


Use LINQ to determine unique elements in a list
We need to identify elements that only exist in one list and not in other lists. LINQ provides a neat solution to this challenge.
Consider the following code snippet:
class Program { static void Main(string[] args) { // 初始化两个Person对象列表。 List<Person> peopleList1 = new List<Person>(); peopleList1.Add(new Person() { ID = 1 }); peopleList1.Add(new Person() { ID = 2 }); peopleList1.Add(new Person() { ID = 3 }); List<Person> peopleList2 = new List<Person>(); peopleList2.Add(new Person() { ID = 1 }); peopleList2.Add(new Person() { ID = 2 }); peopleList2.Add(new Person() { ID = 3 }); peopleList2.Add(new Person() { ID = 4 }); peopleList2.Add(new Person() { ID = 5 }); } } class Person { public int ID { get; set; } }
Suppose we want to determine which people in peopleList2 do not exist in peopleList1. To do this, we can make use of LINQ's Where() method.
One way is to use the Any() method:
var result = peopleList2.Where(p => !peopleList1.Any(p2 => p2.ID == p.ID));
This query checks each person in peopleList2 to see if there is anyone in peopleList1 with the same ID. If there is no match, the person is included in the results.
Or, a more concise way of expressing the same logic is:
var result = peopleList2.Where(p => peopleList1.All(p2 => p2.ID != p.ID));
In this case, All() checks whether the ID of all people in peopleList1 is different from the ID of the current person in peopleList2. If this condition is true, the person is included in the results.
Note: It is important to note that both methods perform O(n*m) operations, which may cause performance issues on large datasets. Additional methods may be needed to optimize performance. The provided LINQ syntax provides a simple solution to the problem, but it is always recommended to evaluate it based on the performance requirements of the project.
The above is the detailed content of How Can LINQ Efficiently Identify Elements Unique to One List Among Multiple Lists?. For more information, please follow other related articles on the PHP Chinese website!
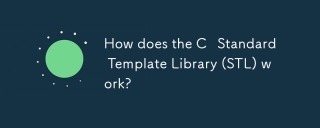
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
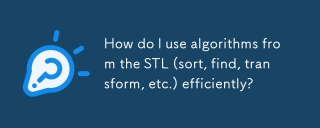
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
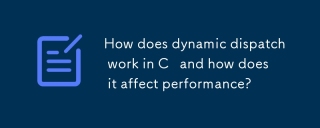
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
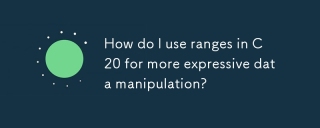
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
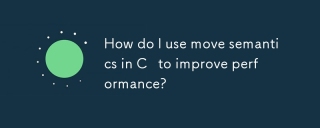
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
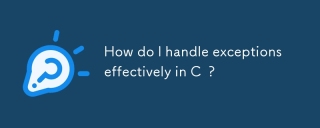
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
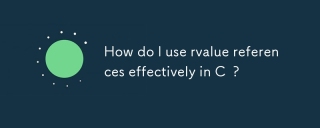
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
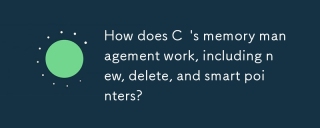
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
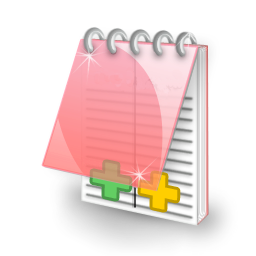
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
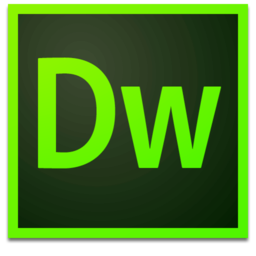
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
