


WinForms Progress Bar Updates from External Processes: A Non-Blocking Solution
WinForms progress bars offer visual feedback on task progress. However, managing progress updates from external calculations or libraries without freezing the UI presents a challenge. Directly updating the progress bar from within these external processes creates a tight coupling and can lead to UI freezes.
Effective Solution: Leveraging BackgroundWorker
The BackgroundWorker
class provides an elegant solution. It enables the execution of long-running operations on a separate thread, preventing UI blockage. Progress updates are seamlessly relayed back to the main UI thread.
Implementation Steps
-
BackgroundWorker Initialization:
Create a
BackgroundWorker
instance:BackgroundWorker backgroundWorker = new BackgroundWorker();
-
Event Handling:
Subscribe to the
BackgroundWorker
's events:backgroundWorker.DoWork += backgroundWorker_DoWork; backgroundWorker.ProgressChanged += backgroundWorker_ProgressChanged; backgroundWorker.RunWorkerCompleted += backgroundWorker_RunWorkerCompleted;
-
Initiating the Background Task:
Start the background operation using:
backgroundWorker.RunWorkerAsync(); ``` This is typically triggered by a button click or similar UI event.
-
Progress Reporting:
Inside the
backgroundWorker_DoWork
event handler, report progress at regular intervals:backgroundWorker.ReportProgress((j * 100) / totalIterations);
-
UI Progress Bar Update:
The
backgroundWorker_ProgressChanged
event handler updates the progress bar:progressBar1.Value = e.ProgressPercentage;
Illustrative Code
This example demonstrates the process:
private void Calculate(int i) { // Your external calculation logic here... double result = Math.Pow(i, i); // Example calculation } private void button1_Click(object sender, EventArgs e) { progressBar1.Maximum = 100; progressBar1.Value = 0; backgroundWorker.RunWorkerAsync(); } private void backgroundWorker_DoWork(object sender, DoWorkEventArgs e) { BackgroundWorker worker = sender as BackgroundWorker; int totalIterations = 100000; // Adjust as needed for (int j = 0; j < totalIterations; j++) { Calculate(j); worker.ReportProgress((j * 100) / totalIterations); } } private void backgroundWorker_ProgressChanged(object sender, ProgressChangedEventArgs e) { progressBar1.Value = e.ProgressPercentage; } private void backgroundWorker_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e) { // Handle completion, e.g., display a message MessageBox.Show("Calculation complete!"); }
This approach ensures smooth UI responsiveness while providing clear progress visualization, even with computationally intensive external calculations. Remember to handle potential exceptions within the backgroundWorker_DoWork
method for robustness.
The above is the detailed content of How Can I Display Progress from External Calculations in a WinForms Progress Bar Without Blocking the UI?. For more information, please follow other related articles on the PHP Chinese website!
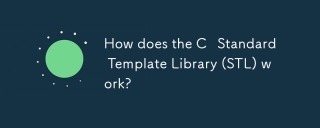
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
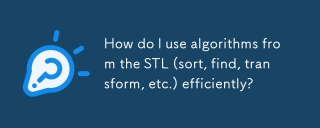
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
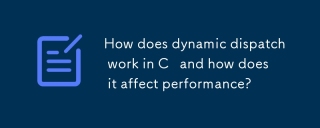
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
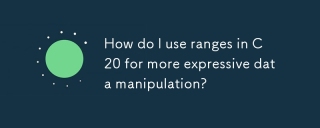
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
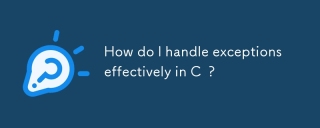
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
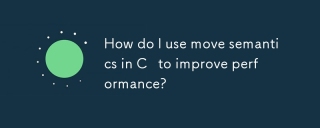
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
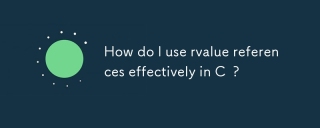
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
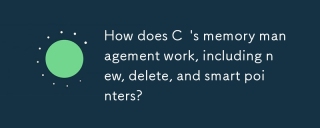
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
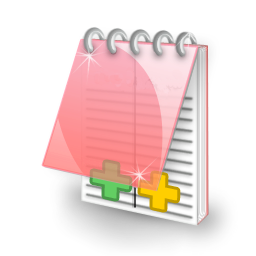
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
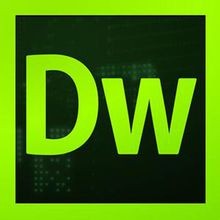
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
