


Integrating PowerShell scripts into C# applications provides powerful automation capabilities. However, handling command-line arguments containing spaces can be tricky. This article demonstrates a robust method for executing PowerShell scripts from C#, reliably passing arguments with embedded spaces.
The common approach of directly passing arguments often fails when spaces are present. The solution lies in using the PowerShell Command
and CommandParameter
objects to properly manage argument construction.
Here's a refined approach to execute a PowerShell script from C# with arguments, even those containing spaces:
-
Instantiate a PowerShell Runspace: Create a
Runspace
to manage the PowerShell execution environment. -
Construct the Command: Create a
Command
object specifying the path to your PowerShell script. -
Add Parameters: Use
CommandParameter
objects to add arguments. This correctly handles spaces and special characters. EachCommandParameter
takes the argument name and its value. -
Add to Pipeline: Add the
Command
object to aPipeline
. - Invoke the Pipeline: Execute the pipeline, initiating the PowerShell script execution.
Here's an improved code example reflecting these steps:
RunspaceConfiguration runspaceConfiguration = RunspaceConfiguration.Create(); Runspace runspace = RunspaceFactory.CreateRunspace(runspaceConfiguration); runspace.Open(); Pipeline pipeline = runspace.CreatePipeline(); // Path to your PowerShell script string scriptFile = "path/to/your/script.ps1"; // Create the Command object Command myCommand = new Command(scriptFile); // Add parameters with spaces handled correctly myCommand.Parameters.Add(new CommandParameter("key1", "value with spaces")); myCommand.Parameters.Add(new CommandParameter("key2", "another value")); // Add the command to the pipeline pipeline.Commands.Add(myCommand); // Invoke the pipeline and handle the results Collection<PSObject> results = pipeline.Invoke(); // Process the results as needed foreach (PSObject result in results) { // ... handle each result ... } runspace.Close();
This approach ensures that command-line arguments, regardless of whether they contain spaces, are correctly passed to your PowerShell script, enabling seamless integration between C# and PowerShell. Remember to replace "path/to/your/script.ps1"
with the actual path to your script.
The above is the detailed content of How to Pass Command-Line Arguments with Spaces to PowerShell Scripts from C#?. For more information, please follow other related articles on the PHP Chinese website!
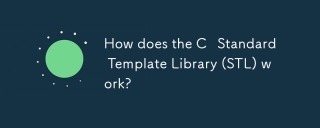
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
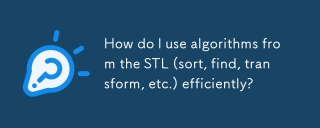
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
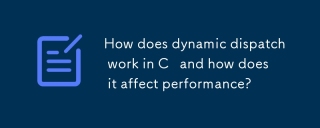
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
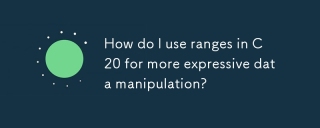
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
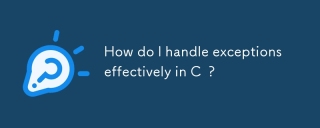
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
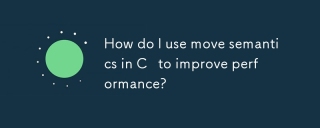
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
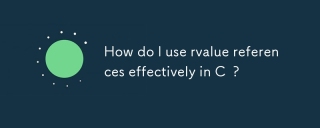
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
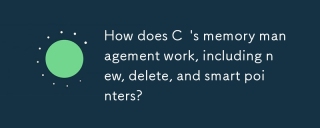
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
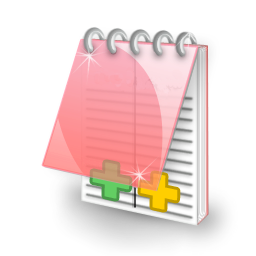
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
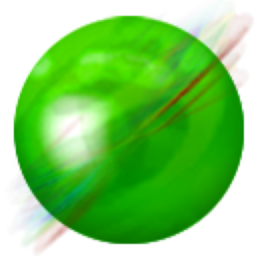
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
