Tracking Down the Last Run Time of a Terminated .NET Process
The .NET Process
class offers insights into currently active processes. However, it falls short when trying to determine the last execution time of a process that's already ended.
WMI: The Solution
This challenge is effectively addressed using Windows Management Instrumentation (WMI). WMI allows monitoring process start and stop events. Here's a practical implementation:
using System; using System.Management; public class ProcessMonitor { public static void Main(string[] args) { // Watch for process starts using (var startWatch = new ManagementEventWatcher(new WqlEventQuery("SELECT * FROM Win32_ProcessStartTrace"))) { startWatch.EventArrived += StartWatch_EventArrived; startWatch.Start(); // Watch for process stops using (var stopWatch = new ManagementEventWatcher(new WqlEventQuery("SELECT * FROM Win32_ProcessStopTrace"))) { stopWatch.EventArrived += StopWatch_EventArrived; stopWatch.Start(); Console.WriteLine("Monitoring process activity. Press any key to exit."); Console.ReadKey(); } startWatch.Stop(); } } private static void StopWatch_EventArrived(object sender, EventArrivedEventArgs e) { Console.WriteLine($"Process stopped: {e.NewEvent.Properties["ProcessName"].Value}"); } private static void StartWatch_EventArrived(object sender, EventArrivedEventArgs e) { Console.WriteLine($"Process started: {e.NewEvent.Properties["ProcessName"].Value}"); } }
Essential: Elevated Permissions
To effectively monitor process events, this application requires elevated privileges. Adjust the application manifest accordingly.
How to Use
Run the program. It will continuously monitor process starts and stops, displaying the process name each time. Press any key to end monitoring.
The above is the detailed content of How Can I Determine the Last Execution Time of a Stopped Process in .NET?. For more information, please follow other related articles on the PHP Chinese website!
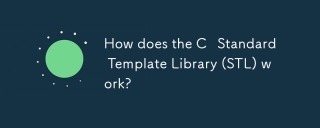
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
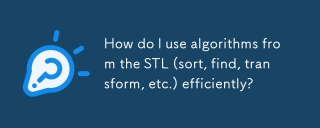
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
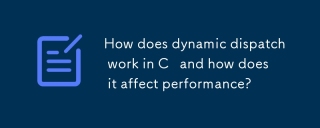
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
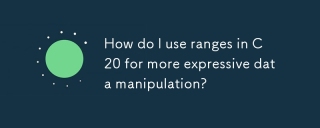
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
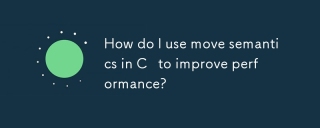
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
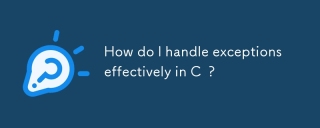
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
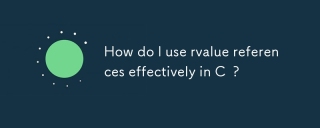
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
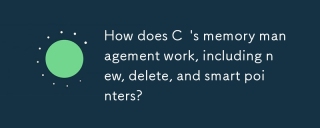
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
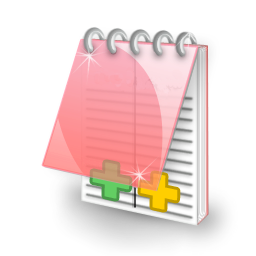
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
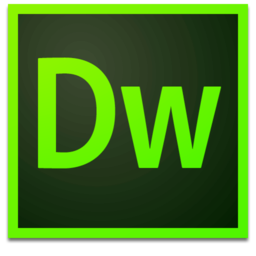
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
