


Using jQuery Ajax to Send an Array of Objects to an MVC Controller
When sending an array of objects to an MVC controller using jQuery's ajax()
method, the controller's parameter might receive a null
value. This article outlines the solution.
Troubleshooting the Null Value
The problem typically stems from incorrect data serialization and content type handling. Here's how to fix it:
-
Setting Content Type and Data Type:
The
ajax()
function requires explicitcontentType
anddataType
settings:contentType: 'application/json; charset=utf-8', dataType: 'json',
-
JSON Serialization:
The array of objects must be serialized into JSON format using
JSON.stringify()
. Structure the data like this:things = JSON.stringify({ things: things });
Illustrative Example
This example demonstrates how to successfully pass an array of objects:
$(document).ready(function() { const things = [ { id: 1, color: 'yellow' }, { id: 2, color: 'blue' }, { id: 3, color: 'red' } ]; const data = JSON.stringify({ things: things }); $.ajax({ contentType: 'application/json; charset=utf-8', dataType: 'json', type: 'POST', url: '/Home/PassThings', data: data, success: function() { $('#result').html('"PassThings()" successfully executed.'); }, error: function(response) { $('#result').html(response.responseText); // Display error details } }); });
Corresponding Controller Method (C# Example):
public void PassThings(List<Thing> things) { // Process the 'things' list here }
Remember to replace /Home/PassThings
with your actual controller and action. Using error
instead of failure
provides more informative error handling. This revised approach ensures proper data transmission and prevents the null
value issue.
The above is the detailed content of How to Properly Pass an Array of Objects to an MVC Controller Using jQuery Ajax?. For more information, please follow other related articles on the PHP Chinese website!
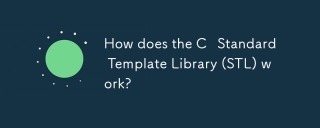
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
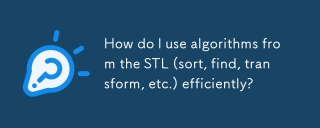
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
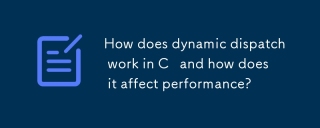
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
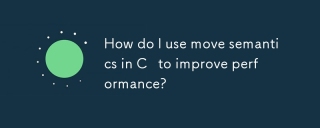
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
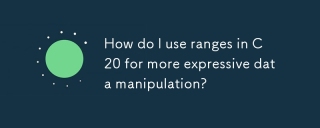
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
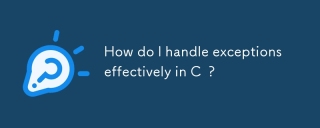
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
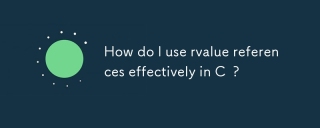
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
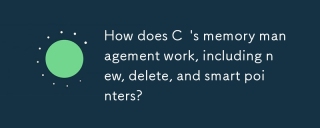
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
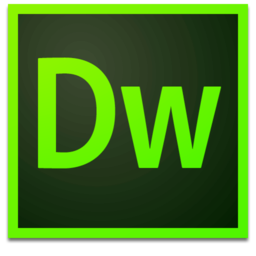
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
