


Build AI Agent Connected to Unlimited APIs with Vercels AI SDK & Picas OneTool
Unlock the power of seamless API interaction in your software projects! This tutorial guides you through building an AI agent that effortlessly connects to multiple APIs using Express, Vercel's AI SDK, and Pica's AI infrastructure.
Prerequisites
Before you begin, ensure you have Node.js and npm installed. You'll also need an OpenAI API key and a Pica Secret Key. Create a new project and install the required packages:
npm install express @ai-sdk/openai ai @picahq/ai dotenv
Next, create a .env
file in your project's root directory and add your API keys:
<code>PICA_SECRET_KEY=your-pica-secret-key OPENAI_API_KEY=your-openai-api-key PORT=3000</code>
Remember to replace the placeholder keys with your actual keys.
Step 1: Setting Up the Express Server
Create server.js
and build a basic Express server to handle AI interactions:
import express from "express"; import { openai } from "@ai-sdk/openai"; import { generateText } from "ai"; import { Pica } from "@picahq/ai"; import * as dotenv from "dotenv"; dotenv.config(); const app = express(); const port = process.env.PORT || 3000; app.use(express.json()); app.post("/api/ai", async (req, res) => { try { const { message } = req.body; const pica = new Pica(process.env.PICA_SECRET_KEY); const systemPrompt = await pica.generateSystemPrompt(); const { text } = await generateText({ model: openai("gpt-4o"), system: systemPrompt, tools: { ...pica.oneTool }, prompt: message, maxSteps: 5, }); res.setHeader("Content-Type", "application/json"); res.status(200).json({ text }); } catch (error) { console.error("Error:", error); res.status(500).json({ error: "Server error" }); } }); app.listen(port, () => { console.log(`Server listening on port ${port}`); }); export default app;
Step 2: API Testing
Start your server. You can test the /api/ai
endpoint using curl
or Postman:
curl --location 'http://localhost:3000/api/ai' \ --header 'Content-Type: application/json' \ --data '{ "message": "What connections do I have access to?" }'
Expect a response indicating no connections are available until you configure them in the Pica dashboard.
Understanding the Code
The code utilizes several key components:
- Express: Manages the server and routing.
-
@ai-sdk/openai
andai
: Handle OpenAI API calls. -
@picahq/ai
: Provides access to Pica's infrastructure. -
dotenv
: Securely loads API keys from the.env
file. - The
/api/ai
endpoint initializes Pica, generates a system prompt, processes the user's message, and returns the AI's response.
Step 3: Next Steps and Deployment
- Enhancements: Implement authentication and rate limiting for production environments.
- Expansion: Leverage Pica's additional tools to connect to a broader range of APIs and data sources.
- Deployment: Deploy your server to a platform like Vercel or AWS for wider accessibility.
Conclusion
You've successfully built a foundation for an AI agent that can interact with numerous APIs. Expand upon this to automate tasks, process complex requests, and seamlessly integrate with other services. Feel free to share your questions or connect on Twitter! Happy coding!
The above is the detailed content of Build AI Agent Connected to Unlimited APIs with Vercels AI SDK & Picas OneTool. For more information, please follow other related articles on the PHP Chinese website!
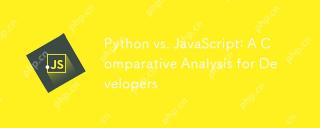
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
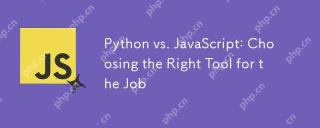
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
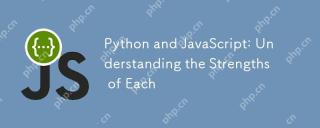
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
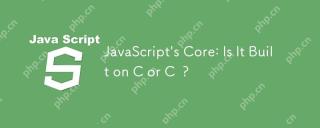
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
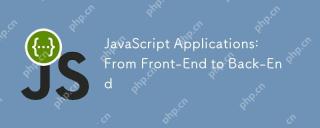
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
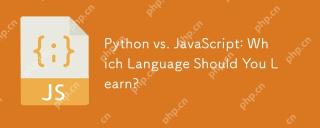
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
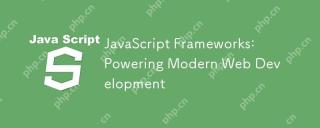
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
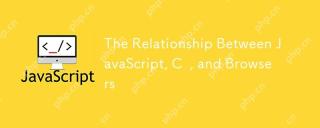
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
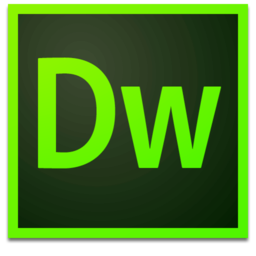
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
