C# list object value is overwritten: reference semantic issue
Why do all items in the list overwrite the previous values when adding a new value to the list? This puzzling behavior stems from the underlying data structures and reference semantics in C#.
Understand the semantics of classes and structures
In C#, classes are reference types, which means that variables of class type store a reference to the actual object location in memory. On the other hand, structures are value types, and variables directly hold the actual data.
In the code snippet provided:
List<Tag> tags = new List<Tag>(); Tag _tag = new Tag(); string[] tagList = new[] { "Foo", "Bar" }; foreach (string t in tagList) { _tag.tagName = t; // 设置所有属性 tags.Add(_tag); // 覆盖之前的数值 }
Since _tag
is a reference type, the tags.Add(_tag)
operation adds a reference to the same object to the list. As a result, all elements in the list actually point to the same instance. Modifying an instance via _tag
will change all items in the list.
Resolve the problem by creating a new instance
To prevent overwriting, a new Tag
instance should be created for each iteration of the loop:
foreach (string t in tagList) { Tag _tag = new Tag(); // 为每次迭代创建一个新实例 _tag.tagName = t; tags.Add(_tag); }
Now, each iteration creates a new Tag
instance, ensuring that each object in the list has its own unique value.
Additional Note: Structures and Classes
Using structs instead of classes eliminates overwriting issues because structs are value types. When a structure is assigned to a new variable, it creates a completely new instance containing its own set of values. Therefore, tags.Add(_tag)
performs a copy operation and does not affect previously added items.
In summary, understanding reference semantics and the difference between classes and structs is crucial to avoid overwriting issues in lists of objects in C#.
The above is the detailed content of Why are My List Items Overwriting Each Other in C#?. For more information, please follow other related articles on the PHP Chinese website!
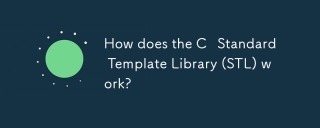
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
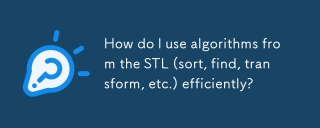
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
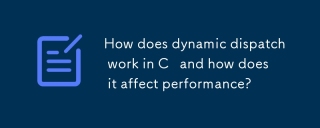
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
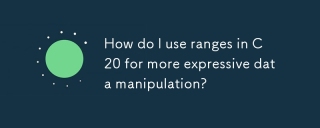
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
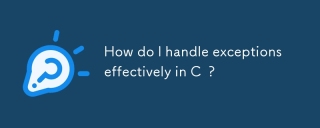
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
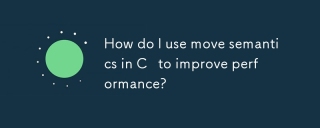
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
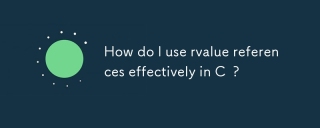
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
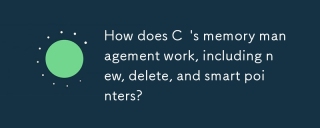
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
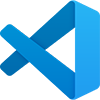
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
