Efficient method to remove array elements in C#
In C#, arrays are implemented as contiguous blocks of memory that store values of the same type. However, unlike other collections such as ArrayLists, arrays have limited flexibility in element manipulation. Here's how to handle element deletion from an array:
Remove elements by value
To remove all instances of a specific value, you can use the .Where() LINQ extension method (if targeting .NET Framework 3.5 or later) or the .NET Framework 2.0's Array.FindAll() method. Consider the following code:
int[] numbers = { 1, 3, 4, 9, 2 }; int numToRemove = 4; // LINQ numbers = numbers.Where(val => val != numToRemove).ToArray(); // 非LINQ numbers = Array.FindAll(numbers, isNotFour).ToArray(); bool isNotFour(int n) { return n != 4; }
Remove the first instance of the value
If you only want to remove the first instance of the value, you can again leverage the LINQ or non-LINQ approach:
int[] numbers = { 1, 3, 4, 9, 2, 4 }; int numToRemove = 4; // LINQ int numIdx = Array.IndexOf(numbers, numToRemove); numbers = numbers.Where((val, idx) => idx != numIdx).ToArray(); // 非LINQ int numIdx = Array.IndexOf(numbers, numToRemove); List<int> tmp = new List<int>(numbers); tmp.RemoveAt(numIdx); numbers = tmp.ToArray();
In summary, while arrays provide efficient element storage and retrieval, they have limited flexibility in element manipulation, making methods such as RemoveAt() unsuitable. Instead, the method outlined above provides an efficient alternative for removing values from an array.
The above is the detailed content of How Can I Efficiently Remove Elements from C# Arrays?. For more information, please follow other related articles on the PHP Chinese website!
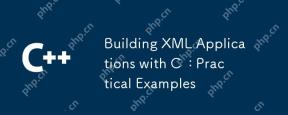
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
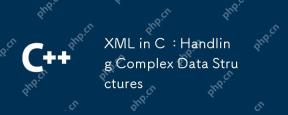
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
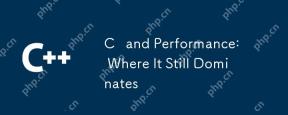
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
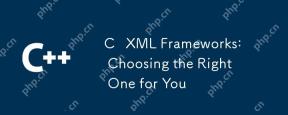
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
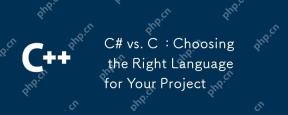
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
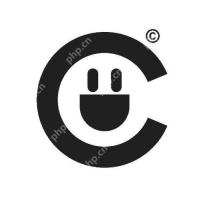
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
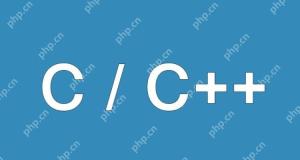
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
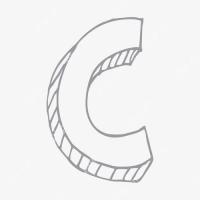
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
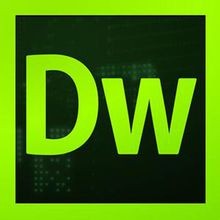
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
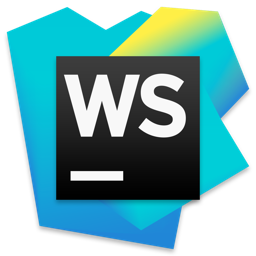
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
