Gracefully Handling Asynchronous Task Cancellation in WinRT
Windows 8 WinRT's asynchronous task management, while powerful, presents challenges when canceling tasks. A common problem is the CancelNotification
method firing, yet the task continues execution, leaving the task status incorrectly marked as "completed" instead of "canceled."
The Solution: Leveraging CancellationToken
Effectively
The key to resolving this lies in correctly implementing the CancellationToken
and adhering to the Task-Based Asynchronous Pattern (TAP) guidelines. This involves passing a CancellationToken
to every cancellable method and regularly checking its status within those methods.
Revised Code Example:
This improved code snippet demonstrates the proper use of CancellationToken
:
private async Task TryTask() { CancellationTokenSource source = new CancellationTokenSource(); source.CancelAfter(TimeSpan.FromSeconds(1)); Task<int> task = Task.Run(() => slowFunc(1, 2, source.Token), source.Token); try { // A canceled task will throw an exception when awaited. await task; } catch (OperationCanceledException) { // Handle cancellation gracefully. } } private int slowFunc(int a, int b, CancellationToken cancellationToken) { for (int i = 0; i < 1000000; i++) { cancellationToken.ThrowIfCancellationRequested(); // Check for cancellation // ... your long-running operation ... } return a + b; }
This revised slowFunc
method incorporates a cancellationToken.ThrowIfCancellationRequested()
check within its loop. If cancellation is requested, an OperationCanceledException
is thrown, effectively stopping the task and correctly setting its status to "canceled." The TryTask
method now includes a try-catch
block to handle this exception.
Outcome:
This approach ensures that tasks are completely halted upon cancellation, providing accurate task status reporting and preventing unintended background execution. Proper exception handling further enhances robustness.
The above is the detailed content of How Can I Properly Cancel Asynchronous Tasks in Windows 8 WinRT?. For more information, please follow other related articles on the PHP Chinese website!
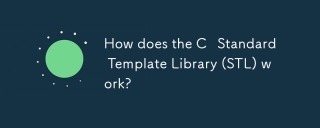
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
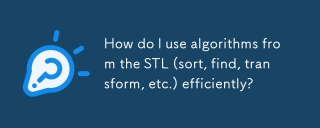
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
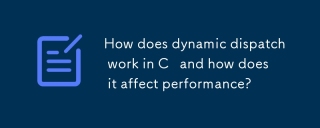
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
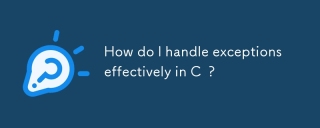
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
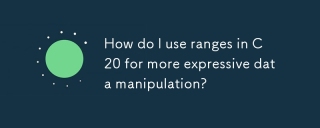
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
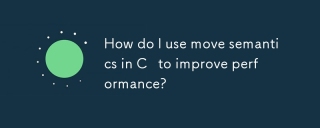
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
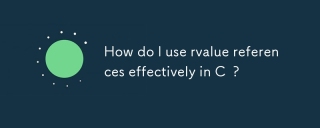
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
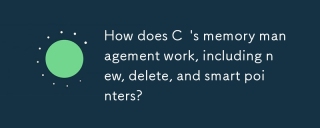
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
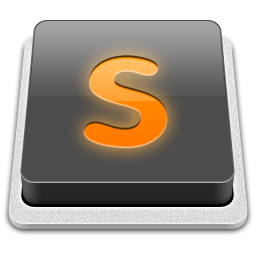
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
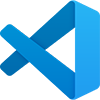
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
