Conquering Extension Method Mocking with Moq: A Practical Guide
Effective unit testing often relies on mocking dependencies. However, mocking extension methods, which add functionality to existing interfaces, presents a unique challenge. Let's explore this problem and its solutions.
Imagine an ISomeInterface
and its extension methods defined in SomeInterfaceExtensions
. A Caller
class uses the AnotherMethod
extension:
public interface ISomeInterface { } public static class SomeInterfaceExtensions { public static void AnotherMethod(this ISomeInterface someInterface) { } } public class Caller { private readonly ISomeInterface someInterface; public Caller(ISomeInterface someInterface) { this.someInterface = someInterface; } public void Main() { someInterface.AnotherMethod(); } }
Testing Caller.Main()
requires mocking ISomeInterface
and verifying AnotherMethod
's call. Directly mocking the extension method with Moq, however, results in an "Invalid setup on a non-member method" error.
The Root of the Problem
Moq's limitation stems from extension methods' nature. They aren't part of the interface's definition; Moq relies on interface members for mocking.
The Wrapper Method: A Robust Solution
A practical solution involves creating a wrapper class that encapsulates the extension method's logic:
public class SomeInterfaceExtensionWrapper { private readonly ISomeInterface wrappedInterface; public SomeInterfaceExtensionWrapper(ISomeInterface wrappedInterface) { this.wrappedInterface = wrappedInterface; } public void AnotherMethod() { wrappedInterface.AnotherMethod(); // Calls the extension method } }
Now, the test can mock the wrapper:
var wrapperMock = new Mock<SomeInterfaceExtensionWrapper>(); wrapperMock.Setup(x => x.AnotherMethod()).Verifiable(); var caller = new Caller(wrapperMock.Object); caller.Main(); wrapperMock.Verify();
Alternative Strategies
While the wrapper approach is effective, it adds complexity. Consider these alternatives:
- Alternative Mocking Frameworks: Explore frameworks supporting extension method mocking.
- Dependency Injection: Inject the extension method's implementation directly as a dependency.
- Architectural Refactoring: Redesign to minimize extension method usage within testable components.
The best approach depends on your project's context and priorities.
The above is the detailed content of How Can I Effectively Mock Extension Methods in Unit Tests Using Moq?. For more information, please follow other related articles on the PHP Chinese website!
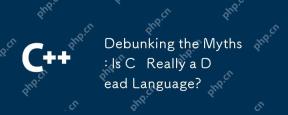
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
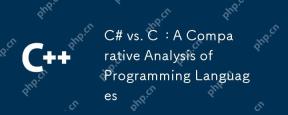
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
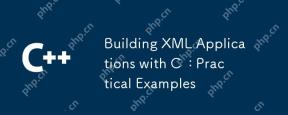
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
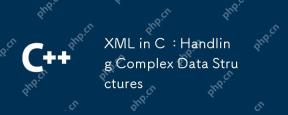
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
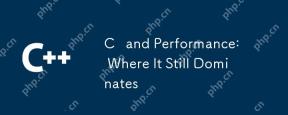
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
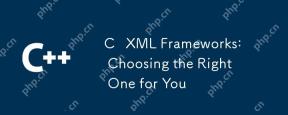
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
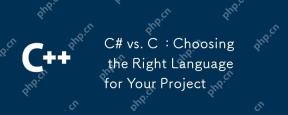
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
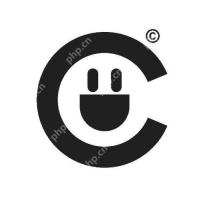
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
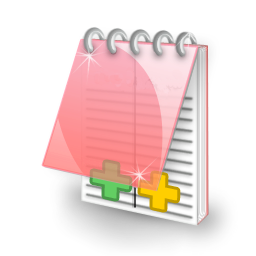
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
