This tutorial demonstrates how to implement Bootstrap pagination in a Laravel Blade application. We'll create an application that populates a database with 10,000 movie records and displays them in a paginated list using Bootstrap's styling and Laravel's Blade templating engine. The large dataset ensures sufficient pages for thorough testing of pagination functionality.
Let's begin!
How to Use Bootstrap Pagination in Laravel Blade
Step 1: Setting up Laravel
First, create a new Laravel project (if you haven't already). Open your terminal and execute:
composer create-project laravel/laravel bootstrap-pagination-demo cd bootstrap-pagination-demo
Step 2: Creating the Movie Model and Migration
Next, generate a Movie
model and its corresponding migration file:
php artisan make:model Movie -m
Modify the migration file (database/migrations/xxxx_xxxx_xx_create_movies_table.php
) to define the 'movies' table structure:
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { public function up(): void { Schema::create('movies', function (Blueprint $table) { $table->id(); $table->string('title'); $table->string('country'); $table->date('release_date'); $table->timestamps(); }); } public function down(): void { Schema::dropIfExists('movies'); } };
Step 3: Running the Migration
Run the migration to create the 'movies' table in your database:
php artisan migrate
Step 4: Creating the Movie Factory
Generate a factory for the Movie
model to create sample data:
php artisan make:factory MovieFactory --model=Movie
Populate the factory file (database/factories/MovieFactory.php
) with the following code:
<?php namespace Database\Factories; use Illuminate\Database\Eloquent\Factories\Factory; /** * @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Movie> */ class MovieFactory extends Factory { /** * Define the model's default state. * * @return array<string, mixed> */ public function definition(): array { return [ 'title' => $this->faker->sentence, 'country' => $this->faker->country, 'release_date' => $this->faker->dateTimeBetween('-40 years', 'now'), ]; } }
Read More
The above is the detailed content of How to Use Bootstrap Pagination in Laravel Blade (Tutorial). For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
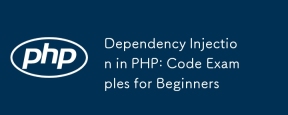
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
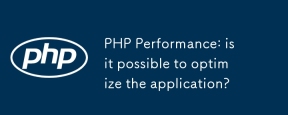
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
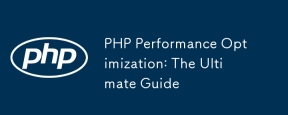
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
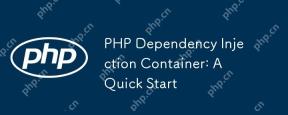
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
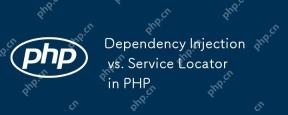
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
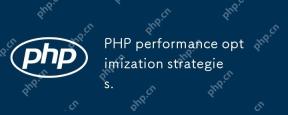
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
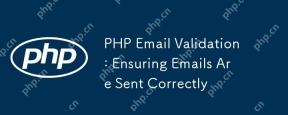
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
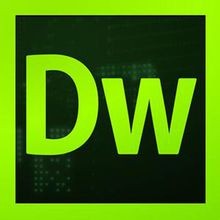
Dreamweaver CS6
Visual web development tools
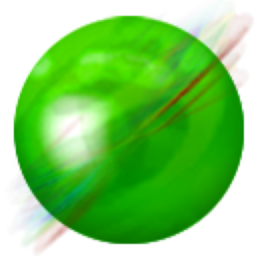
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
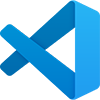
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
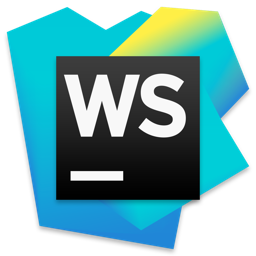
WebStorm Mac version
Useful JavaScript development tools
