Using C# to Execute SQL Script Files
This guide demonstrates how to execute an .SQL script file using C#. We'll utilize the Microsoft.SqlServer.Management.Smo
assembly for this task.
Implementation
The following C# code provides a complete implementation:
using Microsoft.SqlServer.Management.Smo; using Microsoft.SqlServer.Management.Common; using System.IO; using System.Data.SqlClient; public class SqlScriptExecutor { public static void Main(string[] args) { string connectionString = "Integrated Security=SSPI;Persist Security Info=False;Initial Catalog=ccwebgrity;Data Source=SURAJIT\SQLEXPRESS"; string scriptPath = @"E:\Project Docs\MX462-PD\MX756_ModMappings1.sql"; try { // Connect to the SQL Server database. using (SqlConnection connection = new SqlConnection(connectionString)) { connection.Open(); // Create a Server object. Server server = new Server(new ServerConnection(connection)); // Read the SQL script. string script = File.ReadAllText(scriptPath); // Execute the script. server.ConnectionContext.ExecuteNonQuery(script); Console.WriteLine("SQL script executed successfully."); } } catch (Exception ex) { Console.WriteLine($"Error executing SQL script: {ex.Message}"); } } }
Code Explanation
- Namespaces: The code imports necessary namespaces for SQL Server interaction and file I/O.
- Connection String: A connection string is defined to specify the SQL Server instance and database. Remember to replace this with your actual connection string.
- Script Path: The path to your .SQL script file is specified. Replace this with the correct path.
-
Connection and Server Object: A
SqlConnection
is created and opened, then aServer
object is instantiated to represent the database server. Theusing
statement ensures the connection is properly closed. -
Script Reading:
File.ReadAllText
reads the entire SQL script into a string. -
Script Execution:
server.ConnectionContext.ExecuteNonQuery
executes the SQL script. This method handles multiple SQL statements within the script file. -
Error Handling: A
try-catch
block handles potential exceptions during script execution.
This method provides a robust way to execute SQL scripts from within your C# application, managing multiple statements and line breaks effectively. Remember to adjust the connection string and script path to match your environment.
The above is the detailed content of How to Execute an .SQL Script File Using C#?. For more information, please follow other related articles on the PHP Chinese website!
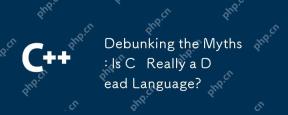
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
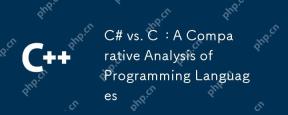
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
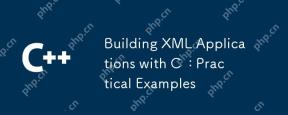
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
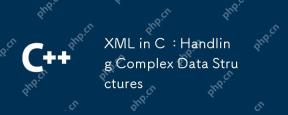
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
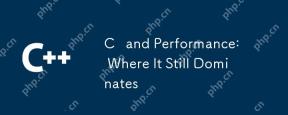
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
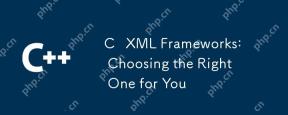
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
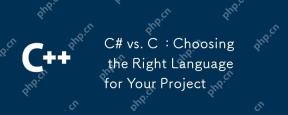
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
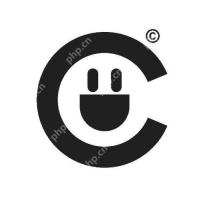
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
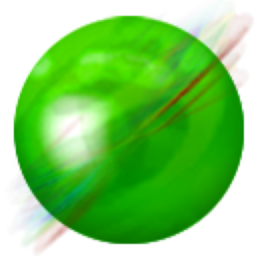
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
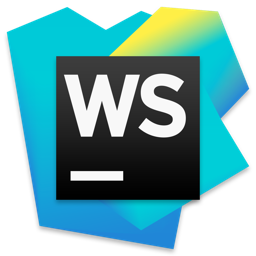
WebStorm Mac version
Useful JavaScript development tools
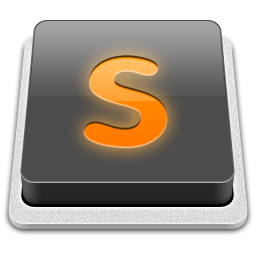
SublimeText3 Mac version
God-level code editing software (SublimeText3)
