Embedding Images in Email Bodies with C
Directly embedding images within the body of an email using C# requires a specific approach, as standard email attachments only display as placeholders. This refined C# code demonstrates how to correctly embed images:
MailMessage mailWithImg = GetMailWithImg(); MySMTPClient.Send(mailWithImg); // Ensure MySMTPClient is properly configured private MailMessage GetMailWithImg() { MailMessage mail = new MailMessage(); mail.IsBodyHtml = true; mail.AlternateViews.Add(GetEmbeddedImage("c:/image.png")); // Replace with your image path mail.From = new MailAddress("yourAddress@yourDomain"); // Replace with your email address mail.To.Add("recipient@hisDomain"); // Replace with recipient's email address mail.Subject = "yourSubject"; // Replace with your email subject return mail; } private AlternateView GetEmbeddedImage(String filePath) { LinkedResource res = new LinkedResource(filePath); res.ContentId = Guid.NewGuid().ToString(); string htmlBody = $"<img src=\"cid:{res.ContentId}\" / alt="How Can I Embed Images Directly into the Body of an Email Using C#?" >"; // Note the escaped quotes AlternateView alternateView = AlternateView.CreateAlternateViewFromString(htmlBody, null, MediaTypeNames.Text.Html); alternateView.LinkedResources.Add(res); return alternateView; }
This code leverages the AlternateView
and LinkedResource
classes. LinkedResource
associates the image file with a unique ContentId
. The HTML within AlternateView
then references this ContentId
, ensuring the image is displayed inline. This method prevents the common "red x" placeholder often seen with attached images. Remember to replace placeholder values with your actual data.
The above is the detailed content of How Can I Embed Images Directly into the Body of an Email Using C#?. For more information, please follow other related articles on the PHP Chinese website!
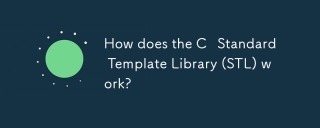
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
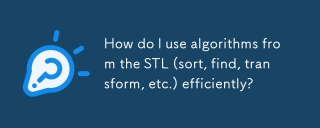
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
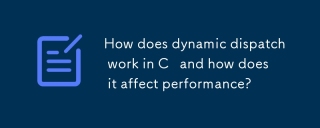
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
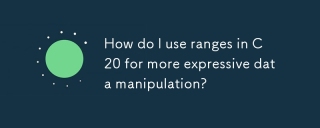
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
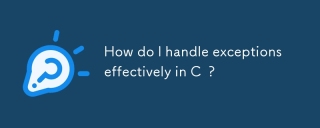
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
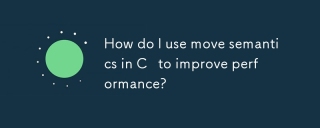
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
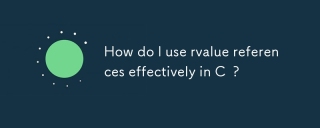
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
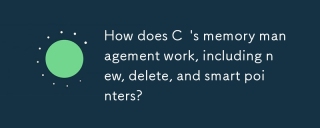
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
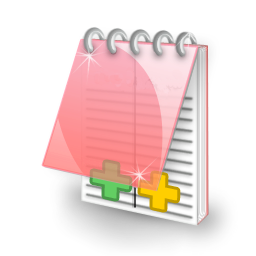
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
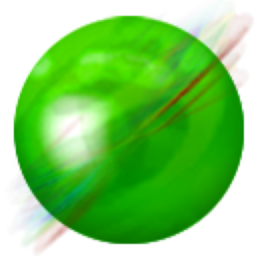
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
