Accessing the 64-bit Registry from a 32-bit Application
The Challenge: 32-bit applications often need access to 64-bit registry keys—for instance, to retrieve SQL Server instance details. However, they typically only access the Wow6432Node
branch.
The Solution: Windows 7 and later offer built-in support for accessing 64-bit registry keys via the .NET Framework 4.x or later. The key is using the RegistryView
enumeration:
-
RegistryView.Registry64
: Accesses the 64-bit registry. -
RegistryView.Registry32
: Accesses the 32-bit registry.
Code Example (Direct 64-bit Access):
This code snippet demonstrates direct access to the 64-bit registry:
using Microsoft.Win32; RegistryKey localKey = RegistryKey.OpenBaseKey(RegistryHive.LocalMachine, RegistryView.Registry64); RegistryKey sqlServerKey = localKey.OpenSubKey(@"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL"); string sqlExpressKeyName = (string)sqlServerKey.GetValue("SQLEXPRESS");
A More Robust Approach (Helper Functions):
For greater flexibility, these helper functions query both 32-bit and 64-bit registries, consolidating the results:
using Microsoft.Win32; using System.Collections.Generic; using System.Linq; public static IEnumerable<string> GetAllRegValueNames(string RegPath, RegistryHive hive = RegistryHive.LocalMachine) { var reg64 = GetRegValueNames(RegistryView.Registry64, RegPath, hive); var reg32 = GetRegValueNames(RegistryView.Registry32, RegPath, hive); var result = (reg64 != null && reg32 != null) ? reg64.Union(reg32) : (reg64 ?? reg32); return (result ?? new List<string>().AsEnumerable()).OrderBy(x => x); } public static object GetRegValue(string RegPath, string ValueName = "", RegistryHive hive = RegistryHive.LocalMachine) { return GetRegValue(RegistryView.Registry64, RegPath, ValueName, hive) ?? GetRegValue(RegistryView.Registry32, RegPath, ValueName, hive); } private static IEnumerable<string> GetRegValueNames(RegistryView view, string regPath, RegistryHive hive) { try { using (var key = RegistryKey.OpenBaseKey(hive, view).OpenSubKey(regPath)) { return key?.GetValueNames(); } } catch (Exception) { return null; } } private static object GetRegValue(RegistryView view, string regPath, string valueName, RegistryHive hive) { try { using (var key = RegistryKey.OpenBaseKey(hive, view).OpenSubKey(regPath)) { return key?.GetValue(valueName); } } catch (Exception) { return null; } }
Using the Helper Functions:
This example utilizes the helper functions:
var sqlRegPath = @"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL"; foreach (var valueName in GetAllRegValueNames(sqlRegPath)) { var value = GetRegValue(sqlRegPath, valueName); Console.WriteLine($"{valueName}={value}"); }
This improved approach handles potential exceptions and provides a more robust solution for accessing registry information regardless of the application's or registry key's architecture.
The above is the detailed content of How Can 32-Bit Applications Access the 64-Bit Windows Registry?. For more information, please follow other related articles on the PHP Chinese website!
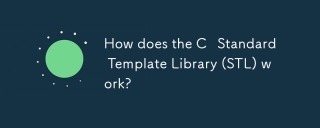
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
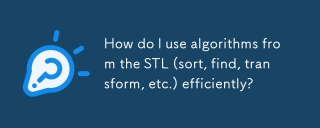
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
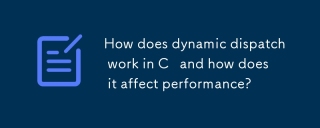
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
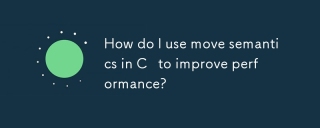
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
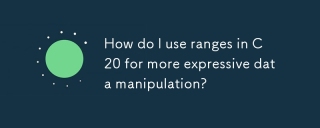
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
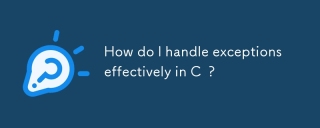
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
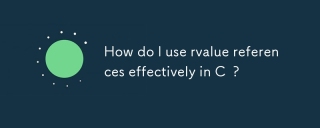
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
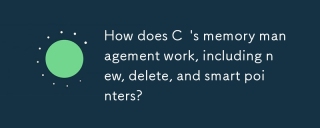
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
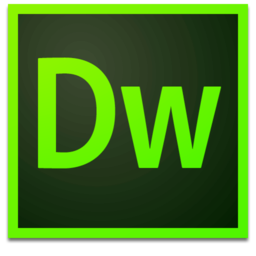
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
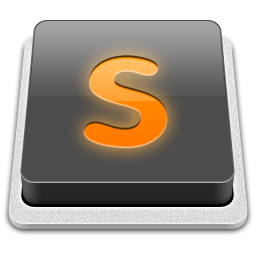
SublimeText3 Mac version
God-level code editing software (SublimeText3)
