React.js has become one of the most popular JavaScript libraries for building user interfaces, especially single-page applications. Its component-based architecture and efficient rendering make it a developer favorite. Whether you are a beginner or an experienced developer, there are always new tips and tricks to learn to make your development process more efficient and your code more elegant. Listed below are 11 useful React.js tips every developer should know:
-
Use function components with Hooks
With the introduction of React Hooks, functional components are more powerful than ever. Hooks allow you to use state and other React features without writing classes. This makes your code cleaner and easier to understand.
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default Counter;
-
Use React.memo memory component
To optimize performance, you can use React.memo to memorize function components. By comparing props, only re-render when props change, thus avoiding unnecessary re-rendering.
import React from 'react'; const MemoizedComponent = React.memo(({ value }) => { console.log('Rendering...'); return <div>{value}</div>; }); export default MemoizedComponent;
-
Use useEffect to deal with side effects
useEffect hook is used to perform side effects in function components. It can be used for data retrieval, subscription or manual changes to the DOM.
import React, { useEffect, useState } from 'react'; const DataFetcher = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => setData(data)); }, []); return ( <div> {data ? <pre class="brush:php;toolbar:false">{JSON.stringify(data, null, 2)}: 'Loading...'} ); }; export default DataFetcher;
-
Customized Hooks to implement reusable logic
Custom Hooks allow you to extract and reuse logic in different components. This promotes code reusability and keeps components clean.
import { useState, useEffect } from 'react'; const useFetch = (url) => { const [data, setData] = useState(null); const [loading, setLoading] = useState(true); useEffect(() => { fetch(url) .then(response => response.json()) .then(data => { setData(data); setLoading(false); }); }, [url]); return { data, loading }; }; export default useFetch;
-
Conditional rendering using short-circuit evaluation
Use short-circuit evaluation to simplify conditional rendering. This makes your JSX more concise and readable.
const ConditionalRender = ({ isLoggedIn }) => { return ( <div> {isLoggedIn && <p>Welcome back!</p>} {!isLoggedIn && <p>Please log in.</p>} </div> ); }; export default ConditionalRender;
-
Code splitting using React.lazy
Code splitting helps reduce the initial load time of your application by splitting the code into multiple packages that can be loaded on demand.
import React, { Suspense, lazy } from 'react'; const LazyComponent = lazy(() => import('./LazyComponent')); const App = () => ( <div> <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> </div> ); export default App;
-
Use error boundaries for elegant error handling
Error boundaries can catch JavaScript errors anywhere in its child component tree, log those errors, and display an alternate UI instead of a crashed component tree.
import React from 'react'; class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } componentDidCatch(error, errorInfo) { console.error(error, errorInfo); } render() { if (this.state.hasError) { return <h1 id="Something-went-wrong">Something went wrong.</h1>; } return this.props.children; } } export default ErrorBoundary;
-
Group elements using React.Fragment
React.Fragment allows you to group a list of child elements without adding extra nodes to the DOM. This is especially useful when you need to return multiple elements from a component.
const List = () => { return ( <React.Fragment> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </React.Fragment> ); }; export default List;
-
Higher Order Components (HOC) for code reuse
Higher-order components (HOC) are a pattern in React for reusing component logic. A HOC is a function that takes a component and returns a new component.
const withLogger = (WrappedComponent) => { return class extends React.Component { componentDidMount() { console.log('Component mounted'); } render() { return <WrappedComponent {...this.props} />; } }; }; export default withLogger;
-
Use React.Context for global state management
React.Context provides a way to pass data through the component tree without manually passing props at each layer. This is useful for managing global state.
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default Counter;
-
Use React.PureComponent to optimize performance
React.PureComponent is similar to React.Component, but it uses shallow props and state comparison to implement shouldComponentUpdate. This can improve performance by reducing unnecessary re-rendering.
import React from 'react'; const MemoizedComponent = React.memo(({ value }) => { console.log('Rendering...'); return <div>{value}</div>; }); export default MemoizedComponent;
Conclusion
React.js is a powerful library that provides a wide range of features and best practices to help developers build efficient and easy-to-maintain applications. By leveraging these 11 tips, you can streamline your development process, improve performance, and write cleaner, more reusable code. Whether you're just getting started with React or looking to improve your skills, these tips will help you become a more proficient React developer.
Happy coding!
The above is the detailed content of Useful React.js Hacks Every Developer Should Know. For more information, please follow other related articles on the PHP Chinese website!
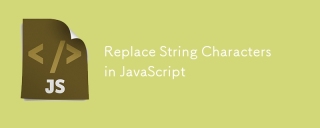
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
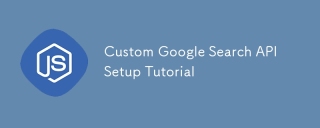
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
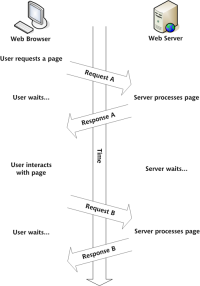
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
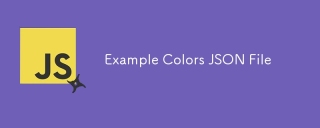
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
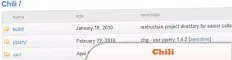
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
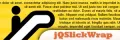
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
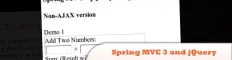
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
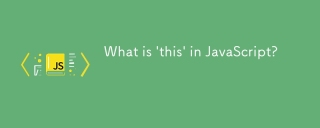
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
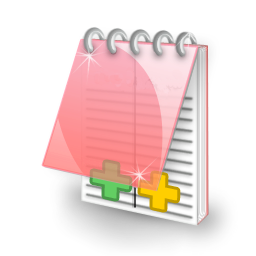
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
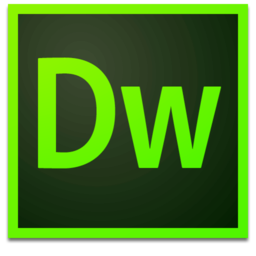
Dreamweaver Mac version
Visual web development tools
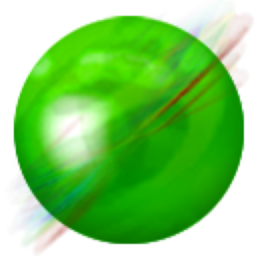
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
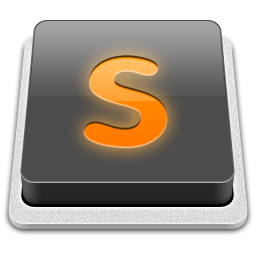
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
