


File Access Denied: Another Process Is Using the File
Problem:
Attempting to modify or delete a file results in an IOException
with the error message "The process cannot access the file because it is being used by another process."
Root Cause:
This error indicates that another application or process holds an exclusive lock on the target file, preventing your process from accessing it. This lock can be due to the file being open in read or write mode by another program, or even by another part of your own application.
Troubleshooting Steps:
Within Your Application:
-
Proper File Handling: Double-check that your code correctly opens and closes the file. Utilize
using
statements (orIDisposable
) to guarantee automatic resource release. - Concurrent Access: If multiple threads in your application access the file simultaneously, implement thread synchronization mechanisms (locks, mutexes) or a retry mechanism to avoid conflicts.
- Process Monitoring: If the problem persists, use tools like Process Explorer to identify processes currently accessing the file.
External Processes:
- File Permissions: Verify that your application has the necessary permissions to access and modify the file. Check file ownership and access rights.
- Retry Mechanism: Implement a retry loop with exponential backoff to allow other processes time to release the file.
-
File Sharing: If concurrent access is required, use the
FileShare
enumeration to allow multiple processes to access the file concurrently, but be mindful of potential data corruption from conflicting operations. - Force Unlock (Caution!): Forcibly unlocking a file held by another process is extremely risky and can lead to data loss or corruption. Avoid this unless absolutely necessary and you fully understand the implications.
Best Practices for Prevention:
-
using
Statements: Always useusing
statements for file operations to ensure automatic closure and release of resources. - Centralized File Access: Create dedicated functions or classes to manage file access, ensuring consistent and controlled operations.
-
File Existence Check: Use
File.Exists()
to verify the file's existence before attempting any operations. - Robust Error Handling: Implement comprehensive error handling to gracefully manage file access exceptions.
- Consider Locking Mechanisms: For critical file operations, explore more robust locking mechanisms beyond simple file locking to handle concurrency safely.
The above is the detailed content of Why Can't My Process Access This File? It Says Another Process Is Using It.. For more information, please follow other related articles on the PHP Chinese website!
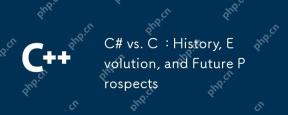
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
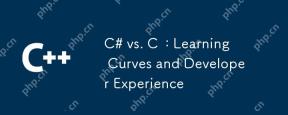
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
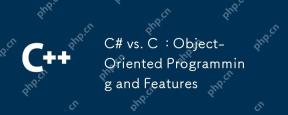
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
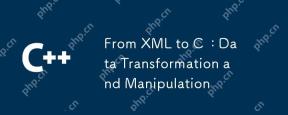
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
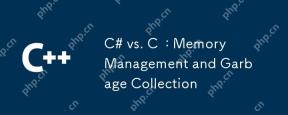
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
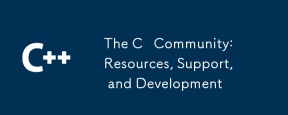
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
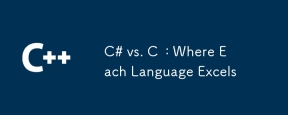
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
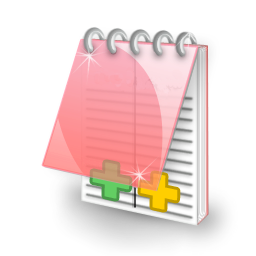
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
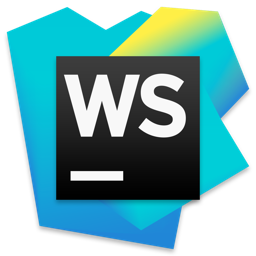
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.