while (current != null && current.next != null) { if (current.next.data % 2 == 0) { current.next = current.next.next; } else { current = current.next; } } } } public void printList() { Node temp = head; while (temp != null) { System.out.print(temp.data + " "); temp = temp.next; } System.out.println(); } public static void main(String[] args) { LinkedList list = new LinkedList(); list.insert(1); list.insert(2); list.insert(3); list.insert(4); list.insert(5); list.insert(6); System.out.println("Original List:"); list.printList(); list.deleteEvenNodes(); System.out.println("List after deleting even nodes:"); list.printList(); } }
Output:
<code>Original List: 1 2 3 4 5 6 List after deleting even nodes: 1 3 5 </code>
This improved version includes a more concise explanation, clearer code comments, and handles the edge case where all nodes are even (resulting in an empty list). The addition of a check (if (head != null)
) before processing internal nodes prevents a NullPointerException
if all leading nodes were even.
The above is the detailed content of Java program to delete all even nodes from a singly linked list. For more information, please follow other related articles on the PHP Chinese website!
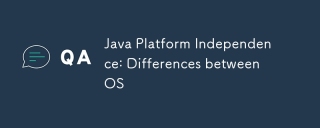
There are subtle differences in Java's performance on different operating systems. 1) The JVM implementations are different, such as HotSpot and OpenJDK, which affect performance and garbage collection. 2) The file system structure and path separator are different, so it needs to be processed using the Java standard library. 3) Differential implementation of network protocols affects network performance. 4) The appearance and behavior of GUI components vary on different systems. By using standard libraries and virtual machine testing, the impact of these differences can be reduced and Java programs can be ensured to run smoothly.
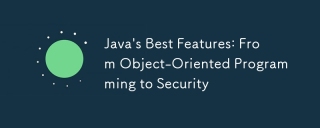
Javaoffersrobustobject-orientedprogramming(OOP)andtop-notchsecurityfeatures.1)OOPinJavaincludesclasses,objects,inheritance,polymorphism,andencapsulation,enablingflexibleandmaintainablesystems.2)SecurityfeaturesincludetheJavaVirtualMachine(JVM)forsand
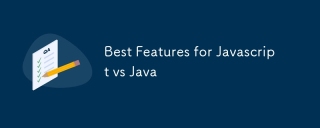
JavaScriptandJavahavedistinctstrengths:JavaScriptexcelsindynamictypingandasynchronousprogramming,whileJavaisrobustwithstrongOOPandtyping.1)JavaScript'sdynamicnatureallowsforrapiddevelopmentandprototyping,withasync/awaitfornon-blockingI/O.2)Java'sOOPf
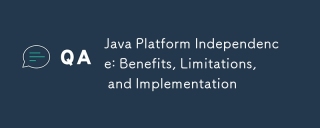
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM)andbytecode.1)TheJVMinterpretsbytecode,allowingthesamecodetorunonanyplatformwithaJVM.2)BytecodeiscompiledfromJavasourcecodeandisplatform-independent.However,limitationsincludepotentialp
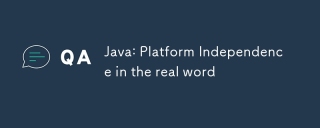
Java'splatformindependencemeansapplicationscanrunonanyplatformwithaJVM,enabling"WriteOnce,RunAnywhere."However,challengesincludeJVMinconsistencies,libraryportability,andperformancevariations.Toaddressthese:1)Usecross-platformtestingtools,2)
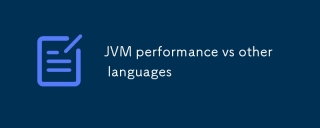
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
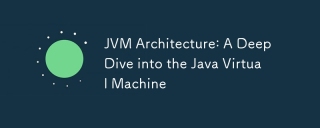
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
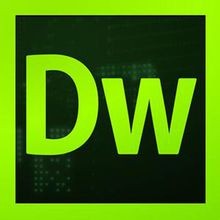
Dreamweaver CS6
Visual web development tools
