


Dynamic web pages, increasingly common in modern web development, present a challenge for traditional web scraping methods. Their asynchronous content loading, driven by JavaScript, often evades standard HTTP requests. Selenium, a powerful web automation tool, offers a solution by mimicking user interactions to access this dynamically generated data. Coupled with proxy IP usage (like that offered by 98IP), it effectively mitigates IP blocking, enhancing crawler efficiency and reliability. This article details how to leverage Selenium and proxy IPs for dynamic web scraping.
I. Selenium Fundamentals and Setup
Selenium simulates user actions (clicks, input, scrolling) within a browser, making it ideal for dynamic content extraction.
1.1 Selenium Installation:
Ensure Selenium is installed in your Python environment. Use pip:
pip install selenium
1.2 WebDriver Installation:
Selenium requires a browser driver (ChromeDriver, GeckoDriver, etc.) compatible with your browser version. Download the appropriate driver and place it in your system's PATH or a specified directory.
II. Core Selenium Operations
Understanding Selenium's basic functions is crucial. This example demonstrates opening a webpage and retrieving its title:
from selenium import webdriver # Set WebDriver path (Chrome example) driver_path = '/path/to/chromedriver' driver = webdriver.Chrome(executable_path=driver_path) # Open target page driver.get('https://example.com') # Get page title title = driver.title print(title) # Close browser driver.quit()
III. Handling Dynamic Content
Dynamic content loads asynchronously via JavaScript. Selenium's waiting mechanisms ensure data integrity.
3.1 Explicit Waits:
Explicit waits pause execution until a specified condition is met, ideal for dynamically loaded content:
from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC # Open page and wait for element driver.get('https://example.com/dynamic-page') try: element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.ID, 'dynamic-content-id')) ) content = element.text print(content) except Exception as e: print(f"Element load failed: {e}") finally: driver.quit()
IV. Utilizing Proxy IPs to Prevent Blocking
Frequent scraping triggers anti-scraping measures, leading to IP blocks. Proxy IPs circumvent this. 98IP Proxy offers numerous IPs for integration with Selenium.
4.1 Configuring Selenium for Proxy Use:
Selenium's proxy settings are configured through browser launch parameters. (Chrome example):
from selenium import webdriver from selenium.webdriver.chrome.options import Options # Configure Chrome options chrome_options = Options() chrome_options.add_argument('--proxy-server=http://YOUR_PROXY_IP:PORT') # Replace with 98IP proxy # Set WebDriver path and launch browser driver_path = '/path/to/chromedriver' driver = webdriver.Chrome(executable_path=driver_path, options=chrome_options) # Open target page and process data driver.get('https://example.com/protected-page') # ... further operations ... # Close browser driver.quit()
Note: Using plain-text proxy IPs is insecure; free proxies are often unreliable. Employ a proxy API service (like 98IP's) for better security and stability, retrieving and rotating IPs programmatically.
V. Advanced Techniques and Considerations
5.1 User-Agent Randomization:
Varying the User-Agent header adds crawler diversity, reducing detection.
from selenium.webdriver.chrome.service import Service from webdriver_manager.chrome import ChromeDriverManager from selenium.webdriver.chrome.options import Options import random user_agents = [ 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36', # ... more user agents ... ] chrome_options = Options() chrome_options.add_argument(f'user-agent={random.choice(user_agents)}') driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=chrome_options) # ... further operations ...
5.2 Error Handling and Retries:
Implement robust error handling and retry mechanisms to account for network issues and element loading failures.
VI. Conclusion
The combination of Selenium and proxy IPs provides a powerful approach to scraping dynamic web content while avoiding IP bans. Proper Selenium configuration, explicit waits, proxy integration, and advanced techniques are key to creating efficient and reliable web scrapers. Always adhere to website robots.txt
rules and relevant laws and regulations.
The above is the detailed content of Use Selenium and proxy IP to easily crawl dynamic page information. For more information, please follow other related articles on the PHP Chinese website!
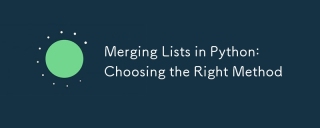
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
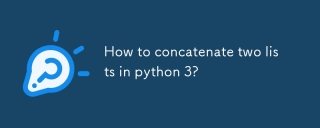
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
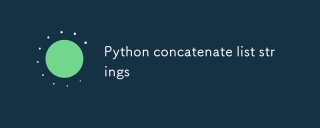
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
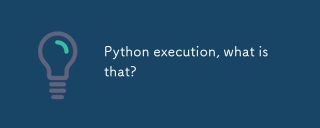
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
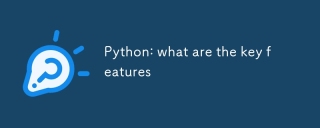
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
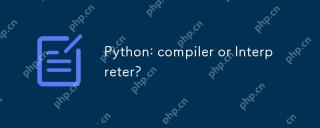
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
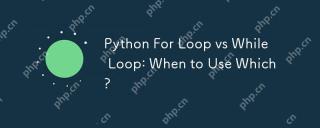
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
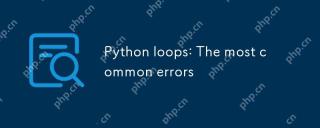
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
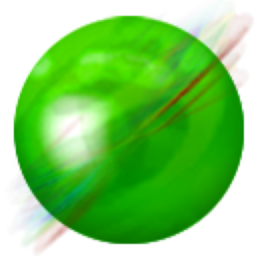
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
