Accurately identifying a string's encoding is paramount for correct data interpretation in C#. While some strings explicitly declare their encoding, many do not. This presents a challenge, but a reliable solution is crucial.
This article details a robust C# method for detecting string encoding. The approach considers several factors, including BOM markers, UTF-8 and UTF-16 patterns, and explicit encoding declarations within the source file.
C# Encoding Detection
The following code provides a comprehensive approach to detect the encoding of a string:
public Encoding detectTextEncoding(string filename, out String text, int taster = 1000) { // Attempts to identify UTF-7, UTF-8/16/32 encodings. // ... (Implementation details omitted for brevity) ... // Heuristic check for UTF-8 without a BOM. // ... (Implementation details omitted for brevity) ... // Heuristic check for UTF-16 without a BOM. // ... (Implementation details omitted for brevity) ... // Searches for "charset=xyz" or "encoding=xyz" within the file. // ... (Implementation details omitted for brevity) ... // Default fallback encoding. text = Encoding.Default.GetString(b); // Assuming 'b' is a byte array representing the file content. return Encoding.Default; }
Method Usage
The detectTextEncoding
method takes the filename and an optional taster
parameter (defaulting to 1000 bytes) to control the amount of data examined for encoding detection. It returns the detected encoding and assigns the decoded string to the text
output parameter.
Accuracy and Limitations
While this method strives for high accuracy, no encoding detection method is completely foolproof, particularly with non-Unicode encodings. The approach employs multiple strategies to minimize errors and maximize the likelihood of correct identification.
Conclusion
This multi-faceted approach to string encoding detection in C# offers improved reliability and flexibility. By considering various factors and incorporating fallback mechanisms, it ensures accurate interpretation of string data across diverse scenarios.
The above is the detailed content of How Can I Reliably Determine a String's Encoding in C#?. For more information, please follow other related articles on the PHP Chinese website!
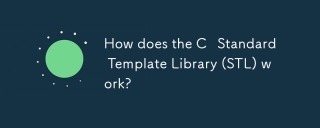
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
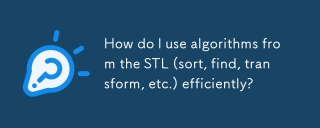
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
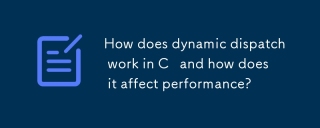
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
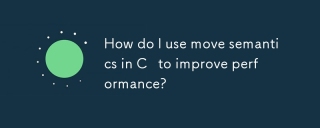
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
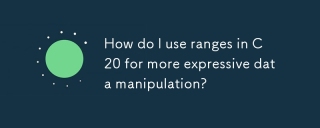
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
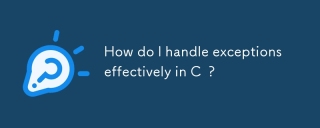
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
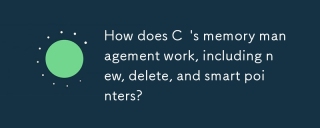
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
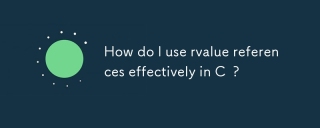
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
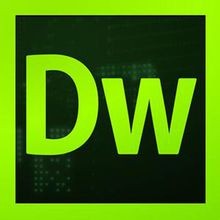
Dreamweaver CS6
Visual web development tools
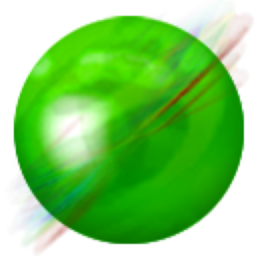
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
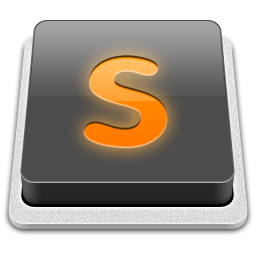
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
