One of the most confused keyword in JavaScript is the this keyword. It's a special identifier keyword that's automatically defined in the scope of every function, but what exactly it refers to confuse even seasoned JavaScript developers.
The this keyword refers to the context where a piece of code, such as a function's body, is supposed to run. Most typically, it is used in object methods, where this refers to the object that the method is attached to, thus allowing the same method to be reused on different objects.
The value of this can be identified where the function is executed not where the function is declared
We will examine different rules to identify the this in javascript
Default binding
The default rule we will apply most common case of function calls: standalone function execution. Think of this this rule as the default catch-all rule when none of the other rules apply.
In standalone functions call this value will be globalObject (in browser env it is window object, in node env it will be global)
function bar() { console.log(this) // this will be global object (window) } bar()
But......
the value of this can be different how the code is running in strict mode or non strict mode
If When function invoked as standalone function this typically refer to global object in non strict mode and undefined in strict mode
"use strict" function bar() { console.log(this) // undefined } bar()
Subtle but important, the global object is only eligible for the default binding if the contents of bar() are not running in strict mode;
function bar() { console.log(this) // global object (window) } (function() { "use strict" bar() })()
Implicit binding
When a regular function is invoked as a method of an object (obj.method()), this points to that object.
function bar() { console.log(this) } const obj = { name: "javascript", foo } obj.foo() // this here is object owing the function
Firstly, notice the manner in which bar() is declared and then later added as a reference property onto obj. Regardless of whether foo() is initially declared on obj, or is added as a reference later (as this snippet shows), in neither case is the function really "owned" or "contained" by the obj object.
Implicit lost:
When using callbacks, implicitly bound function loses that binding, which usually means it falls back to the default binding, of either the global object or undefined, depending on strict mode.
function bar() { setTimeout(function() { console.log(this) // this will be global object }, 1000); } const obj = { name: "javascript", bar } obj.bar() // this will be global object
function bar() { console.log(this) } const obj = { name: "javascript", bar } const a = obj.bar a() // this will be global object
Explicit binding
With implicit binding as we just saw, we had to mutate the object in question to include a reference on itself to the function, and use this property function reference to indirectly (implicitly) bind this to the object.
But, what if you want to force a function call to use a particular object for the this binding, without putting a property function reference on the object?
Yes its possible, javascript provide many methods like .map, .filter for array we have few methods in function. These are apply , call and bind
Here is the syntax for these methods
call
function bar() { console.log(this) // this will be global object (window) } bar()
apply
"use strict" function bar() { console.log(this) // undefined } bar()
Subtle different between apply and call. The syntax is same but we pass arguments as array in apply method
function bar() { console.log(this) // global object (window) } (function() { "use strict" bar() })()
Invoking bar with explicit binding by bar.call(..) allows us to force its this to be obj.
new Binding
When a function is used as a constructor (with the new keyword), its this is bound to the new object being constructed, no matter which object the constructor function is accessed on. The value of this becomes the value of the new expression unless the constructor returns another non–primitive value.
function bar() { console.log(this) } const obj = { name: "javascript", foo } obj.foo() // this here is object owing the function
The above is the detailed content of this keyword in javascript. For more information, please follow other related articles on the PHP Chinese website!
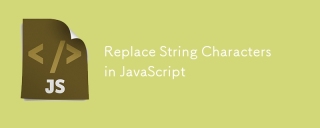
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
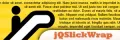
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
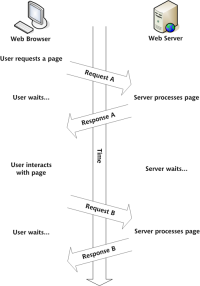
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
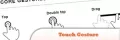
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
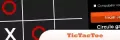
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
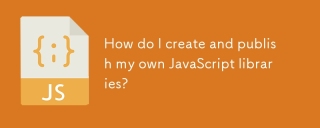
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
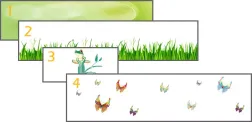
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
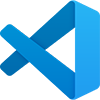
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
