Accessing Command-Line Arguments in WinForms Applications
Unlike console applications, WinForms apps don't directly expose command-line arguments via a main()
method's args
parameter. This article details how to retrieve these arguments within a WinForms application.
Using Environment.GetCommandLineArgs()
The Environment.GetCommandLineArgs()
method provides the solution. It returns a string array containing all command-line arguments passed to your application.
Here's a step-by-step guide:
-
Locate your application's entry point: This is typically found within the
Program.cs
file. -
Access the arguments within the
Main
method: Modify yourMain
method to utilizeEnvironment.GetCommandLineArgs()
:
static void Main(string[] args) { // Retrieve command-line arguments string[] commandLineArgs = Environment.GetCommandLineArgs(); // Process the arguments // ... }
-
Process the arguments: The
commandLineArgs
array contains your arguments.commandLineArgs[0]
is usually the application's path. Subsequent elements (commandLineArgs[1]
,commandLineArgs[2]
, etc.) represent the arguments you provided.
Example:
static void Main(string[] args) { string[] commandLineArgs = Environment.GetCommandLineArgs(); Console.WriteLine($"Application path: {commandLineArgs[0]}"); if (commandLineArgs.Length > 1) { Console.WriteLine("Command-line arguments:"); for (int i = 1; i < commandLineArgs.Length; i++) { Console.WriteLine($"- {commandLineArgs[i]}"); } } }
This approach offers a straightforward way to handle command-line arguments in your WinForms applications, enhancing the flexibility and functionality of your code.
The above is the detailed content of How Do I Pass Command-Line Arguments to My WinForms Application?. For more information, please follow other related articles on the PHP Chinese website!

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
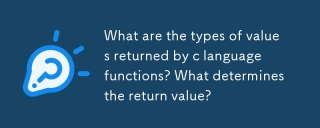
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing
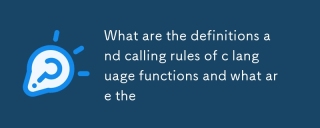
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
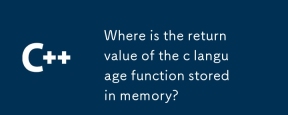
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
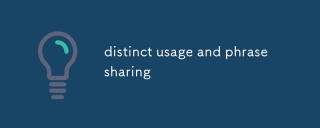
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
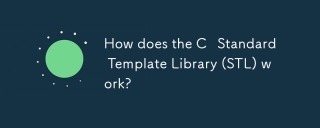
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
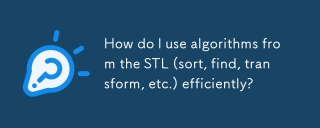
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
