Stack is a basic data structure in computer science and is usually used for its Last-in-first-out (LIFO) attribute. When using the stack, you may encounter an interesting problem, which is to check if the elements of the stack are continuous in pairs. In this article, we will learn how to solve this problem using Java to ensure that the solution is efficient and clear.
Problem Statement
Given an integer stack, the task is to determine whether the elements of the stack are continuous in pairs. If the difference between two elements is exactly 1, they are considered continuous.
Enter
<code>4, 5, 2, 3, 10, 11</code>
Output
<code>元素是否成对连续?<br>true</code>
Step to check if stack elements are paired and continuous
The following are steps to check whether the stack elements are paired and continuous:
- Check stack size: If the number of elements on the stack is odd, the last element will not be paired, so it should be ignored for pairwise inspection.
- Pair-based check: Loop through the stack, pop the elements in pairs, and check if they are continuous.
- Restore stack: After performing a check, the stack should be restored to its original state.
Java program used to check whether stack elements are in pairs and contiguous
The following is a program in Java that checks whether stack elements are in pairs and contiguous:
import java.util.Stack; public class PairwiseConsecutiveChecker { public static boolean areElementsPairwiseConsecutive(Stack<Integer> stack) { // 基本情况:如果堆栈为空或只有一个元素,则返回 true if (stack.isEmpty() || stack.size() == 1) { return true; } // 使用临时堆栈在检查时保存元素 Stack<Integer> tempStack = new Stack<>(); boolean isPairwiseConsecutive = true; // 成对处理堆栈元素 while (!stack.isEmpty()) { int first = stack.pop(); tempStack.push(first); if (!stack.isEmpty()) { int second = stack.pop(); tempStack.push(second); // 检查这对元素是否连续 if (Math.abs(first - second) != 1) { isPairwiseConsecutive = false; } } } // 恢复原始堆栈 while (!tempStack.isEmpty()) { stack.push(tempStack.pop()); } return isPairwiseConsecutive; } public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(4); stack.push(5); stack.push(2); stack.push(3); stack.push(10); stack.push(11); boolean result = areElementsPairwiseConsecutive(stack); System.out.println("元素是否成对连续? " + result); } }
Explanation
Restore stack: Since we modified the stack when checking the right, it is very important to restore it to its original state after the check is completed. This ensures that the stack remains unchanged for any subsequent operations.
Edge case: This function handles edge cases, such as an empty stack or a stack with only one element, returning true because these cases satisfy the condition insignificantly.
Time Complexity: The time complexity of this method is O(n), where n is the number of elements in the stack. This is because we only traverse the stack once, popping and pressing elements as needed.
Space complexity: Because the temporary stack is used, the space complexity is also O(n).
Conclusion
This solution provides an efficient way to check if elements in the stack are continuous in pairs. The key is to process the stack in pairs and make sure the stack is restored to its original state after the operation. This approach maintains the integrity of the stack while providing a clear and effective solution.
The above is the detailed content of Check if stack elements are pairwise consecutive in Java. For more information, please follow other related articles on the PHP Chinese website!
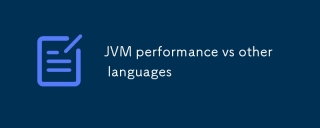
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
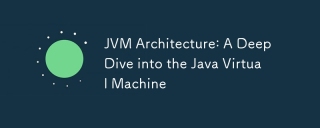
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
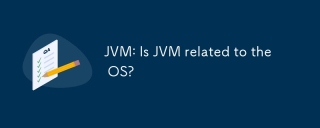
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
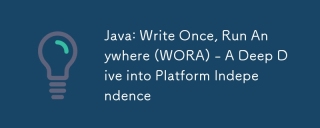
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
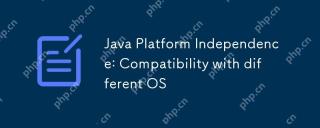
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
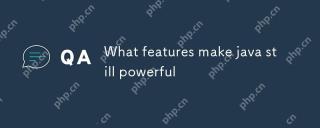
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
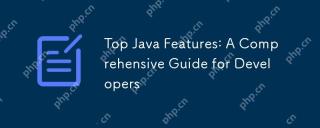
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
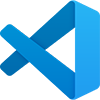
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
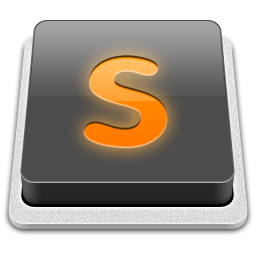
SublimeText3 Mac version
God-level code editing software (SublimeText3)
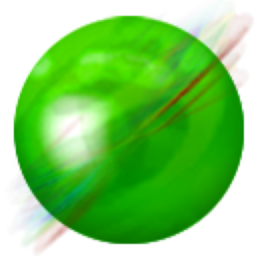
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
