


Handling Accents and Case in C# String Comparisons
C# string comparisons can be complicated by accented characters and case sensitivity. This article demonstrates how to perform case-insensitive comparisons while also ignoring diacritical marks (accents).
Accented characters are often treated differently than their non-accented counterparts, leading to inaccurate comparisons. To address this, we can preprocess strings to remove accents before comparison. The following RemoveDiacritics
function uses Unicode normalization to achieve this:
static string RemoveDiacritics(string text) { string formD = text.Normalize(NormalizationForm.FormD); StringBuilder sb = new StringBuilder(); foreach (char ch in formD) { UnicodeCategory uc = CharUnicodeInfo.GetUnicodeCategory(ch); if (uc != UnicodeCategory.NonSpacingMark) { sb.Append(ch); } } return sb.ToString().Normalize(NormalizationForm.FormC); }
This function normalizes the input string and then iterates through its characters, removing any non-spacing marks (accents). The result is a string with accents removed.
Now, to perform a case-insensitive comparison ignoring accents, simply apply this function to your strings before using Equals
:
string s1 = "résumé"; string s2 = "resume"; bool areEqual = RemoveDiacritics(s1).Equals(RemoveDiacritics(s2), StringComparison.OrdinalIgnoreCase); // true
Using StringComparison.OrdinalIgnoreCase
provides a culture-insensitive case-insensitive comparison, ensuring consistent results across different systems. This approach ensures that strings with and without accents are treated as equivalent when performing case-insensitive comparisons, improving the accuracy and robustness of your application logic.
The above is the detailed content of How Can I Perform Case-Insensitive String Comparisons in C# While Ignoring Accents?. For more information, please follow other related articles on the PHP Chinese website!
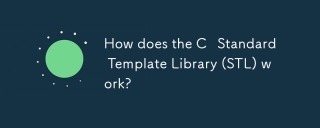
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
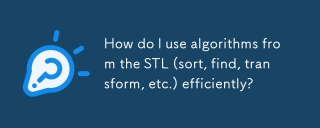
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
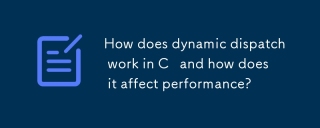
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
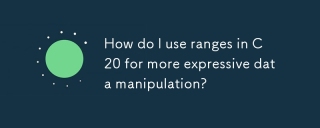
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
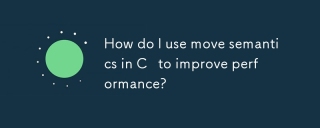
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
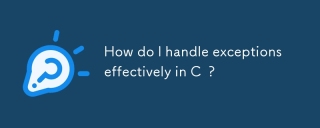
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
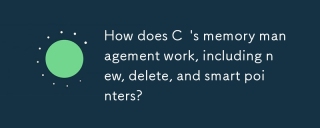
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
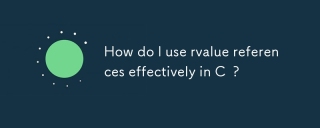
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
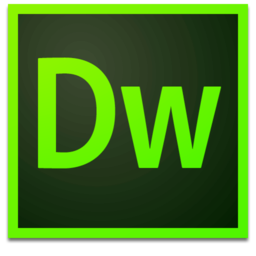
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
