This beginner's guide explores JavaScript's Document Object Model (DOM) manipulation, a powerful technique for creating interactive web pages. We'll cover the basics, enabling you to transform static content into dynamic, engaging experiences.
Understanding the DOM
The DOM is a programming interface representing your HTML document as a tree-like structure of objects. Think of it like a family tree: the tag is the root, branching out to
and
, which further branch into other elements like
<title></title>
, <div>, and <code><p></p>
. JavaScript lets you access, modify, add, remove, and rearrange these elements.
Why Learn DOM Manipulation?
- Dynamic Content: Update web page content (text, images) based on user actions.
- Interactive Features: Create elements like modals, dropdowns, and sliders.
- Event Handling: Respond to user input (clicks, key presses, mouse movements).
Accessing DOM Elements
Selecting elements is the first step. JavaScript offers several methods:
1. By ID
const element = document.getElementById('myId');
2. By Class Name
const elements = document.getElementsByClassName('myClass');
3. By Tag Name
const paragraphs = document.getElementsByTagName('p');
4. Using CSS Selectors (querySelector/querySelectorAll)
const firstElement = document.querySelector('.myClass'); // First element matching the selector const allElements = document.querySelectorAll('.myClass'); // All matching elements
Common DOM Manipulations
1. Changing Content
Modify element text or HTML:
- Text Content:
document.getElementById('example').textContent = 'New Text';
- HTML Content:
document.getElementById('example').innerHTML = '<b>Bold Text</b>';
2. Changing Attributes
Update or add element attributes:
const image = document.querySelector('img'); image.setAttribute('src', 'newImage.jpg'); image.setAttribute('alt', 'New Description');
3. Modifying Styles
Change element appearance using .style
or CSS classes:
const box = document.getElementById('box'); box.style.backgroundColor = 'blue'; box.style.color = 'white'; box.classList.add('active'); box.classList.remove('inactive'); box.classList.toggle('highlight');
4. Adding and Removing Elements
Create and manipulate elements dynamically:
- Create and Append:
const newDiv = document.createElement('div'); newDiv.textContent = 'I am a new div!'; document.body.appendChild(newDiv);
- Remove Element:
const element = document.getElementById('removeMe'); element.remove();
Event Handling
Combine DOM manipulation with event listeners to respond to user actions.
Example: Button Click
(Example code omitted for brevity, but would include adding an event listener to a button element.)
Common Events: click
, mouseover
, keydown
, etc.
DOM Traversal
Navigate the DOM tree using properties like .parentNode
, .parentElement
, .children
, .childNodes
, .nextElementSibling
, and .previousElementSibling
to access related elements.
Performance Tips
- Minimize DOM Access: Store element references in variables for reuse.
-
Batch Updates: Use
documentFragment
to add multiple elements efficiently. -
Avoid Excessive
innerHTML
: UsecreateElement
for better performance and security.
Conclusion
DOM manipulation is essential for modern web development. Mastering these techniques allows you to build dynamic and engaging user interfaces. Start with simple projects and gradually explore more advanced concepts.
The above is the detailed content of Understanding DOM Manipulation in JavaScript: A Beginner's Guide. For more information, please follow other related articles on the PHP Chinese website!
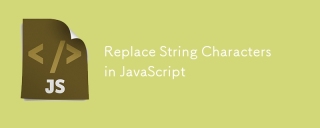
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
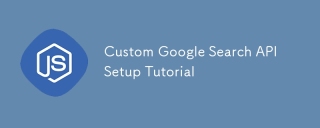
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
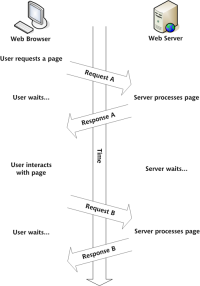
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
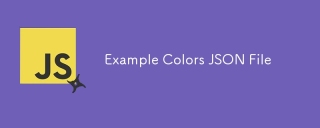
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
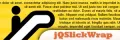
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
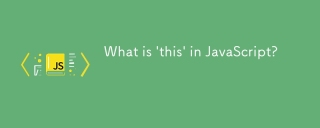
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
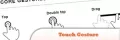
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
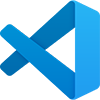
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
