The steps to build a MySQL database include: 1. Create a database and table, 2. Insert data, and 3. Conduct queries. First, use the CREATE DATABASE and CREATE TABLE statements to create the database and table, then use the INSERT INTO statement to insert the data, and finally use the SELECT statement to query the data.
introduction
In today's data-driven world, mastering database construction and management skills is a must-have tool for every developer. MySQL, as one of the most popular open source databases in the world, provides us with powerful capabilities and flexibility, making building databases both fun and efficient. The purpose of this article is to lead you to build your first MySQL database from scratch. By reading this article, you will learn how to design database structures, create tables, insert data, and perform basic query operations. Whether you are a beginner or a developer with some experience, this article will provide you with practical guidance and insights.
Review of basic knowledge
MySQL is a relational database management system (RDBMS) that uses a structured query language (SQL) to manage and manipulate data. Relational databases organize data through tables, each table containing rows and columns, similar to Excel tables. Understanding these basic concepts is essential to building a database.
Before you start building the database, you need to install MySQL server and a client tool such as MySQL Workbench or command line tools. The installation process varies by operating system, but can usually be easily done via the official website or package manager.
Core concept or function analysis
Definition and function of databases and tables
In MySQL, a database is a collection of data, and a table is the basic storage unit in the database. Each table contains multiple columns, each column defines the type and name of the data. Table design is the core part of database design, which determines how data is organized and accessed.
For example, suppose we want to build a book management system, we can create a table called books
to store book information:
CREATE TABLE books ( id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(100) NOT NULL, author VARCHAR(100) NOT NULL, isbn VARCHAR(13) UNIQUE, publication_date DATE );
This table defines the book's ID, title, author, ISBN, and publication date. AUTO_INCREMENT
ensures that each newly inserted book has a unique ID, and UNIQUE
ensures the uniqueness of the ISBN.
How it works
When you execute the CREATE TABLE
statement, MySQL creates a file on disk to store the table's data. The structure information of each table is stored in the system table, and MySQL uses this information to manage the access and operation of the table.
When inserting data, MySQL verifies the type and constraints of the data according to the definition of the table. If the data meets the requirements, MySQL will write the data to the table and update the relevant index to improve query efficiency.
Example of usage
Basic usage
Let's start by creating the database and tables:
CREATE DATABASE library; USE library; CREATE TABLE books ( id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(100) NOT NULL, author VARCHAR(100) NOT NULL, isbn VARCHAR(13) UNIQUE, publication_date DATE );
Now we can insert some data into the books
table:
INSERT INTO books (title, author, isbn, publication_date) VALUES ('The Great Gatsby', 'F. Scott Fitzgerald', '9780743273565', '1925-04-10'); INSERT INTO books (title, author, isbn, publication_date) VALUES ('To Kill a Mockingbird', 'Harper Lee', '9780446310789', '1960-07-11');
Query all books:
SELECT * FROM books;
Advanced Usage
Suppose we want to add a genres
table to store the book type and establish an association with books
table:
CREATE TABLE genres ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(50) NOT NULL ); CREATE TABLE book_genres ( book_id INT, genre_id INT, PRIMARY KEY (book_id, genre_id), FOREIGN KEY (book_id) REFERENCES books(id), FOREIGN KEY (genre_id) REFERENCES genres(id) ); INSERT INTO genres (name) VALUES ('Fiction'); INSERT INTO genres (name) VALUES ('Classic'); INSERT INTO book_genres (book_id, genre_id) VALUES (1, 1); INSERT INTO book_genres (book_id, genre_id) VALUES (1, 2); INSERT INTO book_genres (book_id, genre_id) VALUES (2, 1);
Now we can query the books and their types:
SELECT b.title, b.author, g.name AS genre FROM books b JOIN book_genres bg ON b.id = bg.book_id JOIN genres g ON bg.genre_id = g.id;
Common Errors and Debugging Tips
Common errors when building databases include data type mismatch, uniqueness violations, and foreign key reference errors. For example, if you try to insert an existing ISBN, MySQL will report an error:
INSERT INTO books (title, author, isbn, publication_date) VALUES ('The Great Gatsby', 'F. Scott Fitzgerald', '9780743273565', '1925-04-10'); -- Error: Duplicate entry '9780743273565' for key 'books.isbn'
The solution to this problem is to make sure the inserted data complies with the table's constraints, or to check the uniqueness of the data before inserting.
