


Copying Images with Transparency: A Windows Clipboard Solution
Problem: Preserving image transparency when copying to the Windows clipboard.
Background
The Windows clipboard doesn't inherently support transparency. However, it can handle various data formats, including PNG, which does support alpha channels (transparency).
Solution
To maintain transparency, employ this strategy:
Storing the Image
- Convert your image to a PNG stream using
MemoryStream
andImage.Save()
. - Also convert it to Device Independent Bitmap (DIB) format, widely accepted for its transparency handling.
- Store both the PNG and DIB streams, along with a standard bitmap, within a
DataObject
.
Retrieving the Image
- Access the
DataObject
from the clipboard. - Prioritize retrieving the image as a PNG; if unavailable, try DIB; then fall back to standard Bitmap or Image types.
- Crucially, create a clone of the retrieved image to avoid resource conflicts and potential crashes.
Code Implementation
public static void SetClipboardImage(Bitmap image, Bitmap imageNoTr, DataObject data) { Clipboard.Clear(); data ??= new DataObject(); //Null-conditional operator for brevity imageNoTr ??= image; //Null-conditional operator for brevity using (MemoryStream pngMemStream = new MemoryStream()) using (MemoryStream dibMemStream = new MemoryStream()) { data.SetData(DataFormats.Bitmap, true, imageNoTr); image.Save(pngMemStream, ImageFormat.Png); data.SetData("PNG", false, pngMemStream); byte[] dibData = ConvertToDib(image); dibMemStream.Write(dibData, 0, dibData.Length); data.SetData(DataFormats.Dib, false, dibMemStream); Clipboard.SetDataObject(data, true); } } public static Bitmap GetClipboardImage(DataObject retrievedData) { Bitmap clipboardimage = null; if (retrievedData.GetDataPresent("PNG", false)) { MemoryStream pngStream = retrievedData.GetData("PNG", false) as MemoryStream; using (Bitmap bm = new Bitmap(pngStream)) clipboardimage = ImageUtils.CloneImage(bm); } else if (retrievedData.GetDataPresent(DataFormats.Dib, false)) { MemoryStream dib = retrievedData.GetData(DataFormats.Dib, false) as MemoryStream; clipboardimage = dib != null ? ImageFromClipboardDib(dib.ToArray()) : null; } else if (retrievedData.GetDataPresent(DataFormats.Bitmap)) { clipboardimage = new Bitmap(retrievedData.GetData(DataFormats.Bitmap) as Image); } else if (retrievedData.GetDataPresent(typeof(Image))) { clipboardimage = new Bitmap(retrievedData.GetData(typeof(Image)) as Image); } return clipboardimage; }
The above is the detailed content of How to Maintain Transparency When Copying Images to the Windows Clipboard?. For more information, please follow other related articles on the PHP Chinese website!
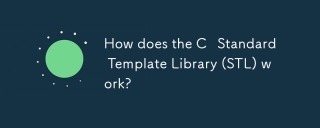
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
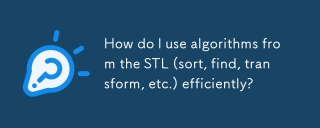
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
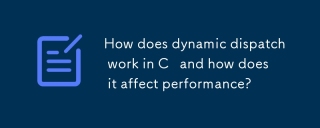
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
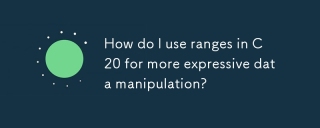
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
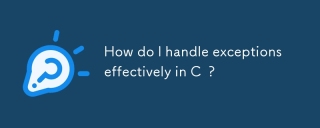
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
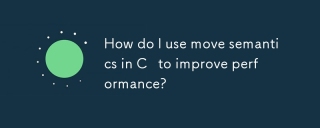
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
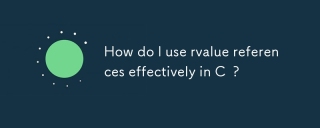
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
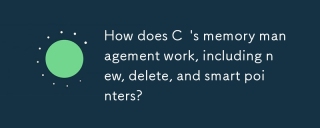
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
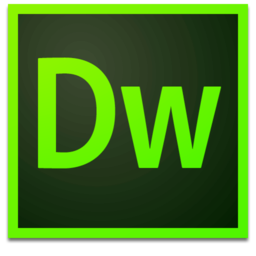
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
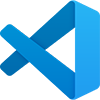
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
