WPF Runtime Image Loading: A Comprehensive Guide
When developing a WPF application, you may need to dynamically load images at runtime. While this seems simple, there are some subtleties that need to be taken care of to display the image correctly.
Load image from URI
A common way to load images in WPF is to use the BitmapImage
class. It supports loading images from URI, allowing you to specify remote and local image sources. For example, to load an image from a remote URL, you can use the following code:
var uri = new Uri("http://..."); var bitmap = new BitmapImage(uri);
Load image from local file path
Alternatively, if your image is stored locally, you can use the file://
URI by constructing it from a file path:
var path = Path.Combine(Environment.CurrentDirectory, "Bilder", "sas.png"); var uri = new Uri(path);
Load image as assembly resource
For images embedded as assembly resources, you should use the Pack URI scheme:
var uri = new Uri("pack://application:,,,/Bilder/sas.png");
Please make sure the image file has a "Resource" build action in Visual Studio.
Assign BitmapImage to Image control
After creating the BitmapImage
, you need to assign it to the Source property of the Image control. This will display the image in the WPF window:
image1.Source = bitmap;
Troubleshooting: Resolving red squiggly lines in code
If a red squiggly line appears under your code, make sure you include the following using
statement to import the necessary WPF namespace:
using System.Windows; using System.Windows.Controls; using System.Windows.Media.Imaging;
Also, please verify that the referenced image file exists in the correct path or assembly location.
The above is the detailed content of How Can I Efficiently Load Images at Runtime in My WPF Application?. For more information, please follow other related articles on the PHP Chinese website!
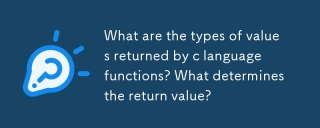
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
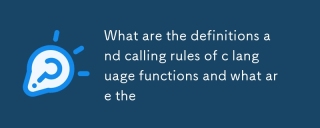
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
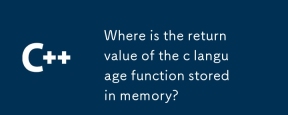
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
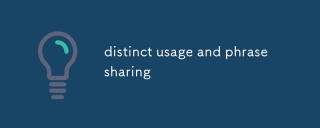
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
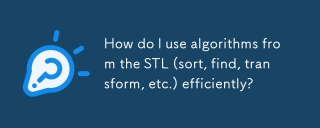
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
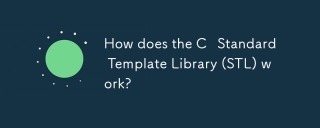
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
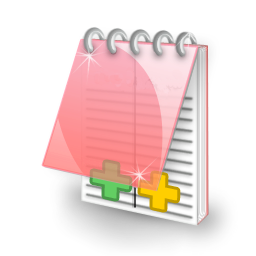
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
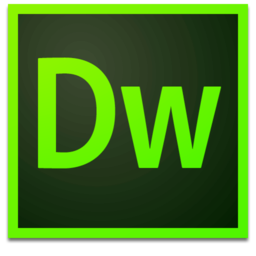
Dreamweaver Mac version
Visual web development tools
