


Json.NET processing large JSON streams in a memory-constrained environment
Parsing JSON files containing a large number of similar objects is a challenge in a memory-constrained environment. Consider the following scenario:
A huge JSON file contains many JSON objects with the same structure. If each object is a separate file, you can deserialize the objects one by one. However, due to the nested nature of the data, directly deserializing a JSON array of these objects will throw an exception saying an object was expected rather than a list.
Attempting to deserialize the entire JSON file into a list of C# objects successfully avoids the problem of reading the entire JSON file into RAM. However, it introduces a new problem of creating a C# list object that still holds all the data from the JSON file in memory.
To overcome these limitations, a strategy is needed to read objects one at a time. This approach eliminates the need to load the entire JSON string or all data into RAM as a C# object.
Solution
The following code example illustrates this approach:
JsonSerializer serializer = new JsonSerializer(); MyObject o; using (FileStream s = File.Open("bigfile.json", FileMode.Open)) using (StreamReader sr = new StreamReader(s)) using (JsonReader reader = new JsonTextReader(sr)) { while (reader.Read()) { // 只在流中存在"{"字符时才反序列化 if (reader.TokenType == JsonToken.StartObject) { o = serializer.Deserialize<MyObject>(reader); // 对o进行处理 } } }
This code simulates the initial method of deserializing the object in a loop, but it only deserializes the object when the reader encounters a "{" character in the stream. This approach efficiently processes JSON streams without overusing RAM by skipping to the next object until another starting object marker is found. Note that myobject
in the code has been corrected to MyObject
, and comments on the processing of the o
object have been added to facilitate users to add subsequent operations according to the actual situation.
The above is the detailed content of How Can I Parse Large JSON Streams Efficiently with Limited RAM in Json.NET?. For more information, please follow other related articles on the PHP Chinese website!
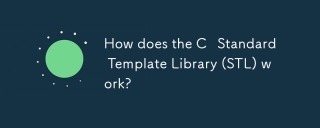
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
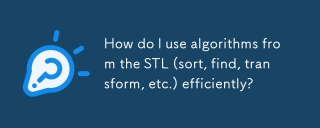
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
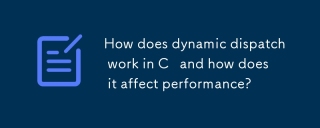
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
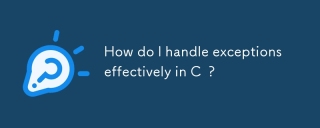
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
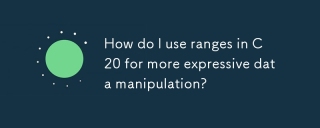
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
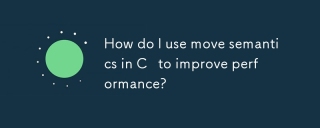
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
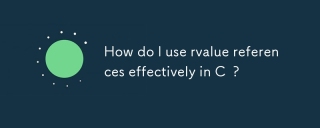
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
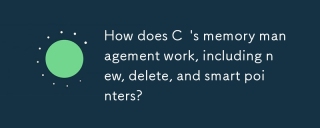
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
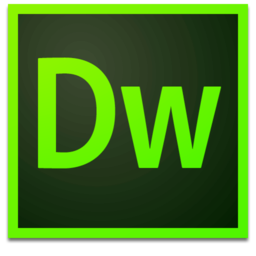
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
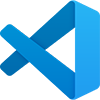
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
