JSON: A Versatile Data Format for Server-Client Communication
Efficiently sending data from a server to a client for display on a webpage or other visual interface often involves using JSON (JavaScript Object Notation). Let's explore JSON's capabilities and compare it to alternative approaches.
Why JSON? The Advantages of Text-Based Formats
Without JSON (or a similar text-based format), updating data structures between different versions of your application can be problematic. Older versions might not understand new data fields, and newer versions might struggle with missing data from older versions. JSON elegantly solves this: older versions simply ignore unknown fields, and newer versions can use default values for missing ones. While binary formats can achieve this, they require maintaining both old and new read/write functions, along with version numbers embedded in the file—a significant overhead compared to JSON's simplicity. The inherent readability of text-based formats like JSON also aids in debugging and development.
Understanding JSON (JavaScript Object Notation)
JSON is a standard text-based format for structured data, inspired by JavaScript object syntax. Its widespread adoption transcends JavaScript; many programming languages provide built-in or readily available libraries for parsing and generating JSON. This article focuses on its use in Java.
Parsing JSON in Java: A Comparison of Three Libraries
Several Java libraries excel at parsing JSON data. This article examines three popular choices: Jackson, Gson, and org.json.
1. Jackson (FasterXML)
Jackson is a high-performance JSON processor. To use it, add the following dependency to your pom.xml
:
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.15.2</version> </dependency>
Example Code:
This code snippet demonstrates converting JSON to a Java object using Jackson:
import com.fasterxml.jackson.databind.ObjectMapper; public class JsonParserExample { public static void main(String[] args) throws Exception { String json = "{\"name\": \"John\", \"age\": 30}"; ObjectMapper objectMapper = new ObjectMapper(); Person person = objectMapper.readValue(json, Person.class); System.out.println("Name: " + person.getName()); System.out.println("Age: " + person.getAge()); } } class Person { private String name; private int age; // Getters and setters public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
Jackson's readValue
method parses the JSON string and maps it to the Person
class, leveraging the class's fields and getter/setter methods.
2. Gson (Google)
Gson, developed by Google, offers another robust solution. Add this dependency to your pom.xml
:
<dependency> <groupId>com.google.gson</groupId> <artifactId>gson</artifactId> <version>2.10.1</version> </dependency>
Example Code:
Gson's fromJson
method performs a similar conversion:
import com.google.gson.Gson; public class JsonParserExample { public static void main(String[] args) { String json = "{\"name\": \"John\", \"age\": 30}"; Gson gson = new Gson(); Person person = gson.fromJson(json, Person.class); System.out.println("Name: " + person.getName()); System.out.println("Age: " + person.getAge()); } } // Person class remains the same as in the Jackson example
3. org.json
The org.json
library provides a straightforward approach. You'll need to add the appropriate dependency (consult the org.json documentation for the latest version).
Example Code:
import org.json.JSONObject; public class JsonParserExample { public static void main(String[] args) { String json = "{\"name\": \"John\", \"age\": 30}"; JSONObject jsonObject = new JSONObject(json); String name = jsonObject.getString("name"); int age = jsonObject.getInt("age"); System.out.println("Name: " + name); System.out.println("Age: " + age); } }
Here, org.json
directly parses the JSON into a JSONObject
, allowing access to its fields using methods like getString
and getInt
.
Choosing the Right Library
Jackson is often favored for enterprise projects due to its performance and extensive features. Gson's simplicity makes it a good choice for smaller projects. org.json
offers a basic but functional alternative. The best library depends on your project's specific needs and preferences. Consider factors such as performance requirements, ease of use, and the availability of additional features. Please share your experiences with other JSON libraries in the comments below!
The above is the detailed content of How can one parse JSON text in a Java application?. For more information, please follow other related articles on the PHP Chinese website!
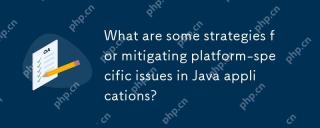
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
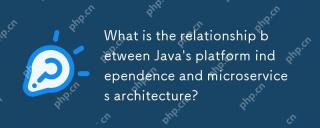
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
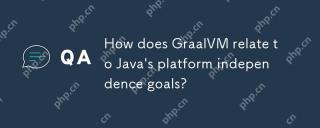
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.
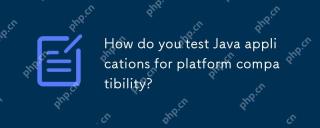
ToeffectivelytestJavaapplicationsforplatformcompatibility,followthesesteps:1)SetupautomatedtestingacrossmultipleplatformsusingCItoolslikeJenkinsorGitHubActions.2)ConductmanualtestingonrealhardwaretocatchissuesnotfoundinCIenvironments.3)Checkcross-pla
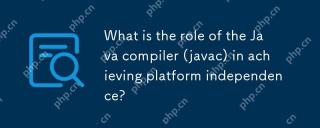
The Java compiler realizes Java's platform independence by converting source code into platform-independent bytecode, allowing Java programs to run on any operating system with JVM installed.
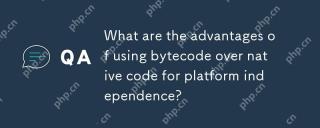
Bytecodeachievesplatformindependencebybeingexecutedbyavirtualmachine(VM),allowingcodetorunonanyplatformwiththeappropriateVM.Forexample,JavabytecodecanrunonanydevicewithaJVM,enabling"writeonce,runanywhere"functionality.Whilebytecodeoffersenh

Java cannot achieve 100% platform independence, but its platform independence is implemented through JVM and bytecode to ensure that the code runs on different platforms. Specific implementations include: 1. Compilation into bytecode; 2. Interpretation and execution of JVM; 3. Consistency of the standard library. However, JVM implementation differences, operating system and hardware differences, and compatibility of third-party libraries may affect its platform independence.
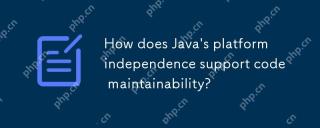
Java realizes platform independence through "write once, run everywhere" and improves code maintainability: 1. High code reuse and reduces duplicate development; 2. Low maintenance cost, only one modification is required; 3. High team collaboration efficiency is high, convenient for knowledge sharing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
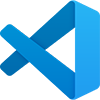
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