Performance optimization and best practices
In practical applications, optimizing database performance is crucial. Here are some optimization suggestions:
- Index : Creating indexes for frequently queried columns can significantly improve query speed. For example, create an index for
title
column ofbooks
table:
CREATE INDEX idx_title ON books(title);
Standardization : By standardizing database design, data redundancy can be reduced and data consistency can be improved. For example, we separate the book types from the
books
table and creategenres
andbook_genres
tables.Query optimization : Use
EXPLAIN
statement to analyze query plans and find out performance bottlenecks. For example:
EXPLAIN SELECT * FROM books WHERE title = 'The Great Gatsby';
- Best practice : Keep code readable and maintainable. For example, use meaningful table and column names to add comments to describe the purpose of the table and the meaning of the column.
Keeping these suggestions and best practices in mind when building your first MySQL database will help you create an efficient and reliable database system. Through continuous practice and learning, you will be able to deal with more complex database design and optimization challenges.
The above is the detailed content of MySQL: Building Your First Database. For more information, please follow other related articles on the PHP Chinese website!
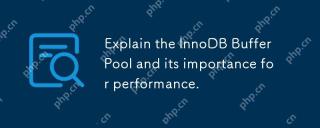
InnoDBBufferPool reduces disk I/O by caching data and indexing pages, improving database performance. Its working principle includes: 1. Data reading: Read data from BufferPool; 2. Data writing: After modifying the data, write to BufferPool and refresh it to disk regularly; 3. Cache management: Use the LRU algorithm to manage cache pages; 4. Reading mechanism: Load adjacent data pages in advance. By sizing the BufferPool and using multiple instances, database performance can be optimized.
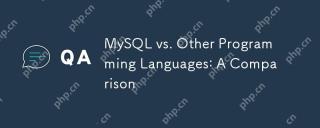
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
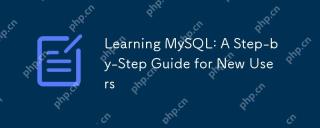
MySQL is worth learning because it is a powerful open source database management system suitable for data storage, management and analysis. 1) MySQL is a relational database that uses SQL to operate data and is suitable for structured data management. 2) The SQL language is the key to interacting with MySQL and supports CRUD operations. 3) The working principle of MySQL includes client/server architecture, storage engine and query optimizer. 4) Basic usage includes creating databases and tables, and advanced usage involves joining tables using JOIN. 5) Common errors include syntax errors and permission issues, and debugging skills include checking syntax and using EXPLAIN commands. 6) Performance optimization involves the use of indexes, optimization of SQL statements and regular maintenance of databases.
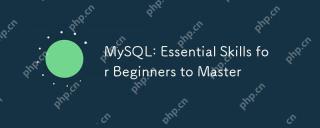
MySQL is suitable for beginners to learn database skills. 1. Install MySQL server and client tools. 2. Understand basic SQL queries, such as SELECT. 3. Master data operations: create tables, insert, update, and delete data. 4. Learn advanced skills: subquery and window functions. 5. Debugging and optimization: Check syntax, use indexes, avoid SELECT*, and use LIMIT.
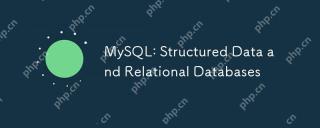
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
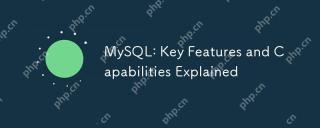
MySQL is an open source relational database management system that is widely used in Web development. Its key features include: 1. Supports multiple storage engines, such as InnoDB and MyISAM, suitable for different scenarios; 2. Provides master-slave replication functions to facilitate load balancing and data backup; 3. Improve query efficiency through query optimization and index use.
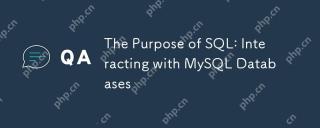
SQL is used to interact with MySQL database to realize data addition, deletion, modification, inspection and database design. 1) SQL performs data operations through SELECT, INSERT, UPDATE, DELETE statements; 2) Use CREATE, ALTER, DROP statements for database design and management; 3) Complex queries and data analysis are implemented through SQL to improve business decision-making efficiency.
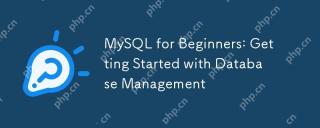
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
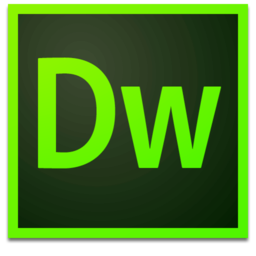
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
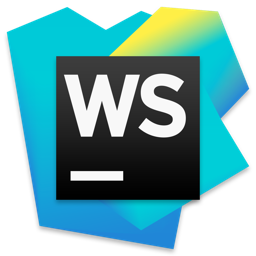
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.