Monitoring Process Activity with .NET and WMI
.NET offers a powerful method for tracking process lifecycle events using Windows Management Instrumentation (WMI). This approach is particularly useful for determining the last execution time of a specific process.
By utilizing the Win32_ProcessTrace
classes, you can effectively monitor process starts and stops. The following code demonstrates how to achieve this:
using System; using System.Management; public class ProcessTracker { public static void Main() { // Initialize event watchers for process start and stop events. ManagementEventWatcher startWatcher = new ManagementEventWatcher( new WqlEventQuery("SELECT * FROM Win32_ProcessStartTrace")); startWatcher.EventArrived += startWatcher_EventArrived; startWatcher.Start(); ManagementEventWatcher stopWatcher = new ManagementEventWatcher( new WqlEventQuery("SELECT * FROM Win32_ProcessStopTrace")); stopWatcher.EventArrived += stopWatcher_EventArrived; stopWatcher.Start(); // Await user input to terminate the application. Console.WriteLine("Press any key to exit."); while (!Console.KeyAvailable) System.Threading.Thread.Sleep(50); // Stop event watchers upon application closure. startWatcher.Stop(); stopWatcher.Stop(); } private static void stopWatcher_EventArrived(object sender, EventArrivedEventArgs e) { // Process stop event handler; logs the process name. Console.WriteLine("Process stopped: {0}", e.NewEvent.Properties["ProcessName"].Value); } private static void startWatcher_EventArrived(object sender, EventArrivedEventArgs e) { // Process start event handler; logs the process name. Console.WriteLine("Process started: {0}", e.NewEvent.Properties["ProcessName"].Value); } }
Remember: This application requires elevated privileges. Modify the application manifest accordingly. This code provides a robust solution for tracking process start and stop events, enabling precise identification of the last execution time for any given process.
The above is the detailed content of How Can I Track Process Start and Stop Events Using .NET and WMI?. For more information, please follow other related articles on the PHP Chinese website!
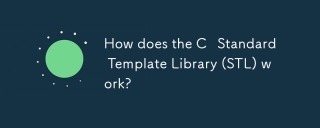
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
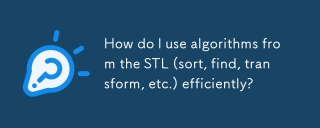
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
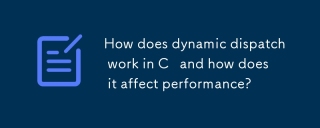
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
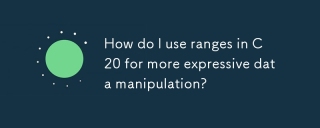
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
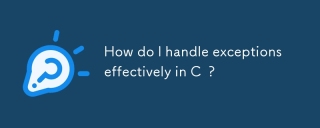
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
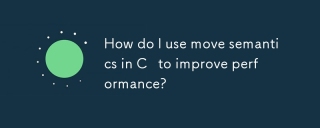
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
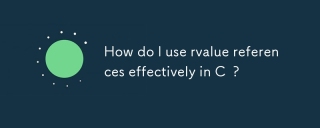
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
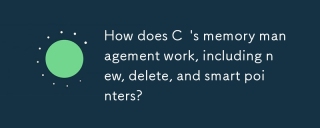
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
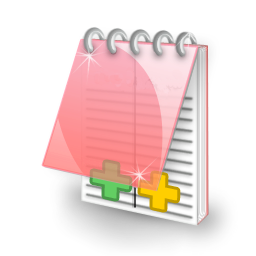
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
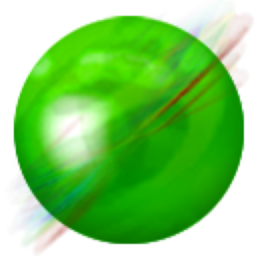
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
