Use C# reflection to obtain the attribute name string
When using reflection, it is often necessary to obtain the property name as a string. This becomes especially necessary when property names may change during refactoring, or when properties are accessed remotely through generic interfaces.
The traditional method is to manually specify the attribute name as the third parameter of methods such as ExposeProperty()
. However, this approach is error-prone and requires constant updating if the property name changes.
C# 6.0 solution: nameof expression
C# 6.0 provides a built-in solution: nameof
expressions. This expression allows you to directly retrieve the property name as a string at compile time.
string name = nameof(SomeClass.SomeProperty);
The above expression will return the string "SomeProperty" even if the property is later renamed.
Simplified reflection code
Using the nameof
expression, we can simplify the ExposeProperty()
method and eliminate the need to manually specify the property name:
public void ExposeProperty(string secretName, Type classType, string propertyName) { // 使用反射获取属性信息 PropertyInfo propertyInfo = classType.GetProperty(propertyName); // 使用反射公开属性 RemoteMgr.ExposeProperty(secretName, classType, propertyInfo); }
This method ensures that the property names used for remote access are always up to date, regardless of changes made during refactoring.
By leveraging nameof
expressions, developers can improve the maintainability and robustness of their code by dynamically retrieving property names as strings, eliminating potential bugs and ensuring that reflective operations remain accurate even after code modifications.
The above is the detailed content of How Can I Get a Property Name as a String in C# Reflection?. For more information, please follow other related articles on the PHP Chinese website!
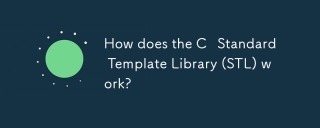
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
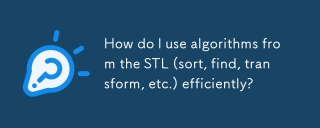
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
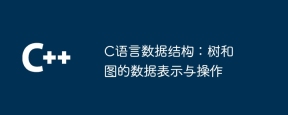
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
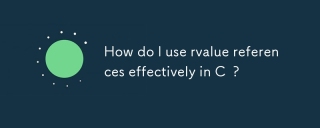
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
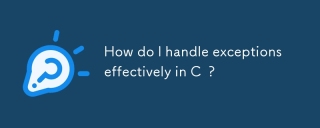
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
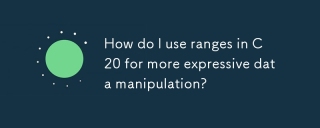
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
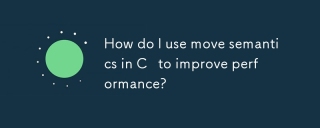
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
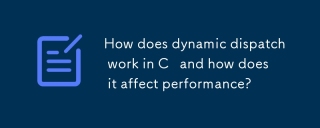
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
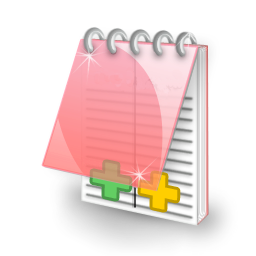
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
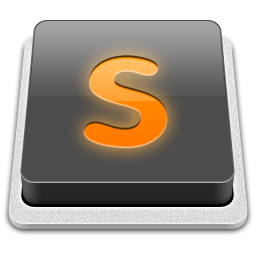
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.