Simple and powerful status processing
export class State<IState extends Record<string, unknown>> { private data = new Map<keyof IState, IState[keyof IState]>(); private subscribers = new Map<string, ((...args: any[]) => void)[]>(); set(name: keyof IState, value: IState[keyof IState]): void { this.data.set(name, value); this.publish(`change:${String(name)}`, value); } get(name: keyof IState): IState[keyof IState] | undefined { return this.data.get(name); } has(name: keyof IState): boolean { return this.data.has(name); } clear(): void { this.data.clear(); this.publish('clear'); } publish(name: string, ...args: any[]): void { this.subscribers.get(name)?.forEach(fn => fn(...args)); } subscribe(name: string, fn: (...args: any[]) => void): void { this.subscribers.has(name) ? this.subscribers.get(name)!.push(fn) : this.subscribers.set(name, [fn]); } unsubscribe(name: string, fn: (...args: any[]) => void): void { if (this.subscribers.has(name)) { const idx = this.subscribers.get(name)!.indexOf(fn); if (idx > -1) this.subscribers.get(name)!.splice(idx, 1); } } unsubscribeAll(name: string): void { this.subscribers.delete(name); } }
Data Binding: True Responsiveness
React integration example
function UserProfile() { const state = new State(); const [userData, setUserData] = useState({ name: state.get('name') || '', age: state.get('age') || 0 }); // 通过状态变化实现自动响应 state.subscribe('change:name', (newName) => { setUserData(prev => ({ ...prev, name: newName })); }); return ( <div> <input type="text" onChange={e => state.set('name', e.target.value)} /> </div> ); }
Why it’s better than reactive libraries
- Zero external dependencies
- Minimized package size
- Native JavaScript performance
- Simple and intuitive API
- Built-in change tracking
Key responsive principles
- Status changes trigger updates
- Automatically notify subscribers
- No need for complex stream processing
- Direct, predictable data flow
Conclusion
Reactive is not dependent on libraries. It's about understanding how data flows and changes. This implementation proves that powerful state management is native to JavaScript.
The above is the detailed content of Reactive State Management Without Libraries. For more information, please follow other related articles on the PHP Chinese website!
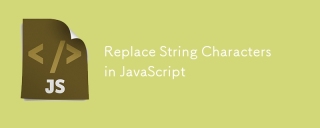
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
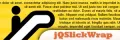
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
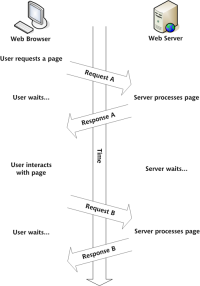
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
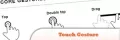
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
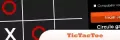
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
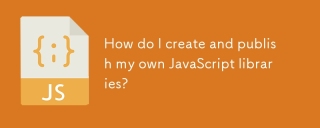
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
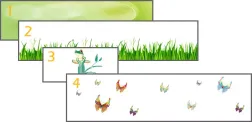
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
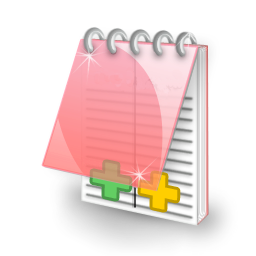
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
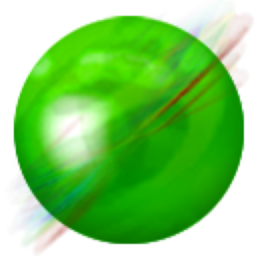
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
