


Pandas DataFrame Creation from Large SQL Queries: Memory Management Strategies
Processing massive SQL tables often leads to memory errors when creating Pandas DataFrames. This article explores effective methods for handling large datasets, preventing memory exhaustion while maintaining data integrity.
Leveraging Pandas' chunksize
Parameter
Pandas (version 0.15 and later) offers a robust solution: the chunksize
parameter within the read_sql
function. This allows for incremental data retrieval and processing, preventing memory overload.
Here's how to use it:
sql = "SELECT * FROM My_Table" for chunk in pd.read_sql_query(sql, engine, chunksize=5): # Process each chunk (e.g., append to a list, perform calculations, etc.) print(chunk)
This code fetches data in 5-row increments. Replace 5
with a suitable chunk size based on your system's memory capacity. Each chunk
is a DataFrame, enabling processing in manageable portions.
Alternative Approaches
While chunksize
is often sufficient, other techniques offer more control:
-
Database APIs: Direct interaction with database APIs (e.g., psycopg2 for PostgreSQL) provides granular control over data retrieval, allowing you to fetch specific data ranges using pagination techniques.
-
Generators: Generators yield data row by row, significantly reducing memory footprint. This is particularly useful for very large tables where even
chunksize
might prove insufficient. -
Low-Level Database Interactions: For ultimate control and optimization, leverage low-level database features to create custom data retrieval mechanisms tailored to your specific needs and database system.
The optimal approach depends on factors like project specifics, performance demands, and developer familiarity. A careful evaluation of each method's strengths and limitations is crucial for selecting the most efficient solution.
The above is the detailed content of How Can I Efficiently Handle Large SQL Queries to Avoid Memory Errors When Creating Pandas DataFrames?. For more information, please follow other related articles on the PHP Chinese website!
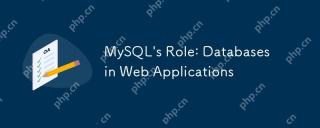
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
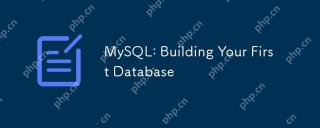
The steps to build a MySQL database include: 1. Create a database and table, 2. Insert data, and 3. Conduct queries. First, use the CREATEDATABASE and CREATETABLE statements to create the database and table, then use the INSERTINTO statement to insert the data, and finally use the SELECT statement to query the data.
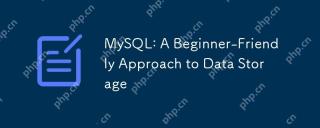
MySQL is suitable for beginners because it is easy to use and powerful. 1.MySQL is a relational database, and uses SQL for CRUD operations. 2. It is simple to install and requires the root user password to be configured. 3. Use INSERT, UPDATE, DELETE, and SELECT to perform data operations. 4. ORDERBY, WHERE and JOIN can be used for complex queries. 5. Debugging requires checking the syntax and use EXPLAIN to analyze the query. 6. Optimization suggestions include using indexes, choosing the right data type and good programming habits.
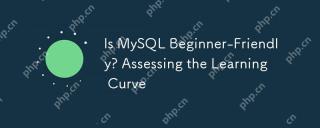
MySQL is suitable for beginners because: 1) easy to install and configure, 2) rich learning resources, 3) intuitive SQL syntax, 4) powerful tool support. Nevertheless, beginners need to overcome challenges such as database design, query optimization, security management, and data backup.
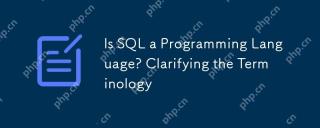
Yes,SQLisaprogramminglanguagespecializedfordatamanagement.1)It'sdeclarative,focusingonwhattoachieveratherthanhow.2)SQLisessentialforquerying,inserting,updating,anddeletingdatainrelationaldatabases.3)Whileuser-friendly,itrequiresoptimizationtoavoidper
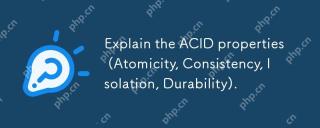
ACID attributes include atomicity, consistency, isolation and durability, and are the cornerstone of database design. 1. Atomicity ensures that the transaction is either completely successful or completely failed. 2. Consistency ensures that the database remains consistent before and after a transaction. 3. Isolation ensures that transactions do not interfere with each other. 4. Persistence ensures that data is permanently saved after transaction submission.
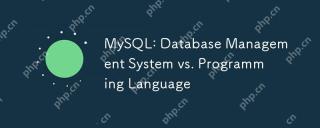
MySQL is not only a database management system (DBMS) but also closely related to programming languages. 1) As a DBMS, MySQL is used to store, organize and retrieve data, and optimizing indexes can improve query performance. 2) Combining SQL with programming languages, embedded in Python, using ORM tools such as SQLAlchemy can simplify operations. 3) Performance optimization includes indexing, querying, caching, library and table division and transaction management.
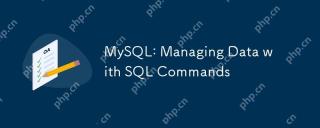
MySQL uses SQL commands to manage data. 1. Basic commands include SELECT, INSERT, UPDATE and DELETE. 2. Advanced usage involves JOIN, subquery and aggregate functions. 3. Common errors include syntax, logic and performance issues. 4. Optimization tips include using indexes, avoiding SELECT* and using LIMIT.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
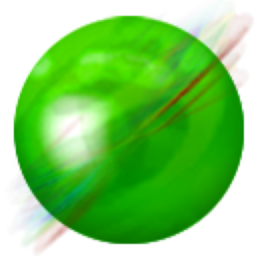
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
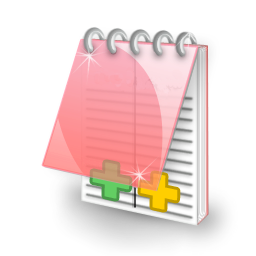
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
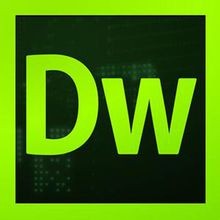
Dreamweaver CS6
Visual web development tools